mirror of
https://github.com/ant-design/ant-design.git
synced 2024-11-24 11:10:01 +08:00
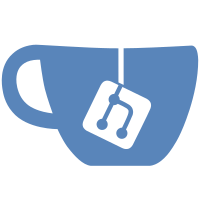
* #18237 add skeleton button * update snapshot * refactoring demo code * lint code * update test snapshots * add test * update snapshot * fix deps lint issue * set avatar siz and update test snapshots * fix lint issue * remove button and just keep skeleton * add active switch for button demo * add size and shape radio for skeleton button demo * add button tests * add skeleton tests * update doc * update test snapshots * omit avatar and button props * refactoring skeleton and update test snapshots
38 lines
1.2 KiB
TypeScript
38 lines
1.2 KiB
TypeScript
import * as React from 'react';
|
|
import omit from 'omit.js';
|
|
import classNames from 'classnames';
|
|
import SkeletonElement, { SkeletonElementProps } from './SkeletonElement';
|
|
import { ConfigConsumer, ConfigConsumerProps } from '../config-provider';
|
|
|
|
interface SkeletonButtonProps extends Omit<SkeletonElementProps, 'size'> {
|
|
active?: boolean;
|
|
size?: 'large' | 'small' | 'default';
|
|
}
|
|
|
|
// eslint-disable-next-line react/prefer-stateless-function
|
|
class SkeletonButton extends React.Component<SkeletonButtonProps, any> {
|
|
static defaultProps: Partial<SkeletonButtonProps> = {
|
|
size: 'default',
|
|
};
|
|
|
|
renderSkeletonButton = ({ getPrefixCls }: ConfigConsumerProps) => {
|
|
const { prefixCls: customizePrefixCls, className, active } = this.props;
|
|
const prefixCls = getPrefixCls('skeleton', customizePrefixCls);
|
|
const otherProps = omit(this.props, ['prefixCls']);
|
|
const cls = classNames(prefixCls, className, `${prefixCls}-element`, {
|
|
[`${prefixCls}-active`]: active,
|
|
});
|
|
return (
|
|
<div className={cls}>
|
|
<SkeletonElement prefixCls={`${prefixCls}-button`} {...otherProps} />
|
|
</div>
|
|
);
|
|
};
|
|
|
|
render() {
|
|
return <ConfigConsumer>{this.renderSkeletonButton}</ConfigConsumer>;
|
|
}
|
|
}
|
|
|
|
export default SkeletonButton;
|