mirror of
https://github.com/microsoft/PowerToys.git
synced 2024-12-21 07:17:56 +08:00
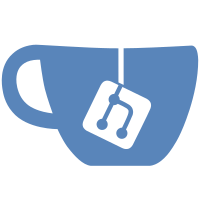
* Fix HotkeyControl virtual key display * A new interop project was setup to provide wrappers for C# projects that want to access functionality in the common project. * Add assembly info * Remove WIN32 configurations
63 lines
2.2 KiB
C++
63 lines
2.2 KiB
C++
#pragma once
|
|
#include <vector>
|
|
#include <winrt/Windows.System.h>
|
|
#include <winrt/Windows.Foundation.h>
|
|
|
|
namespace KeyboardManagerHelper
|
|
{
|
|
// Type to distinguish between keys
|
|
enum class KeyType
|
|
{
|
|
Win,
|
|
Ctrl,
|
|
Alt,
|
|
Shift,
|
|
Action
|
|
};
|
|
|
|
// Function to split a wstring based on a delimiter and return a vector of split strings
|
|
std::vector<std::wstring> splitwstring(const std::wstring& input, wchar_t delimiter);
|
|
|
|
// Function to return the next sibling element for an element under a stack panel
|
|
winrt::Windows::Foundation::IInspectable getSiblingElement(winrt::Windows::Foundation::IInspectable const& element);
|
|
|
|
// Function to convert an unsigned int vector to hstring by concatenating them
|
|
template<typename T>
|
|
winrt::hstring convertVectorToHstring(const std::vector<T>& input)
|
|
{
|
|
winrt::hstring output;
|
|
for (int i = 0; i < input.size(); i++)
|
|
{
|
|
output = output + winrt::to_hstring((unsigned int)input[i]) + winrt::to_hstring(L" ");
|
|
}
|
|
return output;
|
|
}
|
|
|
|
// Function to convert a wstring vector to a integer vector
|
|
template<typename T>
|
|
std::vector<T> convertWStringVectorToIntegerVector(const std::vector<std::wstring>& input)
|
|
{
|
|
std::vector<T> typeVector;
|
|
for (int i = 0; i < input.size(); i++)
|
|
{
|
|
typeVector.push_back((T)std::stoi(input[i]));
|
|
}
|
|
|
|
return typeVector;
|
|
}
|
|
|
|
// Function to return if the key is an extended key which requires the use of the extended key flag
|
|
bool isExtendedKey(DWORD key);
|
|
|
|
// Function to check if the key is a modifier key
|
|
bool IsModifierKey(DWORD key);
|
|
|
|
// Function to get the type of the key
|
|
KeyType GetKeyType(DWORD key);
|
|
|
|
// Function to return if the key is an extended key which requires the use of the extended key flag
|
|
bool isExtendedKey(DWORD key);
|
|
|
|
// Function to return the list of key name in the order for the drop down based on the key codes
|
|
winrt::Windows::Foundation::Collections::IVector<winrt::Windows::Foundation::IInspectable> ToBoxValue(const std::vector<std::wstring>& list);
|
|
} |