mirror of
https://github.com/microsoft/PowerToys.git
synced 2024-12-23 00:17:58 +08:00
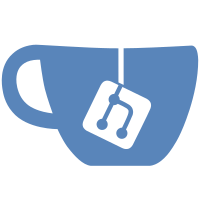
* Initial commit * Changed some loggers (WIP) * ColorPicker * Add version to all logs * FancyZones * push * PowerOCR and Measuretool * Settings * Hosts + Fix settings * Fix some using statements * FileLocksmith * Fix awake * Fixed Hosts logger * Fix spelling * Remove added submodule * Fiy FileLocksmith and PowerAccent * Fix PowerAccent * Test * Changed logger locic and added ColorPicker * Fixed package * Add documentation * Add locallow capability to Logger and add FancyZones * Fixed FancyZones and added FileLocksmith * Add Hosts * Fixed spelling mistakes * Add MeasureTool * Add MouseJump * Add PowerOCR * Add PowerAccent * Add monaco * Add Settings * Fixed Monaco * Update installer * Update doc/devdocs/logging.md Co-authored-by: Stefan Markovic <57057282+stefansjfw@users.noreply.github.com> * Update doc/devdocs/logging.md Co-authored-by: Stefan Markovic <57057282+stefansjfw@users.noreply.github.com> * Update doc/devdocs/logging.md Co-authored-by: Stefan Markovic <57057282+stefansjfw@users.noreply.github.com> * Update logging.md * Fix unneccesairy includes. --------- Co-authored-by: Dustin L. Howett <dustin@howett.net> Co-authored-by: Stefan Markovic <stefan@janeasystems.com> Co-authored-by: Stefan Markovic <57057282+stefansjfw@users.noreply.github.com>
277 lines
9.0 KiB
C#
277 lines
9.0 KiB
C#
// Copyright (c) Microsoft Corporation
|
|
// The Microsoft Corporation licenses this file to you under the MIT license.
|
|
// See the LICENSE file in the project root for more information.
|
|
|
|
using System;
|
|
using System.IO;
|
|
using System.Runtime.CompilerServices;
|
|
using global::PowerToys.GPOWrapper;
|
|
using ManagedCommon;
|
|
using Microsoft.PowerToys.Settings.UI.Library;
|
|
using Microsoft.PowerToys.Settings.UI.Library.Helpers;
|
|
using Microsoft.PowerToys.Settings.UI.Library.Interfaces;
|
|
|
|
namespace Microsoft.PowerToys.Settings.UI.ViewModels
|
|
{
|
|
public class PowerRenameViewModel : Observable
|
|
{
|
|
private GeneralSettings GeneralSettingsConfig { get; set; }
|
|
|
|
private readonly ISettingsUtils _settingsUtils;
|
|
|
|
private const string ModuleName = PowerRenameSettings.ModuleName;
|
|
|
|
private string _settingsConfigFileFolder = string.Empty;
|
|
|
|
private PowerRenameSettings Settings { get; set; }
|
|
|
|
private Func<string, int> SendConfigMSG { get; }
|
|
|
|
public PowerRenameViewModel(ISettingsUtils settingsUtils, ISettingsRepository<GeneralSettings> settingsRepository, Func<string, int> ipcMSGCallBackFunc, string configFileSubfolder = "")
|
|
{
|
|
// Update Settings file folder:
|
|
_settingsConfigFileFolder = configFileSubfolder;
|
|
_settingsUtils = settingsUtils ?? throw new ArgumentNullException(nameof(settingsUtils));
|
|
|
|
if (settingsRepository == null)
|
|
{
|
|
throw new ArgumentNullException(nameof(settingsRepository));
|
|
}
|
|
|
|
GeneralSettingsConfig = settingsRepository.SettingsConfig;
|
|
|
|
try
|
|
{
|
|
PowerRenameLocalProperties localSettings = _settingsUtils.GetSettingsOrDefault<PowerRenameLocalProperties>(GetSettingsSubPath(), "power-rename-settings.json");
|
|
Settings = new PowerRenameSettings(localSettings);
|
|
}
|
|
catch (Exception e)
|
|
{
|
|
Logger.LogError($"Exception encountered while reading {ModuleName} settings.", e);
|
|
#if DEBUG
|
|
if (e is ArgumentException || e is ArgumentNullException || e is PathTooLongException)
|
|
{
|
|
throw;
|
|
}
|
|
#endif
|
|
PowerRenameLocalProperties localSettings = new PowerRenameLocalProperties();
|
|
Settings = new PowerRenameSettings(localSettings);
|
|
_settingsUtils.SaveSettings(localSettings.ToJsonString(), GetSettingsSubPath(), "power-rename-settings.json");
|
|
}
|
|
|
|
// set the callback functions value to hangle outgoing IPC message.
