mirror of
https://github.com/microsoft/PowerToys.git
synced 2024-12-15 12:09:18 +08:00
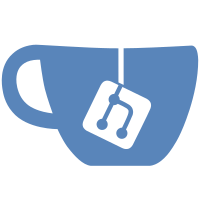
* Added a keyboard hook to the runner * Update RootKeyboardHook * Enable reading the whole JsonObject property * Renamed RootKeyboardHook to CentralizedKeyboardHook * Fixed build break, changed callback return type to bool * Added Hotkey struct which somehow went missing + Cherry-pick fixes * Reorganized the kb hook * Basic version works * Various fixes * Finishing touches * Fix potential threading issue * int -> size_t * Add default initializers to the Hotkey struct * Added a suggested comment * Unified a constant * Use C# classes instead of native calls for sync * Added a claryfing comment * Use std::move * Renamed a method * Possible fix for compilation errors * Fix a regression * Show a message on failure * Added DISABLE_LOWLEVEL_HOOK support * Allow running Launcher as standalone * Rename string constants
52 lines
1.0 KiB
C++
52 lines
1.0 KiB
C++
#pragma once
|
|
#include <interface/powertoy_module_interface.h>
|
|
#include <string>
|
|
#include <memory>
|
|
#include <mutex>
|
|
#include <vector>
|
|
#include <functional>
|
|
|
|
#include <common/json.h>
|
|
|
|
struct PowertoyModuleDeleter
|
|
{
|
|
void operator()(PowertoyModuleIface* module) const
|
|
{
|
|
if (module)
|
|
{
|
|
module->destroy();
|
|
}
|
|
}
|
|
};
|
|
|
|
struct PowertoyModuleDLLDeleter
|
|
{
|
|
using pointer = HMODULE;
|
|
void operator()(HMODULE handle) const
|
|
{
|
|
FreeLibrary(handle);
|
|
}
|
|
};
|
|
|
|
class PowertoyModule
|
|
{
|
|
public:
|
|
PowertoyModule(PowertoyModuleIface* module, HMODULE handle);
|
|
|
|
inline PowertoyModuleIface* operator->()
|
|
{
|
|
return module.get();
|
|
}
|
|
|
|
json::JsonObject json_config() const;
|
|
|
|
void update_hotkeys();
|
|
|
|
private:
|
|
std::unique_ptr<HMODULE, PowertoyModuleDLLDeleter> handle;
|
|
std::unique_ptr<PowertoyModuleIface, PowertoyModuleDeleter> module;
|
|
};
|
|
|
|
PowertoyModule load_powertoy(const std::wstring_view filename);
|
|
std::map<std::wstring, PowertoyModule>& modules();
|