mirror of
https://github.com/microsoft/PowerToys.git
synced 2024-12-21 15:27:55 +08:00
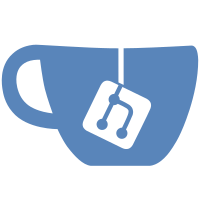
* Add SVG Thumbnail Provider * Some cleanup * Small settings changes * Update PowerToys.sln Remove Any CPU entries * Fix project configuration issues * Fix bad merge * Update output path for SVG thumbnail provider * Sync with latest
107 lines
3.2 KiB
C#
107 lines
3.2 KiB
C#
// Copyright (c) Microsoft Corporation
|
|
// The Microsoft Corporation licenses this file to you under the MIT license.
|
|
// See the LICENSE file in the project root for more information.
|
|
|
|
using System.Runtime.CompilerServices;
|
|
using Microsoft.PowerToys.Settings.UI.Helpers;
|
|
using Microsoft.PowerToys.Settings.UI.Lib;
|
|
using Microsoft.PowerToys.Settings.UI.Views;
|
|
|
|
namespace Microsoft.PowerToys.Settings.UI.ViewModels
|
|
{
|
|
public class PowerPreviewViewModel : Observable
|
|
{
|
|
private const string ModuleName = "File Explorer";
|
|
|
|
private PowerPreviewSettings Settings { get; set; }
|
|
|
|
public PowerPreviewViewModel()
|
|
{
|
|
try
|
|
{
|
|
Settings = SettingsUtils.GetSettings<PowerPreviewSettings>(ModuleName);
|
|
}
|
|
catch
|
|
{
|
|
Settings = new PowerPreviewSettings();
|
|
SettingsUtils.SaveSettings(Settings.ToJsonString(), ModuleName);
|
|
}
|
|
|
|
this._svgRenderIsEnabled = Settings.properties.EnableSvgPreview;
|
|
this._svgThumbnailIsEnabled = Settings.properties.EnableSvgThumbnail;
|
|
this._mdRenderIsEnabled = Settings.properties.EnableMdPreview;
|
|
}
|
|
|
|
private bool _svgRenderIsEnabled = false;
|
|
private bool _mdRenderIsEnabled = false;
|
|
private bool _svgThumbnailIsEnabled = false;
|
|
|
|
public bool SVGRenderIsEnabled
|
|
{
|
|
get
|
|
{
|
|
return _svgRenderIsEnabled;
|
|
}
|
|
|
|
set
|
|
{
|
|
if (value != _svgRenderIsEnabled)
|
|
{
|
|
_svgRenderIsEnabled = value;
|
|
Settings.properties.EnableSvgPreview = value;
|
|
RaisePropertyChanged();
|
|
}
|
|
}
|
|
}
|
|
|
|
public bool SVGThumbnailIsEnabled
|
|
{
|
|
get
|
|
{
|
|
return _svgThumbnailIsEnabled;
|
|
}
|
|
|
|
set
|
|
{
|
|
if (value != _svgThumbnailIsEnabled)
|
|
{
|
|
_svgThumbnailIsEnabled = value;
|
|
Settings.properties.EnableSvgThumbnail = value;
|
|
RaisePropertyChanged();
|
|
}
|
|
}
|
|
}
|
|
|
|
public bool MDRenderIsEnabled
|
|
{
|
|
get
|
|
{
|
|
return _mdRenderIsEnabled;
|
|
}
|
|
|
|
set
|
|
{
|
|
if (value != _mdRenderIsEnabled)
|
|
{
|
|
_mdRenderIsEnabled = value;
|
|
Settings.properties.EnableMdPreview = value;
|
|
RaisePropertyChanged();
|
|
}
|
|
}
|
|
}
|
|
|
|
private void RaisePropertyChanged([CallerMemberName] string propertyName = null)
|
|
{
|
|
// Notify UI of property change
|
|
OnPropertyChanged(propertyName);
|
|
|
|
if (ShellPage.DefaultSndMSGCallback != null)
|
|
{
|
|
SndPowerPreviewSettings snd = new SndPowerPreviewSettings(Settings);
|
|
SndModuleSettings<SndPowerPreviewSettings> ipcMessage = new SndModuleSettings<SndPowerPreviewSettings>(snd);
|
|
ShellPage.DefaultSndMSGCallback(ipcMessage.ToJsonString());
|
|
}
|
|
}
|
|
}
|
|
}
|