mirror of
https://github.com/microsoft/PowerToys.git
synced 2024-12-23 00:17:58 +08:00
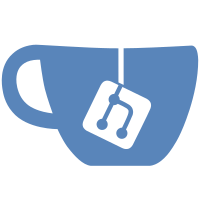
* Fix OOBE size and make it non-resizable Bring back Settings window placement preserve logic * Disable OOBE maximize&minimize * expect.txt * Remove uneeded line * Remove uneeded check * Add brackets
117 lines
3.9 KiB
C#
117 lines
3.9 KiB
C#
// Copyright (c) Microsoft Corporation
|
|
// The Microsoft Corporation licenses this file to you under the MIT license.
|
|
// See the LICENSE file in the project root for more information.
|
|
|
|
using System;
|
|
using System.Drawing;
|
|
using Microsoft.PowerLauncher.Telemetry;
|
|
using Microsoft.PowerToys.Settings.UI.Helpers;
|
|
using Microsoft.PowerToys.Settings.UI.Library.Utilities;
|
|
using Microsoft.PowerToys.Settings.UI.Views;
|
|
using Microsoft.PowerToys.Telemetry;
|
|
using Microsoft.UI;
|
|
using Microsoft.UI.Windowing;
|
|
using Microsoft.UI.Xaml;
|
|
using Windows.ApplicationModel.Resources;
|
|
using Windows.Data.Json;
|
|
|
|
namespace Microsoft.PowerToys.Settings.UI
|
|
{
|
|
/// <summary>
|
|
/// An empty window that can be used on its own or navigated to within a Frame.
|
|
/// </summary>
|
|
public sealed partial class MainWindow : Window
|
|
{
|
|
public MainWindow()
|
|
{
|
|
var bootTime = new System.Diagnostics.Stopwatch();
|
|
bootTime.Start();
|
|
|
|
ShellPage.SetElevationStatus(App.IsElevated);
|
|
ShellPage.SetIsUserAnAdmin(App.IsUserAnAdmin);
|
|
|
|
// Set window icon
|
|
var hWnd = WinRT.Interop.WindowNative.GetWindowHandle(this);
|
|
WindowId windowId = Win32Interop.GetWindowIdFromWindow(hWnd);
|
|
AppWindow appWindow = AppWindow.GetFromWindowId(windowId);
|
|
appWindow.SetIcon("icon.ico");
|
|
|
|
var placement = Utils.DeserializePlacementOrDefault(hWnd);
|
|
NativeMethods.SetWindowPlacement(hWnd, ref placement);
|
|
|
|
ResourceLoader loader = ResourceLoader.GetForViewIndependentUse();
|
|
Title = loader.GetString("SettingsWindow_Title");
|
|
|
|
// send IPC Message
|
|
ShellPage.SetDefaultSndMessageCallback(msg =>
|
|
{
|
|
// IPC Manager is null when launching runner directly
|
|
App.GetTwoWayIPCManager()?.Send(msg);
|
|
});
|
|
|
|
// send IPC Message
|
|
ShellPage.SetRestartAdminSndMessageCallback(msg =>
|
|
{
|
|
App.GetTwoWayIPCManager()?.Send(msg);
|
|
Environment.Exit(0); // close application
|
|
});
|
|
|
|
// send IPC Message
|
|
ShellPage.SetCheckForUpdatesMessageCallback(msg =>
|
|
{
|
|
App.GetTwoWayIPCManager()?.Send(msg);
|
|
});
|
|
|
|
// open oobe
|
|
ShellPage.SetOpenOobeCallback(() =>
|
|
{
|
|
if (App.GetOobeWindow() == null)
|
|
{
|
|
App.SetOobeWindow(new OobeWindow(Microsoft.PowerToys.Settings.UI.OOBE.Enums.PowerToysModules.Overview));
|
|
}
|
|
|
|
App.GetOobeWindow().Activate();
|
|
});
|
|
|
|
this.InitializeComponent();
|
|
|
|
// receive IPC Message
|
|
App.IPCMessageReceivedCallback = (string msg) =>
|
|
{
|
|
if (ShellPage.ShellHandler.IPCResponseHandleList != null)
|
|
{
|
|
var success = JsonObject.TryParse(msg, out JsonObject json);
|
|
if (success)
|
|
{
|
|
foreach (Action<JsonObject> handle in ShellPage.ShellHandler.IPCResponseHandleList)
|
|
{
|
|
handle(json);
|
|
}
|
|
}
|
|
else
|
|
{
|
|
Logger.LogError("Failed to parse JSON from IPC message.");
|
|
}
|
|
}
|
|
};
|
|
|
|
bootTime.Stop();
|
|
|
|
PowerToysTelemetry.Log.WriteEvent(new SettingsBootEvent() { BootTimeMs = bootTime.ElapsedMilliseconds });
|
|
}
|
|
|
|
public void NavigateToSection(System.Type type)
|
|
{
|
|
ShellPage.Navigate(type);
|
|
}
|
|
|
|
private void Window_Closed(object sender, WindowEventArgs args)
|
|
{
|
|
App.ClearSettingsWindow();
|
|
|
|
var hWnd = WinRT.Interop.WindowNative.GetWindowHandle(this);
|
|
Utils.SerializePlacement(hWnd);
|
|
}
|
|
}
|
|
}
|