mirror of
https://github.com/microsoft/PowerToys.git
synced 2024-12-26 02:28:17 +08:00
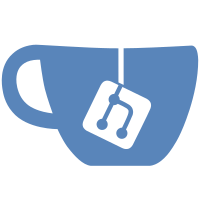
* [Launcher/Settings] Low Level Keyboard Hooks * [Run] LowLevel Keyboard Hook for Hotkeys * Prevent shortcuts from auto repeating when keeping the keys pressed down
104 lines
2.6 KiB
C#
104 lines
2.6 KiB
C#
// Copyright (c) Microsoft Corporation
|
|
// The Microsoft Corporation licenses this file to you under the MIT license.
|
|
// See the LICENSE file in the project root for more information.
|
|
|
|
using System.Text;
|
|
using System.Text.Json.Serialization;
|
|
using Microsoft.PowerToys.Settings.UI.Lib.Utilities;
|
|
|
|
namespace Microsoft.PowerToys.Settings.UI.Lib
|
|
{
|
|
public class HotkeySettings
|
|
{
|
|
public HotkeySettings()
|
|
{
|
|
this.Win = false;
|
|
this.Ctrl = false;
|
|
this.Alt = false;
|
|
this.Shift = false;
|
|
this.Key = string.Empty;
|
|
this.Code = 0;
|
|
}
|
|
|
|
public HotkeySettings(bool win, bool ctrl, bool alt, bool shift, string key, int code)
|
|
{
|
|
Win = win;
|
|
Ctrl = ctrl;
|
|
Alt = alt;
|
|
Shift = shift;
|
|
Key = key;
|
|
Code = code;
|
|
}
|
|
|
|
public HotkeySettings Clone()
|
|
{
|
|
return new HotkeySettings(Win, Ctrl, Alt, Shift, Key, Code);
|
|
}
|
|
|
|
[JsonPropertyName("win")]
|
|
public bool Win { get; set; }
|
|
|
|
[JsonPropertyName("ctrl")]
|
|
public bool Ctrl { get; set; }
|
|
|
|
[JsonPropertyName("alt")]
|
|
public bool Alt { get; set; }
|
|
|
|
[JsonPropertyName("shift")]
|
|
public bool Shift { get; set; }
|
|
|
|
[JsonPropertyName("key")]
|
|
public string Key { get; set; }
|
|
|
|
[JsonPropertyName("code")]
|
|
public int Code { get; set; }
|
|
|
|
public override string ToString()
|
|
{
|
|
StringBuilder output = new StringBuilder();
|
|
|
|
if (Win)
|
|
{
|
|
output.Append("Win + ");
|
|
}
|
|
|
|
if (Ctrl)
|
|
{
|
|
output.Append("Ctrl + ");
|
|
}
|
|
|
|
if (Alt)
|
|
{
|
|
output.Append("Alt + ");
|
|
}
|
|
|
|
if (Shift)
|
|
{
|
|
output.Append("Shift + ");
|
|
}
|
|
|
|
if (Code > 0)
|
|
{
|
|
var localKey = Helper.GetKeyName((uint)Code);
|
|
output.Append(localKey);
|
|
}
|
|
else if (output.Length >= 2)
|
|
{
|
|
output.Remove(output.Length - 2, 2);
|
|
}
|
|
|
|
return output.ToString();
|
|
}
|
|
|
|
public bool IsValid()
|
|
{
|
|
return (Alt || Ctrl || Win || Shift) && Code != 0;
|
|
}
|
|
|
|
public bool IsEmpty()
|
|
{
|
|
return !Alt && !Ctrl && !Win && !Shift && Code == 0;
|
|
}
|
|
}
|
|
}
|