mirror of
https://github.com/microsoft/PowerToys.git
synced 2025-01-12 15:39:33 +08:00
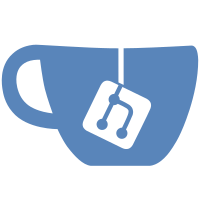
* dotnet sc * MD preview - C# app - working self-contained * Gcode preview - C# app * DevFiles preview - C# app * Fix passing path with spaces as cmd arg and monacocpp proj file * Pdf preview - C# app * Svg preview - C# app * Fix comment * Gcode thumbnail - C# app TODO: - installer - why IThumbnailProvider and IIntializeWithFile doesn't work? * Pdf thumbnail - C# app TODO: - installer - why IThumbnailProvider and IIntializeWithFile doesn't work? * Pdf thumbnail - C# app TODO: - installer - why IThumbnailProvider and IIntializeWithFile doesn't work? * Fix GcodeThumbnailProviderCpp.vcxproj * Svg thumbnail - C# app TODO: - installer - why IThumbnailProvider and IIntializeWithFile doesn't work? * Fix Svg tests * Thumbnail providers - installer * Self-contained Hosts and FileLocksmith * Fix hardcoded <RuntimeIdentifier> * Remove unneeded files * Try to fix Nuget in PR CI * Prefix new dlls with PowerToys. Sign new dlls and exes * Add new .exe files to ProcessList * ci: debug by listing all env vars * ci: try setting variable in the right ci file * Bring back hardcoded RuntimeIdentifier * ci: Add comment and remove debug action * Remove unneeded lib * [WIP] Platform conditional dotnet files & hardlinks * Cleanup * Update expect.txt * Test fix - ARM installer * Fix uninstall bug * Update docs * Fix failing test * Add dll details * Minor cleanup * Improve resizing * Add some logs * Test fix - release build * Remove InvokeOnControlThread * Test fix: logger initialization * Fix arm64 installer Co-authored-by: Jaime Bernardo <jaime@janeasystems.com> Co-authored-by: Dustin L. Howett <dustin@howett.net>
74 lines
2.0 KiB
C++
74 lines
2.0 KiB
C++
// dllmain.cpp : Defines the entry point for the DLL application.
|
|
#include "pch.h"
|
|
#include "ClassFactory.h"
|
|
|
|
HINSTANCE g_hInst = NULL;
|
|
long g_cDllRef = 0;
|
|
|
|
// {77257004-6F25-4521-B602-50ECC6EC62A6}
|
|
static const GUID CLSID_StlThumbnailProvider = { 0x77257004, 0x6f25, 0x4521, { 0xb6, 0x2, 0x50, 0xec, 0xc6, 0xec, 0x62, 0xa6 } };
|
|
|
|
BOOL APIENTRY DllMain(HMODULE hModule,
|
|
DWORD ul_reason_for_call,
|
|
LPVOID lpReserved)
|
|
{
|
|
switch (ul_reason_for_call)
|
|
{
|
|
case DLL_PROCESS_ATTACH:
|
|
g_hInst = hModule;
|
|
DisableThreadLibraryCalls(hModule);
|
|
break;
|
|
case DLL_THREAD_ATTACH:
|
|
case DLL_THREAD_DETACH:
|
|
case DLL_PROCESS_DETACH:
|
|
break;
|
|
}
|
|
return TRUE;
|
|
}
|
|
|
|
//
|
|
// FUNCTION: DllGetClassObject
|
|
//
|
|
// PURPOSE: Create the class factory and query to the specific interface.
|
|
//
|
|
// PARAMETERS:
|
|
// * rclsid - The CLSID that will associate the correct data and code.
|
|
// * riid - A reference to the identifier of the interface that the caller
|
|
// is to use to communicate with the class object.
|
|
// * ppv - The address of a pointer variable that receives the interface
|
|
// pointer requested in riid. Upon successful return, *ppv contains the
|
|
// requested interface pointer. If an error occurs, the interface pointer
|
|
// is NULL.
|
|
//
|
|
STDAPI DllGetClassObject(REFCLSID rclsid, REFIID riid, void** ppv)
|
|
{
|
|
HRESULT hr = CLASS_E_CLASSNOTAVAILABLE;
|
|
|
|
if (IsEqualCLSID(CLSID_StlThumbnailProvider, rclsid))
|
|
{
|
|
hr = E_OUTOFMEMORY;
|
|
|
|
ClassFactory* pClassFactory = new ClassFactory();
|
|
if (pClassFactory)
|
|
{
|
|
hr = pClassFactory->QueryInterface(riid, ppv);
|
|
pClassFactory->Release();
|
|
}
|
|
}
|
|
|
|
return hr;
|
|
}
|
|
|
|
//
|
|
// FUNCTION: DllCanUnloadNow
|
|
//
|
|
// PURPOSE: Check if we can unload the component from the memory.
|
|
//
|
|
// NOTE: The component can be unloaded from the memory when its reference
|
|
// count is zero (i.e. nobody is still using the component).
|
|
//
|
|
STDAPI DllCanUnloadNow(void)
|
|
{
|
|
return g_cDllRef > 0 ? S_FALSE : S_OK;
|
|
}
|