mirror of
https://github.com/microsoft/PowerToys.git
synced 2025-01-11 22:57:59 +08:00
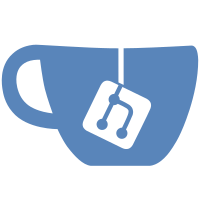
* rename dll -> FancyZonesModuleInterface (#11488) * [FancyZones] Rename "fancyzones/tests" -> "fancyzones/FancyZonesTests" (#11492) * [FancyZones] Rename "fancyzones/lib" -> "fancyzones/FancyZonesLib" (#11489) * [FancyZones] New FancyZones project. (#11544) * [FancyZones] Allow single instance of "PowerToys.FancyZones.exe" (#11558) * [FancyZones] Updated bug reports (#11571) * [FancyZones] Updated installer (#11572) * [FancyZones] Update string resources (#11596) * [FancyZones] Terminate FancyZones with runner (#11696) * [FancyZones] Drop support for the module interface API to save settings (#11661) * Settings telemetry for FancyZones (#11766) * commented out test * enable dpi awareness for the process
69 lines
1.6 KiB
C++
69 lines
1.6 KiB
C++
#include "pch.h"
|
|
#include "FileWatcher.h"
|
|
|
|
std::optional<FILETIME> FileWatcher::MyFileTime()
|
|
{
|
|
HANDLE hFile = CreateFileW(m_path.c_str(), FILE_READ_ATTRIBUTES, FILE_SHARE_DELETE | FILE_SHARE_READ | FILE_SHARE_WRITE, nullptr, OPEN_EXISTING, 0, nullptr);
|
|
std::optional<FILETIME> result;
|
|
if (hFile != INVALID_HANDLE_VALUE)
|
|
{
|
|
FILETIME lastWrite;
|
|
if (GetFileTime(hFile, nullptr, nullptr, &lastWrite))
|
|
{
|
|
result = lastWrite;
|
|
}
|
|
|
|
CloseHandle(hFile);
|
|
}
|
|
|
|
return result;
|
|
}
|
|
|
|
void FileWatcher::Run()
|
|
{
|
|
while (1)
|
|
{
|
|
auto lastWrite = MyFileTime();
|
|
if (!m_lastWrite.has_value())
|
|
{
|
|
m_lastWrite = lastWrite;
|
|
}
|
|
else if (lastWrite.has_value())
|
|
{
|
|
if (m_lastWrite->dwHighDateTime != lastWrite->dwHighDateTime ||
|
|
m_lastWrite->dwLowDateTime != lastWrite->dwLowDateTime)
|
|
{
|
|
m_lastWrite = lastWrite;
|
|
m_callback();
|
|
}
|
|
}
|
|
|
|
if (WaitForSingleObject(m_abortEvent, m_refreshPeriod) == WAIT_OBJECT_0)
|
|
{
|
|
return;
|
|
}
|
|
}
|
|
}
|
|
|
|
FileWatcher::FileWatcher(const std::wstring& path, std::function<void()> callback, DWORD refreshPeriod) :
|
|
m_refreshPeriod(refreshPeriod),
|
|
m_path(path),
|
|
m_callback(callback)
|
|
{
|
|
m_abortEvent = CreateEventW(nullptr, TRUE, FALSE, nullptr);
|
|
if (m_abortEvent)
|
|
{
|
|
m_thread = std::thread([this]() { Run(); });
|
|
}
|
|
}
|
|
|
|
FileWatcher::~FileWatcher()
|
|
{
|
|
if (m_abortEvent)
|
|
{
|
|
SetEvent(m_abortEvent);
|
|
m_thread.join();
|
|
CloseHandle(m_abortEvent);
|
|
}
|
|
}
|