mirror of
https://github.com/microsoft/PowerToys.git
synced 2024-12-24 09:07:59 +08:00
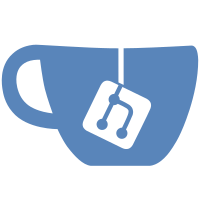
* [CropAndLock]Original POC code dump * Project rename and delete solution * Remove unused architectures * Update cppwinrt to be in line with the solution * Add to PowerToys solution and fix build errors * Initial module interface empty project * Module skeleton based on AlwaysOnTop * Add loggers to module interface * Add crop and lock to the runner * Enable starts and disable kills the process * Events reacting to hotkeys * Main application reacting to events * Initialize unhandled exception handling * Singleton in line with other projects * Also exit when PowerToys exit is detected * Create Settings page * React to shortcut changes in Settings * Disable Crop and Lock through an Event * Disable running Crop and Lock standalone * Remove Crop and Lock tray icon * Module Interface dll version * Fix main app resource file to include version * Make pch conditional on CI build * Add to signing * Remove settings screen opened by removed tray icon * Fix spellcheck * Yet another fix for spellcheck * Fix disabling utility * Fix solution build configurations * Fix C++ analyzer errors * Try to fix pre-compiled header CI errors * Fix crash while exiting with an active reparent window * Fix missing reference when building in release CI * Add OOBE page * GPO: Add admx and adml file changes * GPO: react to changes in GPO * Add quick access flyout menu entry * Use Crop And Lock icon * Use actual images for Settings and OOBE * Module and app telemetry * Add entry to README.md * Add to process lists * Additional logging * Attribution in Settings page * Add attribution to Community.md * Fix spellcheck * Fix typo in strings * Fix crash when window handle is no longer valid * Update COMMUNITY.md * Fix supportedOS in manifest * Don't show msgbox if detecting second instance * Remove unused hotkey * Tweak attribution * Fix attribution spellcheck
69 lines
6.6 KiB
C++
69 lines
6.6 KiB
C++
#include "ReportGPOValues.h"
|
|
#include <common/utils/gpo.h>
|
|
#include <fstream>
|
|
#include <unordered_map>
|
|
#include <string>
|
|
|
|
std::wstring gpo_rule_configured_to_string(powertoys_gpo::gpo_rule_configured_t gpo_rule)
|
|
{
|
|
switch (gpo_rule) {
|
|
case powertoys_gpo::gpo_rule_configured_wrong_value:
|
|
return L"wrong_value";
|
|
case powertoys_gpo::gpo_rule_configured_unavailable:
|
|
return L"can't_access";
|
|
case powertoys_gpo::gpo_rule_configured_not_configured:
|
|
return L"not_configured";
|
|
case powertoys_gpo::gpo_rule_configured_disabled:
|
|
return L"disabled";
|
|
case powertoys_gpo::gpo_rule_configured_enabled:
|
|
return L"enabled";
|
|
default:
|
|
return L"Unrecognized gpo_rule_configured_t value.";
|
|
}
|
|
}
|
|
|
|
void ReportGPOValues(const std::filesystem::path& tmpDir)
|
|
{
|
|
auto reportPath = tmpDir;
|
|
reportPath.append(L"gpo-configuration-info.txt");
|
|
std::wofstream report(reportPath);
|
|
report << "GPO policies configuration" << std::endl;
|
|
report << "getConfiguredAlwaysOnTopEnabledValue: " << gpo_rule_configured_to_string(powertoys_gpo::getConfiguredAlwaysOnTopEnabledValue()) << std::endl;
|
|
report << "getConfiguredAwakeEnabledValue: " << gpo_rule_configured_to_string(powertoys_gpo::getConfiguredAwakeEnabledValue()) << std::endl;
|
|
report << "getConfiguredColorPickerEnabledValue: " << gpo_rule_configured_to_string(powertoys_gpo::getConfiguredColorPickerEnabledValue()) << std::endl;
|
|
report << "getConfiguredCropAndLockEnabledValue: " << gpo_rule_configured_to_string(powertoys_gpo::getConfiguredCropAndLockEnabledValue()) << std::endl;
|
|
report << "getConfiguredFancyZonesEnabledValue: " << gpo_rule_configured_to_string(powertoys_gpo::getConfiguredFancyZonesEnabledValue()) << std::endl;
|
|
report << "getConfiguredFileLocksmithEnabledValue: " << gpo_rule_configured_to_string(powertoys_gpo::getConfiguredFileLocksmithEnabledValue()) << std::endl;
|
|
report << "getConfiguredSvgPreviewEnabledValue: " << gpo_rule_configured_to_string(powertoys_gpo::getConfiguredSvgPreviewEnabledValue()) << std::endl;
|
|
report << "getConfiguredMarkdownPreviewEnabledValue: " << gpo_rule_configured_to_string(powertoys_gpo::getConfiguredMarkdownPreviewEnabledValue()) << std::endl;
|
|
report << "getConfiguredMonacoPreviewEnabledValue: " << gpo_rule_configured_to_string(powertoys_gpo::getConfiguredMonacoPreviewEnabledValue()) << std::endl;
