mirror of
https://github.com/microsoft/PowerToys.git
synced 2024-12-21 15:27:55 +08:00
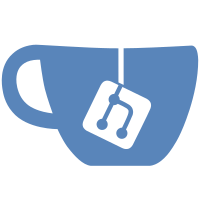
* Screen reader detecting List view * Fixed narrator text for listview items and context menu items * Renamed custom textbox to a more meanigful name * Renamed custom textbox to a more meanigful name * Fix formatting of LauncherControl.xaml * Added support to control multiple elements
43 lines
1.3 KiB
C#
43 lines
1.3 KiB
C#
// Copyright (c) Microsoft Corporation
|
|
// The Microsoft Corporation licenses this file to you under the MIT license.
|
|
// See the LICENSE file in the project root for more information.
|
|
|
|
using System;
|
|
using System.Collections.Generic;
|
|
using System.Text;
|
|
using System.Windows;
|
|
using System.Windows.Automation.Peers;
|
|
using System.Windows.Controls;
|
|
|
|
namespace PowerLauncher
|
|
{
|
|
public class CustomSearchBox : TextBox
|
|
{
|
|
public List<UIElement> ControlledElements { get; } = new List<UIElement>();
|
|
|
|
protected override AutomationPeer OnCreateAutomationPeer()
|
|
{
|
|
return new AutoSuggestTextBoxAutomationPeer(this);
|
|
}
|
|
|
|
internal class AutoSuggestTextBoxAutomationPeer : TextBoxAutomationPeer
|
|
{
|
|
public AutoSuggestTextBoxAutomationPeer(CustomSearchBox owner)
|
|
: base(owner)
|
|
{
|
|
}
|
|
|
|
protected override List<AutomationPeer> GetControlledPeersCore()
|
|
{
|
|
var controlledPeers = new List<AutomationPeer>();
|
|
foreach (UIElement controlledElement in ((CustomSearchBox)Owner).ControlledElements)
|
|
{
|
|
controlledPeers.Add(UIElementAutomationPeer.CreatePeerForElement(controlledElement));
|
|
}
|
|
|
|
return controlledPeers;
|
|
}
|
|
}
|
|
}
|
|
}
|