mirror of
https://github.com/microsoft/PowerToys.git
synced 2025-01-11 22:57:59 +08:00
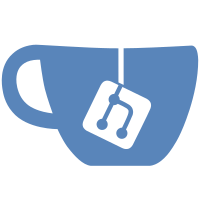
* C++ impl of immersive dark mode * Stop using the hardcoded value. * Conjured up theme listener based on registry. * Update MainWindow.xaml.cpp * Update expect.txt * Moved themehelpers to the common themes lib. * Ported theme helpers back to .NET * Update expect.txt * Updated C# Theme Listening logic to mimic the one from Windows Community Toolkit. * Replaced unmanaged code for RegisterForImmersiveDarkMode with unmanaged ThemeListener class. * Fix upstream changes * Update ThemeListener.h * Update ThemeListener.h * Proper formatting * Added handler to Keyboard Manager. * Update EditKeyboardWindow.cpp * Added dwmapi.lib to runner, removed condition from additional dependencies. * Update PowerRenameUI.vcxproj * Added new deps for ManagedCommon to Product.wxs * Crude attempts and understanding installer * Removed Microsoft.Win32.Registry.dll from product.wxs. * Updated dictionary * Renamed ThemeListener class file for consistency, removed unused CheckImmersiveDarkMode in theme_helpers. * Update Themes.vcxproj * Update theme_listener.cpp * Removed SupportsImmersiveDarkMode version check * Removed SupportsImmersiveDarkMode version check * Whoops * Update expect.txt
122 lines
4.0 KiB
C#
122 lines
4.0 KiB
C#
// Copyright (c) Microsoft Corporation
|
|
// The Microsoft Corporation licenses this file to you under the MIT license.
|
|
// See the LICENSE file in the project root for more information.
|
|
|
|
using System;
|
|
using interop;
|
|
using ManagedCommon;
|
|
using Microsoft.PowerToys.Settings.UI.Helpers;
|
|
using Microsoft.PowerToys.Settings.UI.OOBE.Enums;
|
|
using Microsoft.PowerToys.Settings.UI.OOBE.Views;
|
|
using Microsoft.UI;
|
|
using Microsoft.UI.Windowing;
|
|
using Microsoft.UI.Xaml;
|
|
using Windows.ApplicationModel.Resources;
|
|
using Windows.Graphics;
|
|
|
|
namespace Microsoft.PowerToys.Settings.UI
|
|
{
|
|
/// <summary>
|
|
/// An empty window that can be used on its own or navigated to within a Frame.
|
|
/// </summary>
|
|
public sealed partial class OobeWindow : Window
|
|
{
|
|
private PowerToysModules initialModule;
|
|
|
|
private const int ExpectedWidth = 1100;
|
|
private const int ExpectedHeight = 700;
|
|
private const int DefaultDPI = 96;
|
|
private int _currentDPI;
|
|
private WindowId _windowId;
|
|
private IntPtr _hWnd;
|
|
private AppWindow _appWindow;
|
|
|
|
public OobeWindow(PowerToysModules initialModule, bool isDark)
|
|
{
|
|
this.InitializeComponent();
|
|
|
|
// Set window icon
|
|
_hWnd = WinRT.Interop.WindowNative.GetWindowHandle(this);
|
|
_windowId = Win32Interop.GetWindowIdFromWindow(_hWnd);
|
|
_appWindow = AppWindow.GetFromWindowId(_windowId);
|
|
_appWindow.SetIcon("icon.ico");
|
|
|
|
// Passed by parameter, as it needs to be evaluated ASAP, otherwise there is a white flash
|
|
if (isDark)
|
|
{
|
|
ThemeHelpers.SetImmersiveDarkMode(_hWnd, isDark);
|
|
}
|
|
|
|
OverlappedPresenter presenter = _appWindow.Presenter as OverlappedPresenter;
|
|
presenter.IsMinimizable = false;
|
|
presenter.IsMaximizable = false;
|
|
|
|
var dpi = NativeMethods.GetDpiForWindow(_hWnd);
|
|
_currentDPI = dpi;
|
|
float scalingFactor = (float)dpi / DefaultDPI;
|
|
int width = (int)(ExpectedWidth * scalingFactor);
|
|
int height = (int)(ExpectedHeight * scalingFactor);
|
|
|
|
SizeInt32 size;
|
|
size.Width = width;
|
|
size.Height = height;
|
|
_appWindow.Resize(size);
|
|
|
|
this.initialModule = initialModule;
|
|
|
|
this.SizeChanged += OobeWindow_SizeChanged;
|
|
|
|
ResourceLoader loader = ResourceLoader.GetForViewIndependentUse();
|
|
Title = loader.GetString("OobeWindow_Title");
|
|
|
|
if (shellPage != null)
|
|
{
|
|
shellPage.NavigateToModule(this.initialModule);
|
|
}
|
|
|
|
OobeShellPage.SetRunSharedEventCallback(() =>
|
|
{
|
|
return Constants.PowerLauncherSharedEvent();
|
|
});
|
|
|
|
OobeShellPage.SetColorPickerSharedEventCallback(() =>
|
|
{
|
|
return Constants.ShowColorPickerSharedEvent();
|
|
});
|
|
|
|
OobeShellPage.SetOpenMainWindowCallback((Type type) =>
|
|
{
|
|
App.OpenSettingsWindow(type);
|
|
});
|
|
}
|
|
|
|
private void OobeWindow_SizeChanged(object sender, WindowSizeChangedEventArgs args)
|
|
{
|
|
var dpi = NativeMethods.GetDpiForWindow(_hWnd);
|
|
if (_currentDPI != dpi)
|
|
{
|
|
// Reacting to a DPI change. Should not cause a resize -> sizeChanged loop.
|
|
_currentDPI = dpi;
|
|
float scalingFactor = (float)dpi / DefaultDPI;
|
|
int width = (int)(ExpectedWidth * scalingFactor);
|
|
int height = (int)(ExpectedHeight * scalingFactor);
|
|
SizeInt32 size;
|
|
size.Width = width;
|
|
size.Height = height;
|
|
_appWindow.Resize(size);
|
|
}
|
|
}
|
|
|
|
private void Window_Closed(object sender, WindowEventArgs args)
|
|
{
|
|
App.ClearOobeWindow();
|
|
|
|
var mainWindow = App.GetSettingsWindow();
|
|
if (mainWindow != null)
|
|
{
|
|
mainWindow.CloseHiddenWindow();
|
|
}
|
|
}
|
|
}
|
|
}
|