|
|
SendConfigMSG = ipcMSGCallBackFunc;
|
|
|
|
_powerRenameEnabledOnContextMenu = Settings.Properties.ShowIcon.Value;
|
|
_powerRenameEnabledOnContextExtendedMenu = Settings.Properties.ExtendedContextMenuOnly.Value;
|
|
_powerRenameRestoreFlagsOnLaunch = Settings.Properties.PersistState.Value;
|
|
_powerRenameMaxDispListNumValue = Settings.Properties.MaxMRUSize.Value;
|
|
_autoComplete = Settings.Properties.MRUEnabled.Value;
|
|
_powerRenameUseBoostLib = Settings.Properties.UseBoostLib.Value;
|
|
|
|
InitializeEnabledValue();
|
|
}
|
|
|
|
private void InitializeEnabledValue()
|
|
{
|
|
_enabledGpoRuleConfiguration = GPOWrapper.GetConfiguredPowerRenameEnabledValue();
|
|
if (_enabledGpoRuleConfiguration == GpoRuleConfigured.Disabled || _enabledGpoRuleConfiguration == GpoRuleConfigured.Enabled)
|
|
{
|
|
// Get the enabled state from GPO.
|
|
_enabledStateIsGPOConfigured = true;
|
|
_powerRenameEnabled = _enabledGpoRuleConfiguration == GpoRuleConfigured.Enabled;
|
|
}
|
|
else
|
|
{
|
|
_powerRenameEnabled = GeneralSettingsConfig.Enabled.PowerRename;
|
|
}
|
|
}
|
|
|
|
private GpoRuleConfigured _enabledGpoRuleConfiguration;
|
|
private bool _enabledStateIsGPOConfigured;
|
|
private bool _powerRenameEnabled;
|
|
private bool _powerRenameEnabledOnContextMenu;
|
|
private bool _powerRenameEnabledOnContextExtendedMenu;
|
|
private bool _powerRenameRestoreFlagsOnLaunch;
|
|
private int _powerRenameMaxDispListNumValue;
|
|
private bool _autoComplete;
|
|
private bool _powerRenameUseBoostLib;
|
|
|
|
public bool IsEnabled
|
|
{
|
|
get
|
|
{
|
|
return _powerRenameEnabled;
|
|
}
|
|
|
|
set
|
|
{
|
|
if (_enabledStateIsGPOConfigured)
|
|
{
|
|
// If it's GPO configured, shouldn't be able to change this state.
