mirror of
https://github.com/microsoft/PowerToys.git
synced 2025-01-04 01:57:57 +08:00
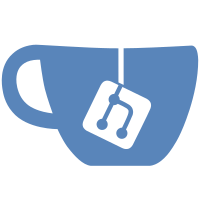
* Add GPOWrapper headers and C++/WinRT library * Check GPO before starting utilities * Show message on GPO having disabled preview panes. * Don't generate thumbnails if GPO disabled * Fix FancyZonesEditor unable to recognize GPOWrapper * Move settings view models to the settings project * Use GPO to block enabling utilities in Settings * Hide context menu entries when gpo disables utilities * Apply gpo policies when enabling PowerToys on runner * Add version and metadata to dll * Add GPOWrapper to the installer * Fix MSBuild errors on WPF apps by using Projection * Signing * Add gpo files and publish them * Add GPO policies to the bug report tool * Add some documentation for using GPO * Mention support to actual lowest supported version of Windows * Move PowerToys to the root of administrative templates tree * Save policies on Software\Policies\PowerToys * Support both machine and user scopes * Fix documentation to reference computer and user scopes * Mention incompatibility with outlook in gpo * Set a better folder structure for gpo assets * Move PDF Handler warning to the description * Update doc/gpo/README.md Co-authored-by: Heiko <61519853+htcfreek@users.noreply.github.com> * Add actual minimum version of PowerToys to gpo files * Fix identation * Remove GPOWrapper Readme * Add Active Directory instructions to doc Co-authored-by: Heiko <61519853+htcfreek@users.noreply.github.com>
241 lines
7.4 KiB
C#
241 lines
7.4 KiB
C#
// Copyright (c) Microsoft Corporation
|
|
// The Microsoft Corporation licenses this file to you under the MIT license.
|
|
// See the LICENSE file in the project root for more information.
|
|
|
|
using System;
|
|
using System.Globalization;
|
|
using System.Runtime.CompilerServices;
|
|
using System.Text.Json;
|
|
using global::PowerToys.GPOWrapper;
|
|
using Microsoft.PowerToys.Settings.UI.Library;
|
|
using Microsoft.PowerToys.Settings.UI.Library.Helpers;
|
|
using Microsoft.PowerToys.Settings.UI.Library.Interfaces;
|
|
|
|
namespace Microsoft.PowerToys.Settings.UI.ViewModels
|
|
{
|
|
public class MeasureToolViewModel : Observable
|
|
{
|
|
private ISettingsUtils SettingsUtils { get; set; }
|
|
|
|
private GeneralSettings GeneralSettingsConfig { get; set; }
|
|
|
|
private GpoRuleConfigured _enabledGpoRuleConfiguration;
|
|
private bool _enabledStateIsGPOConfigured;
|
|
private bool _isEnabled;
|
|
|
|
private MeasureToolSettings Settings { get; set; }
|
|
|
|
public MeasureToolViewModel(ISettingsUtils settingsUtils, ISettingsRepository<GeneralSettings> settingsRepository, ISettingsRepository<MeasureToolSettings> measureToolSettingsRepository, Func<string, int> ipcMSGCallBackFunc)
|
|
{
|
|
SettingsUtils = settingsUtils;
|
|
|
|
if (settingsRepository == null)
|
|
{
|
|
throw new ArgumentNullException(nameof(settingsRepository));
|
|
}
|
|
|
|
GeneralSettingsConfig = settingsRepository.SettingsConfig;
|
|
|
|
_enabledGpoRuleConfiguration = GPOWrapper.GetConfiguredScreenRulerEnabledValue();
|
|
if (_enabledGpoRuleConfiguration == GpoRuleConfigured.Disabled || _enabledGpoRuleConfiguration == GpoRuleConfigured.Enabled)
|
|
{
|
|
// Get the enabled state from GPO.
|
|
_enabledStateIsGPOConfigured = true;
|
|
_isEnabled = _enabledGpoRuleConfiguration == GpoRuleConfigured.Enabled;
|
|
}
|
|
else
|
|
{
|
|
_isEnabled = GeneralSettingsConfig.Enabled.MeasureTool;
|
|
}
|
|
|
|
if (measureToolSettingsRepository == null)
|
|
{
|
|
throw new ArgumentNullException(nameof(measureToolSettingsRepository));
|
|
}
|
|
|
|
Settings = measureToolSettingsRepository.SettingsConfig;
|
|
|
|
SendConfigMSG = ipcMSGCallBackFunc;
|
|
}
|
|
|
|
public bool IsEnabled
|
|
{
|
|
get => _isEnabled;
|
|
set
|
|
{
|
|
if (_enabledStateIsGPOConfigured)
|
|
{
|
|
// If it's GPO configured, shouldn't be able to change this state.
