mirror of
https://github.com/microsoft/PowerToys.git
synced 2025-01-07 03:47:56 +08:00
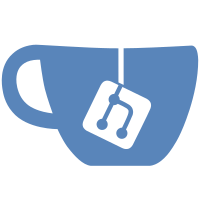
* Moved Logger/Log.cs from Wox.Infrastructure to Wox.Plugin - Installed Logger dependency in Wox.Plugin: NLog.Extensions.Logging - Moved file Log.cs from Wox.Infrastructure/Logger/ to Wox.Plugin/Logger - Moved file Constant.cs from Wox.Infrastructure to Wox.Plugin: This file was moved since Log.cs depends on this class - Copied Wox.Infrastructure.Helper.NonNull to Wox.Plugin.Constant since Constant.cs depends on this method - Replaced all "using Wox.Infrastructure.Logger" to "using Wox.Plugin.Logger" in all files as needed - Replaced Wox.Infrastructure.Constant to Wox.Plugin.Constant in all files as needed * Removed Nlog.Extensions.Logging from Wox.Infrastructure * Added logging and suppressed general exceptions (CA1031: Do not catch general exception types) * Resolved fxcop errors introduced by newly added Log.cs - CA1307: Specify StringComparison for clarity - CA2000: Dispose objects before losing scope - CA1062: Validate arguments of public methods * Replaced Wox.Infrastructure.Logger with Wox.Plugin.Logger
75 lines
2.2 KiB
C#
75 lines
2.2 KiB
C#
// Copyright (c) Microsoft Corporation
|
|
// The Microsoft Corporation licenses this file to you under the MIT license.
|
|
// See the LICENSE file in the project root for more information.
|
|
|
|
using System;
|
|
using System.Diagnostics;
|
|
using System.Globalization;
|
|
using System.Windows;
|
|
using System.Windows.Threading;
|
|
using NLog;
|
|
using Wox.Infrastructure;
|
|
using Wox.Infrastructure.Exception;
|
|
using Wox.Plugin;
|
|
|
|
namespace PowerLauncher.Helper
|
|
{
|
|
public static class ErrorReporting
|
|
{
|
|
private static void Report(Exception e, bool waitForClose)
|
|
{
|
|
if (e != null)
|
|
{
|
|
var logger = LogManager.GetLogger("UnHandledException");
|
|
logger.Fatal(ExceptionFormatter.FormatException(e));
|
|
|
|
var reportWindow = new ReportWindow(e);
|
|
|
|
if (waitForClose)
|
|
{
|
|
reportWindow.ShowDialog();
|
|
}
|
|
else
|
|
{
|
|
reportWindow.Show();
|
|
}
|
|
}
|
|
}
|
|
|
|
public static void ShowMessageBox(string title, string message)
|
|
{
|
|
Application.Current.Dispatcher.Invoke(() =>
|
|
{
|
|
MessageBox.Show(message, title);
|
|
});
|
|
}
|
|
|
|
public static void UnhandledExceptionHandle(object sender, UnhandledExceptionEventArgs e)
|
|
{
|
|
// handle non-ui thread exceptions
|
|
System.Windows.Application.Current.Dispatcher.Invoke(() =>
|
|
{
|
|
Report((Exception)e?.ExceptionObject, true);
|
|
});
|
|
}
|
|
|
|
public static void DispatcherUnhandledException(object sender, DispatcherUnhandledExceptionEventArgs e)
|
|
{
|
|
// handle ui thread exceptions
|
|
Report(e?.Exception, false);
|
|
|
|
// prevent application exist, so the user can copy prompted error info
|
|
e.Handled = true;
|
|
}
|
|
|
|
public static string RuntimeInfo()
|
|
{
|
|
var info = $"\nVersion: {Constant.Version}" +
|
|
$"\nOS Version: {Environment.OSVersion.VersionString}" +
|
|
$"\nIntPtr Length: {IntPtr.Size}" +
|
|
$"\nx64: {Environment.Is64BitOperatingSystem}";
|
|
return info;
|
|
}
|
|
}
|
|
}
|