mirror of
https://github.com/microsoft/PowerToys.git
synced 2024-12-20 23:07:55 +08:00
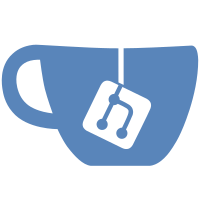
* C++ impl of immersive dark mode * Stop using the hardcoded value. * Conjured up theme listener based on registry. * Update MainWindow.xaml.cpp * Update expect.txt * Moved themehelpers to the common themes lib. * Ported theme helpers back to .NET * Update expect.txt * Updated C# Theme Listening logic to mimic the one from Windows Community Toolkit. * Replaced unmanaged code for RegisterForImmersiveDarkMode with unmanaged ThemeListener class. * Fix upstream changes * Update ThemeListener.h * Update ThemeListener.h * Proper formatting * Added handler to Keyboard Manager. * Update EditKeyboardWindow.cpp * Added dwmapi.lib to runner, removed condition from additional dependencies. * Update PowerRenameUI.vcxproj * Added new deps for ManagedCommon to Product.wxs * Crude attempts and understanding installer * Removed Microsoft.Win32.Registry.dll from product.wxs. * Updated dictionary * Renamed ThemeListener class file for consistency, removed unused CheckImmersiveDarkMode in theme_helpers. * Update Themes.vcxproj * Update theme_listener.cpp * Removed SupportsImmersiveDarkMode version check * Removed SupportsImmersiveDarkMode version check * Whoops * Update expect.txt
91 lines
3.0 KiB
C#
91 lines
3.0 KiB
C#
// Copyright (c) Microsoft Corporation
|
|
// The Microsoft Corporation licenses this file to you under the MIT license.
|
|
// See the LICENSE file in the project root for more information.
|
|
|
|
using System;
|
|
using System.Diagnostics.CodeAnalysis;
|
|
using Microsoft.PowerToys.Settings.UI.Library;
|
|
using Microsoft.PowerToys.Settings.UI.Library.Utilities;
|
|
using Microsoft.PowerToys.Settings.UI.Library.ViewModels;
|
|
using Microsoft.UI.Xaml;
|
|
using Microsoft.UI.Xaml.Controls;
|
|
using Windows.ApplicationModel.Resources;
|
|
using Windows.UI.Core;
|
|
|
|
namespace Microsoft.PowerToys.Settings.UI.Views
|
|
{
|
|
/// <summary>
|
|
/// General Settings Page.
|
|
/// </summary>
|
|
public sealed partial class GeneralPage : Page
|
|
{
|
|
/// <summary>
|
|
/// Gets or sets view model.
|
|
/// </summary>
|
|
public GeneralViewModel ViewModel { get; set; }
|
|
|
|
/// <summary>
|
|
/// Initializes a new instance of the <see cref="GeneralPage"/> class.
|
|
/// General Settings page constructor.
|
|
/// </summary>
|
|
public GeneralPage()
|
|
{
|
|
InitializeComponent();
|
|
|
|
// Load string resources
|
|
ResourceLoader loader = ResourceLoader.GetForViewIndependentUse();
|
|
var settingsUtils = new SettingsUtils();
|
|
|
|
Action stateUpdatingAction = () =>
|
|
{
|
|
this.DispatcherQueue.TryEnqueue(() =>
|
|
{
|
|
ViewModel.RefreshUpdatingState();
|
|
});
|
|
};
|
|
|
|
ViewModel = new GeneralViewModel(
|
|
SettingsRepository<GeneralSettings>.GetInstance(settingsUtils),
|
|
loader.GetString("GeneralSettings_RunningAsAdminText"),
|
|
loader.GetString("GeneralSettings_RunningAsUserText"),
|
|
ShellPage.IsElevated,
|
|
ShellPage.IsUserAnAdmin,
|
|
UpdateUIThemeMethod,
|
|
ShellPage.SendDefaultIPCMessage,
|
|
ShellPage.SendRestartAdminIPCMessage,
|
|
ShellPage.SendCheckForUpdatesIPCMessage,
|
|
string.Empty,
|
|
stateUpdatingAction);
|
|
|
|
DataContext = ViewModel;
|
|
}
|
|
|
|
public static int UpdateUIThemeMethod(string themeName)
|
|
{
|
|
switch (themeName?.ToUpperInvariant())
|
|
{
|
|
case "LIGHT":
|
|
ShellPage.ShellHandler.RequestedTheme = ElementTheme.Light;
|
|
break;
|
|
case "DARK":
|
|
ShellPage.ShellHandler.RequestedTheme = ElementTheme.Dark;
|
|
break;
|
|
case "SYSTEM":
|
|
ShellPage.ShellHandler.RequestedTheme = ElementTheme.Default;
|
|
break;
|
|
default:
|
|
Logger.LogError($"Unexpected theme name: {themeName}");
|
|
break;
|
|
}
|
|
|
|
App.HandleThemeChange();
|
|
return 0;
|
|
}
|
|
|
|
private void OpenColorsSettings_Click(object sender, RoutedEventArgs e)
|
|
{
|
|
Helpers.StartProcessHelper.Start(Helpers.StartProcessHelper.ColorsSettings);
|
|
}
|
|
}
|
|
}
|