mirror of
https://github.com/ant-design/ant-design.git
synced 2024-11-25 19:50:05 +08:00
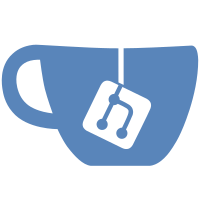
* feat: Table supports filterMode="tree-select" * add tree component * fix detail * use tree * add @table-filter-dropdown-max-height * feat: add check all to filter tree * feat: add search * feat: use filterTreeNode * fix code style * fix style * style: tree node selected bg * fix demo * feat: add filterSearch * fix: clear search value after close filter dropdown * update snapshot * code style * fix test case * chore: new FilterDropdown.tsx file * chore: searchValueMatched function * add test case * test: add test cases * feat: reset only works on dropdown state now * chore: add table locales * fix search input width * tweak style * style: update transfer search input style * perf: improve table perf * fix: filterMuiltiple={false} * test: add test for selecting * chore: fix table filter selection * fix lint * remove unused code * fix: style dependencies * test: turn off bail config for duplidated-package-plugin in feature branch * Update components/table/hooks/useFilter/FilterSearch.tsx Co-authored-by: Peach <scdzwyxst@gmail.com> * fix locale link * Apply suggestions from code review * Apply suggestions from code review Co-authored-by: Peach <scdzwyxst@gmail.com>
40 lines
923 B
TypeScript
40 lines
923 B
TypeScript
import * as React from 'react';
|
|
import SearchOutlined from '@ant-design/icons/SearchOutlined';
|
|
|
|
import Input from '../input';
|
|
|
|
export interface TransferSearchProps {
|
|
prefixCls?: string;
|
|
placeholder?: string;
|
|
onChange?: (e: React.FormEvent<HTMLElement>) => void;
|
|
handleClear?: () => void;
|
|
value?: string;
|
|
disabled?: boolean;
|
|
}
|
|
|
|
export default function Search(props: TransferSearchProps) {
|
|
const { placeholder = '', value, prefixCls, disabled, onChange, handleClear } = props;
|
|
|
|
const handleChange = React.useCallback(
|
|
(e: React.ChangeEvent<HTMLInputElement>) => {
|
|
onChange?.(e);
|
|
if (e.target.value === '') {
|
|
handleClear?.();
|
|
}
|
|
},
|
|
[onChange],
|
|
);
|
|
|
|
return (
|
|
<Input
|
|
placeholder={placeholder}
|
|
className={prefixCls}
|
|
value={value}
|
|
onChange={handleChange}
|
|
disabled={disabled}
|
|
allowClear
|
|
prefix={<SearchOutlined />}
|
|
/>
|
|
);
|
|
}
|