mirror of
https://github.com/microsoft/PowerToys.git
synced 2024-12-24 00:48:00 +08:00
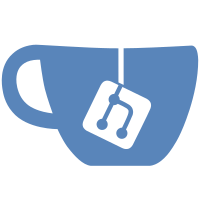
* Unify exe/dll naming - PowerToys.Runner Align naming with other exes - PowerToys Runner -> PowerToys.Runner * Unify exe/dll naming - Microsoft.PowerToys.Common.UI Project name - Microsoft.PowerToys.Common.UI -> Common.UI dll name - Microsoft.PowerToys.Common.UI.dll -> PowerToys.Common.UI.dll * Unify exe/dll naming - Settings Project names - Microsoft.PowerToys.Settings* -> Settings* Dll names - Microsoft.PowerToys.Settings*.dll -> PowerToys.Settings*.dll * Revert file autoformat * [Docs] Update paths to settings projects/files * Fix tests - Update path
73 lines
2.5 KiB
C#
73 lines
2.5 KiB
C#
// Copyright (c) Microsoft Corporation
|
|
// The Microsoft Corporation licenses this file to you under the MIT license.
|
|
// See the LICENSE file in the project root for more information.
|
|
|
|
using System.ComponentModel;
|
|
using Windows.UI.Accessibility;
|
|
using Windows.UI.Xaml;
|
|
using Windows.UI.Xaml.Automation;
|
|
using Windows.UI.Xaml.Controls;
|
|
using Windows.UI.Xaml.Media;
|
|
|
|
namespace Microsoft.PowerToys.Settings.UI.Controls
|
|
{
|
|
public class CheckBoxWithDescriptionControl : CheckBox
|
|
{
|
|
private CheckBoxWithDescriptionControl _checkBoxSubTextControl;
|
|
|
|
public CheckBoxWithDescriptionControl()
|
|
{
|
|
_checkBoxSubTextControl = (CheckBoxWithDescriptionControl)this;
|
|
this.Loaded += CheckBoxSubTextControl_Loaded;
|
|
}
|
|
|
|
protected override void OnApplyTemplate()
|
|
{
|
|
Update();
|
|
base.OnApplyTemplate();
|
|
}
|
|
|
|
private void Update()
|
|
{
|
|
if (!string.IsNullOrEmpty(Header))
|
|
{
|
|
AutomationProperties.SetName(this, Header);
|
|
}
|
|
}
|
|
|
|
private void CheckBoxSubTextControl_Loaded(object sender, RoutedEventArgs e)
|
|
{
|
|
StackPanel panel = new StackPanel() { Orientation = Orientation.Vertical };
|
|
panel.Children.Add(new TextBlock() { Margin = new Thickness(0, 10, 0, 0), Text = Header });
|
|
panel.Children.Add(new IsEnabledTextBlock() { FontSize = (double)App.Current.Resources["SecondaryTextFontSize"], Foreground = (SolidColorBrush)App.Current.Resources["TextFillColorSecondaryBrush"], Text = Description });
|
|
_checkBoxSubTextControl.Content = panel;
|
|
}
|
|
|
|
public static readonly DependencyProperty HeaderProperty = DependencyProperty.Register(
|
|
"Header",
|
|
typeof(string),
|
|
typeof(CheckBoxWithDescriptionControl),
|
|
new PropertyMetadata(default(string)));
|
|
|
|
public static readonly DependencyProperty DescriptionProperty = DependencyProperty.Register(
|
|
"Description",
|
|
typeof(object),
|
|
typeof(CheckBoxWithDescriptionControl),
|
|
new PropertyMetadata(default(string)));
|
|
|
|
[Localizable(true)]
|
|
public string Header
|
|
{
|
|
get => (string)GetValue(HeaderProperty);
|
|
set => SetValue(HeaderProperty, value);
|
|
}
|
|
|
|
[Localizable(true)]
|
|
public string Description
|
|
{
|
|
get => (string)GetValue(DescriptionProperty);
|
|
set => SetValue(DescriptionProperty, value);
|
|
}
|
|
}
|
|
}
|