mirror of
https://github.com/ant-design/ant-design.git
synced 2024-12-04 17:09:46 +08:00
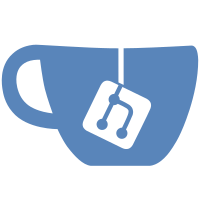
* eslint disallow use of chained assignment expressions eslint disallow use of chained assignment expressions * revert * message-doc-update 1.If a variable is never reassigned, using the const declaration is better. 2.Defaultvalue’s type must be ‘number’ depend on type
141 lines
3.8 KiB
TypeScript
Executable File
141 lines
3.8 KiB
TypeScript
Executable File
import * as React from 'react';
|
|
import Notification from 'rc-notification';
|
|
import Icon from '../icon';
|
|
|
|
let defaultDuration = 3;
|
|
let defaultTop: number;
|
|
let messageInstance: any;
|
|
let key = 1;
|
|
let prefixCls = 'ant-message';
|
|
let transitionName = 'move-up';
|
|
let getContainer: () => HTMLElement;
|
|
let maxCount: number;
|
|
|
|
function getMessageInstance(callback: (i: any) => void) {
|
|
if (messageInstance) {
|
|
callback(messageInstance);
|
|
return;
|
|
}
|
|
Notification.newInstance({
|
|
prefixCls,
|
|
transitionName,
|
|
style: { top: defaultTop }, // 覆盖原来的样式
|
|
getContainer,
|
|
maxCount,
|
|
}, (instance: any) => {
|
|
if (messageInstance) {
|
|
callback(messageInstance);
|
|
return;
|
|
}
|
|
messageInstance = instance;
|
|
callback(instance);
|
|
});
|
|
}
|
|
|
|
type NoticeType = 'info' | 'success' | 'error' | 'warning' | 'loading';
|
|
|
|
function notice(
|
|
content: React.ReactNode,
|
|
duration: (() => void) | number = defaultDuration,
|
|
type: NoticeType,
|
|
onClose?: () => void,
|
|
) {
|
|
const iconType = ({
|
|
info: 'info-circle',
|
|
success: 'check-circle',
|
|
error: 'cross-circle',
|
|
warning: 'exclamation-circle',
|
|
loading: 'loading',
|
|
})[type];
|
|
|
|
if (typeof duration === 'function') {
|
|
onClose = duration;
|
|
duration = defaultDuration;
|
|
}
|
|
|
|
const target = key++;
|
|
getMessageInstance((instance) => {
|
|
instance.notice({
|
|
key: target,
|
|
duration,
|
|
style: {},
|
|
content: (
|
|
<div className={`${prefixCls}-custom-content ${prefixCls}-${type}`}>
|
|
<Icon type={iconType} />
|
|
<span>{content}</span>
|
|
</div>
|
|
),
|
|
onClose,
|
|
});
|
|
});
|
|
return () => {
|
|
if (messageInstance) {
|
|
messageInstance.removeNotice(target);
|
|
}
|
|
};
|
|
}
|
|
|
|
type ConfigContent = React.ReactNode | string;
|
|
type ConfigDuration = number | (() => void);
|
|
export type ConfigOnClose = () => void;
|
|
|
|
export interface ConfigOptions {
|
|
top?: number;
|
|
duration?: number;
|
|
prefixCls?: string;
|
|
getContainer?: () => HTMLElement;
|
|
transitionName?: string;
|
|
maxCount?: number;
|
|
}
|
|
|
|
export default {
|
|
info(content: ConfigContent, duration?: ConfigDuration, onClose?: ConfigOnClose) {
|
|
return notice(content, duration, 'info', onClose);
|
|
},
|
|
success(content: ConfigContent, duration?: ConfigDuration, onClose?: ConfigOnClose) {
|
|
return notice(content, duration, 'success', onClose);
|
|
},
|
|
error(content: ConfigContent, duration?: ConfigDuration, onClose?: ConfigOnClose) {
|
|
return notice(content, duration, 'error', onClose);
|
|
},
|
|
// Departed usage, please use warning()
|
|
warn(content: ConfigContent, duration?: ConfigDuration, onClose?: ConfigOnClose) {
|
|
return notice(content, duration, 'warning', onClose);
|
|
},
|
|
warning(content: ConfigContent, duration?: ConfigDuration, onClose?: ConfigOnClose) {
|
|
return notice(content, duration, 'warning', onClose);
|
|
},
|
|
loading(content: ConfigContent, duration?: ConfigDuration, onClose?: ConfigOnClose) {
|
|
return notice(content, duration, 'loading', onClose);
|
|
},
|
|
config(options: ConfigOptions) {
|
|
if (options.top !== undefined) {
|
|
defaultTop = options.top;
|
|
messageInstance = null; // delete messageInstance for new defaultTop
|
|
}
|
|
if (options.duration !== undefined) {
|
|
defaultDuration = options.duration;
|
|
}
|
|
if (options.prefixCls !== undefined) {
|
|
prefixCls = options.prefixCls;
|
|
}
|
|
if (options.getContainer !== undefined) {
|
|
getContainer = options.getContainer;
|
|
}
|
|
if (options.transitionName !== undefined) {
|
|
transitionName = options.transitionName;
|
|
messageInstance = null; // delete messageInstance for new transitionName
|
|
}
|
|
if (options.maxCount !== undefined) {
|
|
maxCount = options.maxCount;
|
|
messageInstance = null;
|
|
}
|
|
},
|
|
destroy() {
|
|
if (messageInstance) {
|
|
messageInstance.destroy();
|
|
messageInstance = null;
|
|
}
|
|
},
|
|
};
|