mirror of
https://github.com/microsoft/PowerToys.git
synced 2024-12-25 01:27:59 +08:00
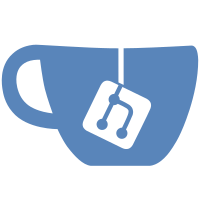
* Returning individual queries for each plugin * Changed cancellation token from Query type to directly using the rawQuery * Changed the way we get the plugins for which we execute the query * updated UpdateResultView to take a string instead of query * Changed the way we set a query for each plugin * removed todo comment * global plugins are added as a part of the query builder * Fix for plugin.json of Folder plugin being copied into the shell plugin * >,< and : are not allowed in file paths and indexer creates a query which searches compares if a file name is greater than or lesser than the query * Reformatted the regex * catching the exception * fixed existing tests * modified it so that it works with action keyword as well as action keywords * Added unit tests for non global plugins * fixed test * add back whitespace that was removed by mistake * fix regex * modified the cold start query * remove extra condition * terms being modified as expected * used key value pairs to iterate through the dictionary * renamed variable * added check for an empty dictionary * remove : because it may appear in the file path * fix some whitespace warnings that were being treated as errors
202 lines
8.4 KiB
C#
202 lines
8.4 KiB
C#
// Copyright (c) Microsoft Corporation
|
|
// The Microsoft Corporation licenses this file to you under the MIT license.
|
|
// See the LICENSE file in the project root for more information.
|
|
|
|
using System.Collections.Generic;
|
|
using NUnit.Framework;
|
|
using Wox.Core.Plugin;
|
|
using Wox.Plugin;
|
|
|
|
namespace Wox.Test
|
|
{
|
|
public class QueryBuilderTest
|
|
{
|
|
private bool AreEqual(Query firstQuery, Query secondQuery)
|
|
{
|
|
return firstQuery.ActionKeyword.Equals(secondQuery.ActionKeyword)
|
|
&& firstQuery.Search.Equals(secondQuery.Search)
|
|
&& firstQuery.RawQuery.Equals(secondQuery.RawQuery);
|
|
}
|
|
|
|
[Test]
|
|
public void QueryBuilder_ShouldRemoveExtraSpaces_ForNonGlobalPlugin()
|
|
{
|
|
// Arrange
|
|
var nonGlobalPlugins = new Dictionary<string, PluginPair>
|
|
{
|
|
{ ">", new PluginPair { Metadata = new PluginMetadata { ActionKeywords = new List<string> { ">" } } } },
|
|
};
|
|
string searchQuery = "> file.txt file2 file3";
|
|
|
|
// Act
|
|
var pluginQueryPairs = QueryBuilder.Build(ref searchQuery, nonGlobalPlugins);
|
|
|
|
// Assert
|
|
Assert.AreEqual("> file.txt file2 file3", searchQuery);
|
|
}
|
|
|
|
[Test]
|
|
public void QueryBuilder_ShouldRemoveExtraSpaces_ForDisabledNonGlobalPlugin()
|
|
{
|
|
// Arrange
|
|
var nonGlobalPlugins = new Dictionary<string, PluginPair>
|
|
{
|
|
{ ">", new PluginPair { Metadata = new PluginMetadata { ActionKeywords = new List<string> { ">" }, Disabled = true } } },
|
|
};
|
|
string searchQuery = "> file.txt file2 file3";
|
|
|
|
// Act
|
|
var pluginQueryPairs = QueryBuilder.Build(ref searchQuery, nonGlobalPlugins);