|
|
return;
|
|
}
|
|
|
|
if (_isEnabled != value)
|
|
{
|
|
_isEnabled = value;
|
|
GeneralSettingsConfig.Enabled.MeasureTool = value;
|
|
OnPropertyChanged(nameof(IsEnabled));
|
|
|
|
OutGoingGeneralSettings outgoing = new OutGoingGeneralSettings(GeneralSettingsConfig);
|
|
SendConfigMSG(outgoing.ToString());
|
|
|
|
NotifyPropertyChanged();
|
|
NotifyPropertyChanged(nameof(ShowContinuousCaptureWarning));
|
|
}
|
|
}
|
|
}
|
|
|
|
public bool IsEnabledGpoConfigured
|
|
{
|
|
get => _enabledStateIsGPOConfigured;
|
|
}
|
|
|
|
public bool ContinuousCapture
|
|
{
|
|
get
|
|
{
|
|
return Settings.Properties.ContinuousCapture;
|
|
}
|
|
|
|
set
|
|
{
|
|
if (Settings.Properties.ContinuousCapture != value)
|
|
{
|
|
Settings.Properties.ContinuousCapture = value;
|
|
NotifyPropertyChanged();
|
|
NotifyPropertyChanged(nameof(ShowContinuousCaptureWarning));
|
|
}
|
|
}
|
|
}
|
|
|
|
public bool DrawFeetOnCross
|
|
{
|
|
get
|
|
{
|
|
return Settings.Properties.DrawFeetOnCross;
|
|
}
|
|
|
|
set
|
|
{
|
|
if (Settings.Properties.DrawFeetOnCross != value)
|
|
{
|
|
Settings.Properties.DrawFeetOnCross = value;
|
|
NotifyPropertyChanged();
|
|
}
|
|
}
|
|
}
|
|
|
|
public string CrossColor
|
|
{
|
|
get
|
|
{
|
|
return Settings.Properties.MeasureCrossColor.Value;
|
|
}
|
|
|
|
set
|
|
{
|
|
value = (value != null) ? SettingsUtilities.ToRGBHex(value) : "#FF4500";
|
|
if (!value.Equals(Settings.Properties.MeasureCrossColor.Value, StringComparison.OrdinalIgnoreCase))
|
|
{
|
|
Settings.Properties.MeasureCrossColor.Value = value;
|
|
NotifyPropertyChanged();
|
|
}
|
|
}
|
|
}
|
|
|
|
public bool PerColorChannelEdgeDetection
|
|
{
|
|
get
|
|
{
|
|
return Settings.Properties.PerColorChannelEdgeDetection;
|
|
}
|
|
|
|
set
|
|
{
|
|
if (Settings.Properties.PerColorChannelEdgeDetection != value)
|
|
{
|
|
Settings.Properties.PerColorChannelEdgeDetection = value;
|
|
NotifyPropertyChanged();
|
|
}
|
|
}
|
|
}
|
|
|
|
public int UnitsOfMeasure
|
|
{
|
|
get
|
|
{
|
|
return Settings.Properties.UnitsOfMeasure.Value;
|
|
}
|
|
|
|
set
|
|
{
|
|
if (Settings.Properties.UnitsOfMeasure.Value != value)
|
|
{
|
|
Settings.Properties.UnitsOfMeasure.Value = value;
|
|
NotifyPropertyChanged();
|
|
}
|
|
}
|
|
}
|
|
|
|
public int PixelTolerance
|
|
{
|
|
get
|
|
{
|
|
return Settings.Properties.PixelTolerance.Value;
|
|
}
|
|
|
|
set
|
|
{
|
|
if (Settings.Properties.PixelTolerance.Value != value)
|
|
{
|
|
Settings.Properties.PixelTolerance.Value = value;
|
|
NotifyPropertyChanged();
|
|
}
|
|
}
|
|
}
|
|
|
|
public HotkeySettings ActivationShortcut
|
|
{
|
|
get
|
|
{
|
|
return Settings.Properties.ActivationShortcut;
|
|
}
|
|
|
|
set
|
|
{
|
|
if (Settings.Properties.ActivationShortcut != value)
|
|
{
|
|
Settings.Properties.ActivationShortcut = value;
|
|
|
|
NotifyPropertyChanged();
|
|
|
|
SendConfigMSG(
|
|
string.Format(
|
|
CultureInfo.InvariantCulture,
|
|
"{{ \"powertoys\": {{ \"{0}\": {1} }} }}",
|
|
MeasureToolSettings.ModuleName,
|
|
JsonSerializer.Serialize(Settings)));
|
|
}
|
|
}
|
|
}
|
|
|
|
public void NotifyPropertyChanged([CallerMemberName] string propertyName = null)
|
|
{
|
|
OnPropertyChanged(propertyName);
|
|
if (propertyName == nameof(ShowContinuousCaptureWarning))
|
|
{
|
|
// Don't trigger a settings update if the changed property is for visual notification.
|
|
return;
|
|
}
|
|
|
|
SettingsUtils.SaveSettings(Settings.ToJsonString(), MeasureToolSettings.ModuleName);
|
|
}
|
|
|
|
public bool ShowContinuousCaptureWarning
|
|
{
|
|
get => IsEnabled && ContinuousCapture;
|
|
}
|
|
|
|
private Func<string, int> SendConfigMSG { get; }
|
|
}
|
|
}
|