mirror of
https://github.com/microsoft/PowerToys.git
synced 2024-12-27 03:14:18 +08:00
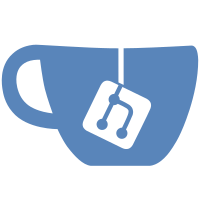
* Draft # Conflicts: # src/modules/keyboardmanager/KeyboardManagerEngineLibrary/KeyboardEventHandlers.cpp * Handle key case * Fix key count * Remember if win key was pressed * Don't search twice, remove redundant assignment * spelling
35 lines
1.0 KiB
C++
35 lines
1.0 KiB
C++
#pragma once
|
|
#include "Shortcut.h"
|
|
#include <variant>
|
|
|
|
// This class stores all the variables associated with each shortcut remapping
|
|
class RemapShortcut
|
|
{
|
|
public:
|
|
KeyShortcutUnion targetShortcut;
|
|
bool isShortcutInvoked;
|
|
ModifierKey winKeyInvoked;
|
|
// This bool value is only required for remapping shortcuts to Disable
|
|
bool isOriginalActionKeyPressed;
|
|
|
|
RemapShortcut(const KeyShortcutUnion& sc) :
|
|
targetShortcut(sc), isShortcutInvoked(false), winKeyInvoked(ModifierKey::Disabled), isOriginalActionKeyPressed(false)
|
|
{
|
|
}
|
|
|
|
RemapShortcut() :
|
|
targetShortcut(Shortcut()), isShortcutInvoked(false), winKeyInvoked(ModifierKey::Disabled), isOriginalActionKeyPressed(false)
|
|
{
|
|
}
|
|
|
|
inline bool operator==(const RemapShortcut& sc) const
|
|
{
|
|
return targetShortcut == sc.targetShortcut && isShortcutInvoked == sc.isShortcutInvoked && winKeyInvoked == sc.winKeyInvoked;
|
|
}
|
|
|
|
bool RemapToKey()
|
|
{
|
|
return targetShortcut.index() == 0;
|
|
}
|
|
};
|