mirror of
https://github.com/microsoft/PowerToys.git
synced 2024-12-26 18:58:29 +08:00
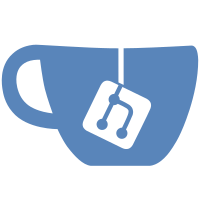
* Added tests for loading and saving remappings in the UI * Added tests for ApplyRemappings methods * Moved single key remap validation logic to separate method so that it can be tested * Added tests for single key remap validation in UI * Refactored shortcut validation code to be testable * Added some shortcut validation tests * Refactored code to be cleaner * Added tests for action key and modifier key selection and formatted file * Added tests for selecting None * Added tests for selecting Null * Added tests for WinL error * Added CtrlAltDel tests * Added tests for MapToSameKey * Added tests for mapping repeated shortcut * Fixed const correctness * Clean up type names with type alias * Clean up ValidateAndUpdateKeyBufferElement tests and tweak ValidateShortcutBufferElement signature * Fixed bug when None selected * Refactored one test as per test case framework * Cleaned up more tests * Cleaned up buffer validation tests * Added tests for KBM Common Helpers and Shortcut
28 lines
1.4 KiB
C++
28 lines
1.4 KiB
C++
#pragma once
|
|
#include <vector>
|
|
#include <keyboardmanager/common/Helpers.h>
|
|
#include <variant>
|
|
|
|
class KeyboardManagerState;
|
|
|
|
namespace LoadingAndSavingRemappingHelper
|
|
{
|
|
// Function to check if the set of remappings in the buffer are valid
|
|
KeyboardManagerHelper::ErrorType CheckIfRemappingsAreValid(const RemapBuffer& remappings);
|
|
|
|
// Function to return the set of keys that have been orphaned from the remap buffer
|
|
std::vector<DWORD> GetOrphanedKeys(const RemapBuffer& remappings);
|
|
|
|
// Function to combine remappings if the L and R version of the modifier is mapped to the same key
|
|
void CombineRemappings(std::unordered_map<DWORD, std::variant<DWORD, Shortcut>>& table, DWORD leftKey, DWORD rightKey, DWORD combinedKey);
|
|
|
|
// Function to pre process the remap table before loading it into the UI
|
|
void PreProcessRemapTable(std::unordered_map<DWORD, std::variant<DWORD, Shortcut>>& table);
|
|
|
|
// Function to apply the single key remappings from the buffer to the KeyboardManagerState variable
|
|
void ApplySingleKeyRemappings(KeyboardManagerState& keyboardManagerState, const RemapBuffer& remappings, bool isTelemetryRequired);
|
|
|
|
// Function to apply the shortcut remappings from the buffer to the KeyboardManagerState variable
|
|
void ApplyShortcutRemappings(KeyboardManagerState& keyboardManagerState, const RemapBuffer& remappings, bool isTelemetryRequired);
|
|
}
|