mirror of
https://github.com/microsoft/PowerToys.git
synced 2024-12-27 11:21:27 +08:00
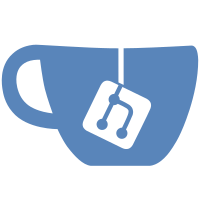
* archive * formmated code * reverted changes to test class file. * reverted changes to test file: reverted name * added class models and updated link * removed test console project
48 lines
1.2 KiB
C#
48 lines
1.2 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.Linq;
|
|
using System.Text;
|
|
using System.Threading.Tasks;
|
|
using System.Text.Json;
|
|
using System.Text.Json.Serialization;
|
|
|
|
namespace Microsoft.PowerToys.Settings.UI.ViewModels
|
|
{
|
|
public class GeneralSettings
|
|
{
|
|
public bool packaged { get; set; }
|
|
public bool startup { get; set; }
|
|
public bool is_elevated { get; set; }
|
|
public bool run_elevated { get; set; }
|
|
public bool is_admin { get; set; }
|
|
public string theme { get; set; }
|
|
public string system_theme { get; set; }
|
|
public string powertoys_version { get; set; }
|
|
|
|
public override string ToString()
|
|
{
|
|
return JsonSerializer.Serialize(this);
|
|
}
|
|
}
|
|
|
|
public class OutGoingGeneralSettings
|
|
{
|
|
public GeneralSettings general { get; set; }
|
|
|
|
public OutGoingGeneralSettings()
|
|
{
|
|
this.general = null;
|
|
}
|
|
|
|
public OutGoingGeneralSettings(GeneralSettings generalSettings)
|
|
{
|
|
this.general = generalSettings;
|
|
}
|
|
|
|
public override string ToString()
|
|
{
|
|
return JsonSerializer.Serialize(this);
|
|
}
|
|
}
|
|
}
|