|
|
report << "getConfiguredPdfPreviewEnabledValue: " << gpo_rule_configured_to_string(powertoys_gpo::getConfiguredPdfPreviewEnabledValue()) << std::endl;
|
|
report << "getConfiguredGcodePreviewEnabledValue: " << gpo_rule_configured_to_string(powertoys_gpo::getConfiguredGcodePreviewEnabledValue()) << std::endl;
|
|
report << "getConfiguredSvgThumbnailsEnabledValue: " << gpo_rule_configured_to_string(powertoys_gpo::getConfiguredSvgThumbnailsEnabledValue()) << std::endl;
|
|
report << "getConfiguredPdfThumbnailsEnabledValue: " << gpo_rule_configured_to_string(powertoys_gpo::getConfiguredPdfThumbnailsEnabledValue()) << std::endl;
|
|
report << "getConfiguredGcodeThumbnailsEnabledValue: " << gpo_rule_configured_to_string(powertoys_gpo::getConfiguredGcodeThumbnailsEnabledValue()) << std::endl;
|
|
report << "getConfiguredStlThumbnailsEnabledValue: " << gpo_rule_configured_to_string(powertoys_gpo::getConfiguredStlThumbnailsEnabledValue()) << std::endl;
|
|
report << "getConfiguredHostsFileEditorEnabledValue: " << gpo_rule_configured_to_string(powertoys_gpo::getConfiguredHostsFileEditorEnabledValue()) << std::endl;
|
|
report << "getConfiguredImageResizerEnabledValue: " << gpo_rule_configured_to_string(powertoys_gpo::getConfiguredImageResizerEnabledValue()) << std::endl;
|
|
report << "getConfiguredKeyboardManagerEnabledValue: " << gpo_rule_configured_to_string(powertoys_gpo::getConfiguredKeyboardManagerEnabledValue()) << std::endl;
|
|
report << "getConfiguredFindMyMouseEnabledValue: " << gpo_rule_configured_to_string(powertoys_gpo::getConfiguredFindMyMouseEnabledValue()) << std::endl;
|
|
report << "getConfiguredMouseHighlighterEnabledValue: " << gpo_rule_configured_to_string(powertoys_gpo::getConfiguredMouseHighlighterEnabledValue()) << std::endl;
|
|
report << "getConfiguredMouseJumpEnabledValue: " << gpo_rule_configured_to_string(powertoys_gpo::getConfiguredMouseJumpEnabledValue()) << std::endl;
|
|
report << "getConfiguredMousePointerCrosshairsEnabledValue: " << gpo_rule_configured_to_string(powertoys_gpo::getConfiguredMousePointerCrosshairsEnabledValue()) << std::endl;
|
|
report << "getConfiguredMouseWithoutBordersEnabledValue: " << gpo_rule_configured_to_string(powertoys_gpo::getConfiguredMouseWithoutBordersEnabledValue()) << std::endl;
|
|
report << "getConfiguredPastePlainEnabledValue: " << gpo_rule_configured_to_string(powertoys_gpo::getConfiguredPastePlainEnabledValue()) << std::endl;
|
|
report << "getConfiguredPowerRenameEnabledValue: " << gpo_rule_configured_to_string(powertoys_gpo::getConfiguredPowerRenameEnabledValue()) << std::endl;
|
|
report << "getConfiguredPowerLauncherEnabledValue: " << gpo_rule_configured_to_string(powertoys_gpo::getConfiguredPowerLauncherEnabledValue()) << std::endl;
|
|
report << "getConfiguredQuickAccentEnabledValue: " << gpo_rule_configured_to_string(powertoys_gpo::getConfiguredQuickAccentEnabledValue()) << std::endl;
|
|
report << "getConfiguredScreenRulerEnabledValue: " << gpo_rule_configured_to_string(powertoys_gpo::getConfiguredScreenRulerEnabledValue()) << std::endl;
|
|
report << "getConfiguredShortcutGuideEnabledValue: " << gpo_rule_configured_to_string(powertoys_gpo::getConfiguredShortcutGuideEnabledValue()) << std::endl;
|
|
report << "getConfiguredTextExtractorEnabledValue: " << gpo_rule_configured_to_string(powertoys_gpo::getConfiguredTextExtractorEnabledValue()) << std::endl;
|
|
report << "getConfiguredPeekEnabledValue: " << gpo_rule_configured_to_string(powertoys_gpo::getConfiguredPeekEnabledValue()) << std::endl;
|
|
report << "getConfiguredVideoConferenceMuteEnabledValue: " << gpo_rule_configured_to_string(powertoys_gpo::getConfiguredVideoConferenceMuteEnabledValue()) << std::endl;
|
|
report << "getDisableAutomaticUpdateDownloadValue: " << gpo_rule_configured_to_string(powertoys_gpo::getDisableAutomaticUpdateDownloadValue()) << std::endl;
|
|
report << "getSuspendNewUpdateToastValue: " << gpo_rule_configured_to_string(powertoys_gpo::getSuspendNewUpdateToastValue()) << std::endl;
|
|
report << "getDisablePeriodicUpdateCheckValue: " << gpo_rule_configured_to_string(powertoys_gpo::getDisablePeriodicUpdateCheckValue()) << std::endl;
|
|
report << "getAllowExperimentationValue: " << gpo_rule_configured_to_string(powertoys_gpo::getAllowExperimentationValue()) << std::endl;
|
|
|
|
}
|