mirror of
https://github.com/microsoft/PowerToys.git
synced 2024-12-24 17:18:00 +08:00
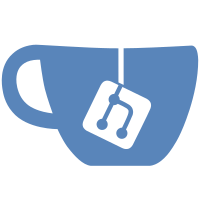
* Analyzers CPP Changing the warning level from 3 to 4. change some project files to make them use the warning config in cpp props file. * Analyzers C++ turn on warning 4706 Change Cpp.Build.props file to enable 4706 fix BugReportTool code to get rid of 4706 * Turn on warning 4100 and fix the code * Follow c++ core guidelines * Adapting to PR comments
46 lines
1.1 KiB
C++
46 lines
1.1 KiB
C++
#pragma once
|
|
#include <Windows.h>
|
|
#include <functional>
|
|
|
|
namespace CentralizedHotkeys
|
|
{
|
|
struct Action
|
|
{
|
|
std::wstring moduleName;
|
|
std::function<void(WORD, WORD)> action;
|
|
|
|
Action(std::wstring moduleName = L"", std::function<void(WORD, WORD)> action = ([](WORD /*modifiersMask*/, WORD /*vkCode*/) {}))
|
|
{
|
|
this->moduleName = moduleName;
|
|
this->action = action;
|
|
}
|
|
};
|
|
|
|
struct Shortcut
|
|
{
|
|
WORD modifiersMask;
|
|
WORD vkCode;
|
|
|
|
Shortcut(WORD modifiersMask = 0, WORD vkCode = 0)
|
|
{
|
|
this->modifiersMask = modifiersMask;
|
|
this->vkCode = vkCode;
|
|
}
|
|
|
|
bool operator<(const Shortcut& key) const
|
|
{
|
|
return std::pair<WORD, WORD>{ this->modifiersMask, this->vkCode } < std::pair<WORD, WORD>{ key.modifiersMask, key.vkCode };
|
|
}
|
|
};
|
|
|
|
std::wstring ToWstring(const Shortcut& shortcut);
|
|
|
|
bool AddHotkeyAction(Shortcut shortcut, Action action);
|
|
|
|
void UnregisterHotkeysForModule(std::wstring moduleName);
|
|
|
|
void PopulateHotkey(Shortcut shortcut);
|
|
|
|
void RegisterWindow(HWND hwnd);
|
|
}
|