mirror of
https://github.com/microsoft/PowerToys.git
synced 2024-12-26 10:48:23 +08:00
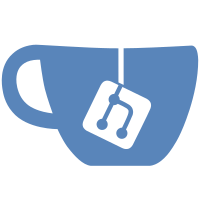
* Enable app specific shortcut remapping * Fixed lowercase function call * Add test file * Moved GetForegroundProcess to II and added tests * Fixed runtime error while testing due to heap allocation across dll boundary * Renamed function * Changed shortcutBuffer type * Linked App specific UI to backend * Added shortcut validation logic on TextBox LostFocus handler * Moved Validate function and changed default text * Changed to case insensitive warning check * Changed to case insensitive warning check at OnClickAccept * Fixed alignment and spacing issues * Added app-specific JSON support in backend * Updated landing page * Make listview horizontally scrollable * Added tests * Consider all case variants of All Apps in textbox to be global shortcuts
40 lines
1.1 KiB
C#
40 lines
1.1 KiB
C#
// Copyright (c) Microsoft Corporation
|
|
// The Microsoft Corporation licenses this file to you under the MIT license.
|
|
// See the LICENSE file in the project root for more information.
|
|
|
|
using System.Collections.Generic;
|
|
using System.Linq;
|
|
using System.Text.Json.Serialization;
|
|
using Microsoft.PowerToys.Settings.UI.Lib.Utilities;
|
|
|
|
namespace Microsoft.PowerToys.Settings.UI.Lib
|
|
{
|
|
public class KeysDataModel
|
|
{
|
|
[JsonPropertyName("originalKeys")]
|
|
public string OriginalKeys { get; set; }
|
|
|
|
[JsonPropertyName("newRemapKeys")]
|
|
public string NewRemapKeys { get; set; }
|
|
|
|
private List<string> MapKeys(string stringOfKeys)
|
|
{
|
|
return stringOfKeys
|
|
.Split(';')
|
|
.Select(uint.Parse)
|
|
.Select(Helper.GetKeyName)
|
|
.ToList();
|
|
}
|
|
|
|
public List<string> GetOriginalKeys()
|
|
{
|
|
return MapKeys(OriginalKeys);
|
|
}
|
|
|
|
public List<string> GetNewRemapKeys()
|
|
{
|
|
return MapKeys(NewRemapKeys);
|
|
}
|
|
}
|
|
}
|