mirror of
https://github.com/microsoft/PowerToys.git
synced 2024-12-29 21:17:54 +08:00
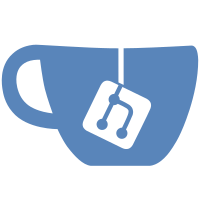
- remove common lib - split settings, remove common-md - move ipc interop/kb_layout to interop - rename core -> settings, settings -> old_settings - os-detect header-only; interop -> PowerToysInterop - split notifications, move single-use headers where they're used - winstore lib - rename com utils - rename Updating and Telemetry projects - rename core -> settings-ui and remove examples folder - rename settings-ui folder + consisent common/version include
64 lines
2.3 KiB
C#
64 lines
2.3 KiB
C#
// Copyright (c) Microsoft Corporation
|
|
// The Microsoft Corporation licenses this file to you under the MIT license.
|
|
// See the LICENSE file in the project root for more information.
|
|
|
|
using System;
|
|
using System.Globalization;
|
|
using Microsoft.PowerToys.Settings.UI.Library;
|
|
using Windows.UI.Xaml;
|
|
using Windows.UI.Xaml.Data;
|
|
using Windows.UI.Xaml.Media;
|
|
|
|
namespace Microsoft.PowerToys.Settings.UI.Converters
|
|
{
|
|
public sealed class ModuleEnabledToForegroundConverter : IValueConverter
|
|
{
|
|
private readonly ISettingsUtils settingsUtils = new SettingsUtils();
|
|
|
|
private string selectedTheme = string.Empty;
|
|
|
|
public object Convert(object value, Type targetType, object parameter, string language)
|
|
{
|
|
bool isEnabled = (bool)value;
|
|
|
|
var defaultTheme = new Windows.UI.ViewManagement.UISettings();
|
|
|
|
// Using InvariantCulture as this is an internal string and expected to be in hexadecimal
|
|
var uiTheme = defaultTheme.GetColorValue(Windows.UI.ViewManagement.UIColorType.Background).ToString(CultureInfo.InvariantCulture);
|
|
|
|
// Normalize strings to uppercase according to Fxcop
|
|
selectedTheme = SettingsRepository<GeneralSettings>.GetInstance(settingsUtils).SettingsConfig.Theme.ToUpperInvariant();
|
|
|
|
if (selectedTheme == "DARK" || (selectedTheme == "SYSTEM" && uiTheme == "#FF000000"))
|
|
{
|
|
// DARK
|
|
if (isEnabled)
|
|
{
|
|
return (SolidColorBrush)Application.Current.Resources["DarkForegroundBrush"];
|
|
}
|
|
else
|
|
{
|
|
return (SolidColorBrush)Application.Current.Resources["DarkForegroundDisabledBrush"];
|
|
}
|
|
}
|
|
else
|
|
{
|
|
// LIGHT
|
|
if (isEnabled)
|
|
{
|
|
return (SolidColorBrush)Application.Current.Resources["LightForegroundBrush"];
|
|
}
|
|
else
|
|
{
|
|
return (SolidColorBrush)Application.Current.Resources["LightForegroundDisabledBrush"];
|
|
}
|
|
}
|
|
}
|
|
|
|
public object ConvertBack(object value, Type targetType, object parameter, string language)
|
|
{
|
|
return value;
|
|
}
|
|
}
|
|
}
|