|
|
|
|
// Assert
|
|
Assert.AreEqual("> file.txt file2 file3", searchQuery);
|
|
}
|
|
|
|
[Test]
|
|
public void QueryBuilder_ShouldRemoveExtraSpaces_ForGlobalPlugin()
|
|
{
|
|
// Arrange
|
|
string searchQuery = "file.txt file2 file3";
|
|
|
|
// Act
|
|
var pluginQueryPairs = QueryBuilder.Build(ref searchQuery, new Dictionary<string, PluginPair>());
|
|
|
|
// Assert
|
|
Assert.AreEqual("file.txt file2 file3", searchQuery);
|
|
}
|
|
|
|
[Test]
|
|
public void QueryBuilder_ShouldGenerateSameQuery_IfEitherActionKeywordOrActionKeywordsListIsSet()
|
|
{
|
|
// Arrange
|
|
string searchQuery = "> query";
|
|
var firstPlugin = new PluginPair { Metadata = new PluginMetadata { ActionKeywords = new List<string> { ">" } } };
|
|
var secondPlugin = new PluginPair { Metadata = new PluginMetadata { ActionKeyword = ">" } };
|
|
|
|
var nonGlobalPluginWithActionKeywords = new Dictionary<string, PluginPair>
|
|
{
|
|
{ ">", firstPlugin },
|
|
};
|
|
|
|
var nonGlobalPluginWithActionKeyword = new Dictionary<string, PluginPair>
|
|
{
|
|
{ ">", secondPlugin },
|
|
};
|
|
string[] terms = { ">", "query" };
|
|
Query expectedQuery = new Query("> query", "query", terms, ">");
|
|
|
|
// Act
|
|
var queriesForPluginsWithActionKeywords = QueryBuilder.Build(ref searchQuery, nonGlobalPluginWithActionKeywords);
|
|
var queriesForPluginsWithActionKeyword = QueryBuilder.Build(ref searchQuery, nonGlobalPluginWithActionKeyword);
|
|
|
|
var firstQuery = queriesForPluginsWithActionKeyword.GetValueOrDefault(firstPlugin);
|
|
var secondQuery = queriesForPluginsWithActionKeywords.GetValueOrDefault(secondPlugin);
|
|
|
|
// Assert
|
|
Assert.IsTrue(AreEqual(firstQuery, expectedQuery));
|
|
Assert.IsTrue(AreEqual(firstQuery, secondQuery));
|
|
}
|
|
|
|
[Test]
|
|
public void QueryBuilder_ShouldGenerateCorrectQueries_ForPluginsWithMultipleActionKeywords()
|
|
{
|
|
// Arrange
|
|
var plugin = new PluginPair { Metadata = new PluginMetadata { ActionKeywords = new List<string> { "a", "b" } } };
|
|
var nonGlobalPlugins = new Dictionary<string, PluginPair>
|
|
{
|
|
{ "a", plugin },
|
|
{ "b", plugin },
|
|
};
|
|
|
|
var firstQueryText = "asearch";
|
|
var secondQueryText = "bsearch";
|
|
|
|
// Act
|
|
var firstPluginQueryPair = QueryBuilder.Build(ref firstQueryText, nonGlobalPlugins);
|
|
var firstQuery = firstPluginQueryPair.GetValueOrDefault(plugin);
|
|
|
|
var secondPluginQueryPairs = QueryBuilder.Build(ref secondQueryText, nonGlobalPlugins);
|
|
var secondQuery = secondPluginQueryPairs.GetValueOrDefault(plugin);
|
|
|
|
// Assert
|
|
Assert.IsTrue(AreEqual(firstQuery, new Query { ActionKeyword = "a", RawQuery = "asearch", Search = "search" }));
|
|
Assert.IsTrue(AreEqual(secondQuery, new Query { ActionKeyword = "b", RawQuery = "bsearch", Search = "search" }));
|
|
}
|
|
|
|
[Test]
|
|
public void QueryBuild_ShouldGenerateSameSearchQuery_WithOrWithoutSpaceAfterActionKeyword()
|
|
{
|
|
// Arrange
|
|
