mirror of
https://github.com/ant-design/ant-design.git
synced 2024-11-24 19:19:57 +08:00
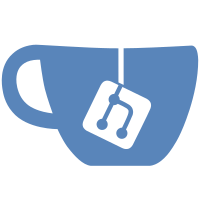
* use flex * show clear btn * support one way * add dropdown * add pagination * support pagination * use flex * operation stretch * pagination works * update selection logic * no need to show checkbox on pagination * fix tree demo * support invert selection * remove current pages * update style * update snapshot * clean up * update test case * update test case * update snapshot * fix lint * add deps * update doc * update hover style * update hover checked style * adjust demo & active region * fix lint * update snapshot
85 lines
2.4 KiB
TypeScript
85 lines
2.4 KiB
TypeScript
import * as React from 'react';
|
|
import classNames from 'classnames';
|
|
import { DeleteOutlined } from '@ant-design/icons';
|
|
import { TransferItem, TransferLocale } from '.';
|
|
import defaultLocale from '../locale/default';
|
|
import Checkbox from '../checkbox';
|
|
import TransButton from '../_util/transButton';
|
|
import LocaleReceiver from '../locale-provider/LocaleReceiver';
|
|
|
|
type ListItemProps = {
|
|
renderedText?: string | number;
|
|
renderedEl: React.ReactNode;
|
|
disabled?: boolean;
|
|
checked?: boolean;
|
|
prefixCls: string;
|
|
onClick: (item: TransferItem) => void;
|
|
onRemove?: (item: TransferItem) => void;
|
|
item: TransferItem;
|
|
showRemove?: boolean;
|
|
};
|
|
|
|
const ListItem = (props: ListItemProps) => {
|
|
const {
|
|
renderedText,
|
|
renderedEl,
|
|
item,
|
|
checked,
|
|
disabled,
|
|
prefixCls,
|
|
onClick,
|
|
onRemove,
|
|
showRemove,
|
|
} = props;
|
|
|
|
const className = classNames({
|
|
[`${prefixCls}-content-item`]: true,
|
|
[`${prefixCls}-content-item-disabled`]: disabled || item.disabled,
|
|
[`${prefixCls}-content-item-checked`]: checked,
|
|
});
|
|
|
|
let title: string | undefined;
|
|
if (typeof renderedText === 'string' || typeof renderedText === 'number') {
|
|
title = String(renderedText);
|
|
}
|
|
|
|
return (
|
|
<LocaleReceiver componentName="Transfer" defaultLocale={defaultLocale.Transfer}>
|
|
{(transferLocale: TransferLocale) => {
|
|
const liProps: React.HTMLAttributes<HTMLLIElement> = { className, title };
|
|
const labelNode = <span className={`${prefixCls}-content-item-text`}>{renderedEl}</span>;
|
|
|
|
// Show remove
|
|
if (showRemove) {
|
|
return (
|
|
<li {...liProps}>
|
|
{labelNode}
|
|
<TransButton
|
|
disabled={disabled || item.disabled}
|
|
className={`${prefixCls}-content-item-remove`}
|
|
aria-label={transferLocale.remove}
|
|
onClick={() => {
|
|
onRemove?.(item);
|
|
}}
|
|
>
|
|
<DeleteOutlined />
|
|
</TransButton>
|
|
</li>
|
|
);
|
|
}
|
|
|
|
// Default click to select
|
|
liProps.onClick = disabled || item.disabled ? undefined : () => onClick(item);
|
|
return (
|
|
<li {...liProps}>
|
|
<Checkbox checked={checked} disabled={disabled || item.disabled} />
|
|
{labelNode}
|
|
</li>
|
|
);
|
|
}}
|
|
</LocaleReceiver>
|
|
);
|
|
};
|
|
|
|
export default React.memo(ListItem);
|