mirror of
https://github.com/ant-design/ant-design.git
synced 2024-12-15 17:19:11 +08:00
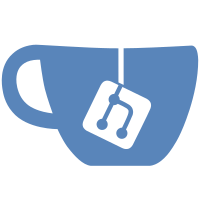
* feat: ConfigProvider support variant * docs: add docs for variant * feat: support config all components variant * chore: fix lint * chore: add test * test: add test
48 lines
1.3 KiB
TypeScript
48 lines
1.3 KiB
TypeScript
import { useContext } from 'react';
|
|
|
|
import { VariantContext } from '../context';
|
|
import type { Variant, ConfigProviderProps } from '../../config-provider';
|
|
import { ConfigContext, Variants } from '../../config-provider';
|
|
|
|
type VariantComponents = keyof Pick<
|
|
ConfigProviderProps,
|
|
| 'input'
|
|
| 'inputNumber'
|
|
| 'textArea'
|
|
| 'mentions'
|
|
| 'select'
|
|
| 'cascader'
|
|
| 'treeSelect'
|
|
| 'datePicker'
|
|
| 'timePicker'
|
|
| 'rangePicker'
|
|
>;
|
|
|
|
/**
|
|
* Compatible for legacy `bordered` prop.
|
|
*/
|
|
const useVariant = (
|
|
component: VariantComponents,
|
|
variant: Variant | undefined,
|
|
legacyBordered: boolean | undefined = undefined,
|
|
): [Variant, boolean] => {
|
|
const { variant: configVariant, [component]: componentConfig } = useContext(ConfigContext);
|
|
const ctxVariant = useContext(VariantContext);
|
|
const configComponentVariant = componentConfig?.variant;
|
|
|
|
let mergedVariant: Variant;
|
|
if (typeof variant !== 'undefined') {
|
|
mergedVariant = variant;
|
|
} else if (legacyBordered === false) {
|
|
mergedVariant = 'borderless';
|
|
} else {
|
|
// form variant > component global variant > global variant
|
|
mergedVariant = ctxVariant ?? configComponentVariant ?? configVariant ?? 'outlined';
|
|
}
|
|
|
|
const enableVariantCls = Variants.includes(mergedVariant);
|
|
return [mergedVariant, enableVariantCls];
|
|
};
|
|
|
|
export default useVariant;
|