|
|
return;
|
|
}
|
|
|
|
if (value != _powerRenameEnabled)
|
|
{
|
|
GeneralSettingsConfig.Enabled.PowerRename = value;
|
|
OutGoingGeneralSettings snd = new OutGoingGeneralSettings(GeneralSettingsConfig);
|
|
|
|
SendConfigMSG(snd.ToString());
|
|
|
|
_powerRenameEnabled = value;
|
|
OnPropertyChanged(nameof(IsEnabled));
|
|
RaisePropertyChanged(nameof(GlobalAndMruEnabled));
|
|
}
|
|
}
|
|
}
|
|
|
|
public bool IsEnabledGpoConfigured
|
|
{
|
|
get => _enabledStateIsGPOConfigured;
|
|
}
|
|
|
|
public bool MRUEnabled
|
|
{
|
|
get
|
|
{
|
|
return _autoComplete;
|
|
}
|
|
|
|
set
|
|
{
|
|
if (value != _autoComplete)
|
|
{
|
|
_autoComplete = value;
|
|
Settings.Properties.MRUEnabled.Value = value;
|
|
RaisePropertyChanged();
|
|
RaisePropertyChanged(nameof(GlobalAndMruEnabled));
|
|
}
|
|
}
|
|
}
|
|
|
|
public bool GlobalAndMruEnabled
|
|
{
|
|
get
|
|
{
|
|
return _autoComplete && _powerRenameEnabled;
|
|
}
|
|
}
|
|
|
|
public bool EnabledOnContextMenu
|
|
{
|
|
get
|
|
{
|
|
return _powerRenameEnabledOnContextMenu;
|
|
}
|
|
|
|
set
|
|
{
|
|
if (value != _powerRenameEnabledOnContextMenu)
|
|
{
|
|
_powerRenameEnabledOnContextMenu = value;
|
|
Settings.Properties.ShowIcon.Value = value;
|
|
RaisePropertyChanged();
|
|
}
|
|
}
|
|
}
|
|
|
|
public bool EnabledOnContextExtendedMenu
|
|
{
|
|
get
|
|
{
|
|
return _powerRenameEnabledOnContextExtendedMenu;
|
|
}
|
|
|
|
set
|
|
{
|
|
if (value != _powerRenameEnabledOnContextExtendedMenu)
|
|
{
|
|
_powerRenameEnabledOnContextExtendedMenu = value;
|
|
Settings.Properties.ExtendedContextMenuOnly.Value = value;
|
|
RaisePropertyChanged();
|
|
}
|
|
}
|
|
}
|
|
|
|
public bool RestoreFlagsOnLaunch
|
|
{
|
|
get
|
|
{
|
|
return _powerRenameRestoreFlagsOnLaunch;
|
|
}
|
|
|
|
set
|
|
{
|
|
if (value != _powerRenameRestoreFlagsOnLaunch)
|
|
{
|
|
_powerRenameRestoreFlagsOnLaunch = value;
|
|
Settings.Properties.PersistState.Value = value;
|
|
RaisePropertyChanged();
|
|
}
|
|
}
|
|
}
|
|
|
|
public int MaxDispListNum
|
|
{
|
|
get
|
|
{
|
|
return _powerRenameMaxDispListNumValue;
|
|
}
|
|
|
|
set
|
|
{
|
|
if (value != _powerRenameMaxDispListNumValue)
|
|
{
|
|
_powerRenameMaxDispListNumValue = value;
|
|
Settings.Properties.MaxMRUSize.Value = value;
|
|
RaisePropertyChanged();
|
|
}
|
|
}
|
|
}
|
|
|
|
public bool UseBoostLib
|
|
{
|
|
get
|
|
{
|
|
return _powerRenameUseBoostLib;
|
|
}
|
|
|
|
set
|
|
{
|
|
if (value != _powerRenameUseBoostLib)
|
|
{
|
|
_powerRenameUseBoostLib = value;
|
|
Settings.Properties.UseBoostLib.Value = value;
|
|
RaisePropertyChanged();
|
|
}
|
|
}
|
|
}
|
|
|
|
public string GetSettingsSubPath()
|
|
{
|
|
return _settingsConfigFileFolder + "\\" + ModuleName;
|
|
}
|
|
|
|
private void RaisePropertyChanged([CallerMemberName] string propertyName = null)
|
|
{
|
|
// Notify UI of property change
|
|
OnPropertyChanged(propertyName);
|
|
|
|
if (SendConfigMSG != null)
|
|
{
|
|
SndPowerRenameSettings snd = new SndPowerRenameSettings(Settings);
|
|
SndModuleSettings<SndPowerRenameSettings> ipcMessage = new SndModuleSettings<SndPowerRenameSettings>(snd);
|
|
SendConfigMSG(ipcMessage.ToJsonString());
|
|
}
|
|
}
|
|
|
|
public void RefreshEnabledState()
|
|
{
|
|
InitializeEnabledValue();
|
|
OnPropertyChanged(nameof(IsEnabled));
|
|
OnPropertyChanged(nameof(GlobalAndMruEnabled));
|
|
}
|
|
}
|
|
}
|