var plugin = new PluginPair { Metadata = new PluginMetadata { ActionKeywords = new List<string> { "a" } } };
|
|
var nonGlobalPlugins = new Dictionary<string, PluginPair>
|
|
{
|
|
{ "a", plugin },
|
|
};
|
|
|
|
var firstQueryText = "asearch";
|
|
var secondQueryText = "a search";
|
|
|
|
// Act
|
|
var firstPluginQueryPair = QueryBuilder.Build(ref firstQueryText, nonGlobalPlugins);
|
|
var firstQuery = firstPluginQueryPair.GetValueOrDefault(plugin);
|
|
|
|
var secondPluginQueryPairs = QueryBuilder.Build(ref secondQueryText, nonGlobalPlugins);
|
|
var secondQuery = secondPluginQueryPairs.GetValueOrDefault(plugin);
|
|
|
|
// Assert
|
|
Assert.IsTrue(firstQuery.Search.Equals(secondQuery.Search));
|
|
Assert.IsTrue(firstQuery.ActionKeyword.Equals(secondQuery.ActionKeyword));
|
|
}
|
|
|
|
[Test]
|
|
public void QueryBuild_ShouldGenerateCorrectQuery_ForPluginsWhoseActionKeywordsHaveSamePrefix()
|
|
{
|
|
// Arrange
|
|
string searchQuery = "abcdefgh";
|
|
var firstPlugin = new PluginPair { Metadata = new PluginMetadata { ActionKeyword = "ab", ID = "plugin1" } };
|
|
var secondPlugin = new PluginPair { Metadata = new PluginMetadata { ActionKeyword = "abcd", ID = "plugin2" } };
|
|
|
|
var nonGlobalPlugins = new Dictionary<string, PluginPair>
|
|
{
|
|
{ "ab", firstPlugin },
|
|
{ "abcd", secondPlugin },
|
|
};
|
|
|
|
// Act
|
|
var pluginQueryPairs = QueryBuilder.Build(ref searchQuery, nonGlobalPlugins);
|
|
|
|
var firstQuery = pluginQueryPairs.GetValueOrDefault(firstPlugin);
|
|
var secondQuery = pluginQueryPairs.GetValueOrDefault(secondPlugin);
|
|
|
|
// Assert
|
|
Assert.IsTrue(AreEqual(firstQuery, new Query { RawQuery = searchQuery, Search = searchQuery.Substring(firstPlugin.Metadata.ActionKeyword.Length), ActionKeyword = firstPlugin.Metadata.ActionKeyword } ));
|
|
Assert.IsTrue(AreEqual(secondQuery, new Query { RawQuery = searchQuery, Search = searchQuery.Substring(secondPlugin.Metadata.ActionKeyword.Length), ActionKeyword = secondPlugin.Metadata.ActionKeyword }));
|
|
}
|
|
|
|
[Test]
|
|
public void QueryBuilder_ShouldSetTermsCorrently_WhenCalled()
|
|
{
|
|
// Arrange
|
|
string searchQuery = "abcd efgh";
|
|
var firstPlugin = new PluginPair { Metadata = new PluginMetadata { ActionKeyword = "ab", ID = "plugin1" } };
|
|
var secondPlugin = new PluginPair { Metadata = new PluginMetadata { ActionKeyword = "abcd", ID = "plugin2" } };
|
|
|
|
var nonGlobalPlugins = new Dictionary<string, PluginPair>
|
|
{
|
|
{ "ab", firstPlugin },
|
|
{ "abcd", secondPlugin },
|
|
};
|
|
|
|
// Act
|
|
var pluginQueryPairs = QueryBuilder.Build(ref searchQuery, nonGlobalPlugins);
|
|
|
|
var firstQuery = pluginQueryPairs.GetValueOrDefault(firstPlugin);
|
|
var secondQuery = pluginQueryPairs.GetValueOrDefault(secondPlugin);
|
|
|
|
// Assert
|
|
Assert.IsTrue(firstQuery.Terms[0].Equals("cd") && firstQuery.Terms[1].Equals("efgh") && firstQuery.Terms.Length == 2);
|
|
Assert.IsTrue(secondQuery.Terms[0].Equals("efgh") && secondQuery.Terms.Length == 1);
|
|
}
|
|
}
|
|
}
|