mirror of
https://github.com/microsoft/PowerToys.git
synced 2024-12-26 10:48:23 +08:00
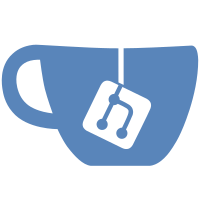
* Enable app specific shortcut remapping * Fixed lowercase function call * Add test file * Moved GetForegroundProcess to II and added tests * Fixed runtime error while testing due to heap allocation across dll boundary * Renamed function * Changed shortcutBuffer type * Linked App specific UI to backend * Added shortcut validation logic on TextBox LostFocus handler * Moved Validate function and changed default text * Changed to case insensitive warning check * Changed to case insensitive warning check at OnClickAccept * Fixed alignment and spacing issues * Added app-specific JSON support in backend * Updated landing page * Make listview horizontally scrollable * Added tests * Consider all case variants of All Apps in textbox to be global shortcuts
33 lines
979 B
C#
33 lines
979 B
C#
// Copyright (c) Microsoft Corporation
|
|
// The Microsoft Corporation licenses this file to you under the MIT license.
|
|
// See the LICENSE file in the project root for more information.
|
|
|
|
using System.Collections.Generic;
|
|
using System.Linq;
|
|
using System.Text.Json.Serialization;
|
|
using Microsoft.PowerToys.Settings.UI.Lib.Utilities;
|
|
|
|
namespace Microsoft.PowerToys.Settings.UI.Lib
|
|
{
|
|
public class AppSpecificKeysDataModel : KeysDataModel
|
|
{
|
|
[JsonPropertyName("targetApp")]
|
|
public string TargetApp { get; set; }
|
|
|
|
public new List<string> GetOriginalKeys()
|
|
{
|
|
return base.GetOriginalKeys();
|
|
}
|
|
|
|
public new List<string> GetNewRemapKeys()
|
|
{
|
|
return base.GetNewRemapKeys();
|
|
}
|
|
|
|
public bool Compare(AppSpecificKeysDataModel arg)
|
|
{
|
|
return OriginalKeys.Equals(arg.OriginalKeys) && NewRemapKeys.Equals(arg.NewRemapKeys) && TargetApp.Equals(arg.TargetApp);
|
|
}
|
|
}
|
|
}
|