mirror of
https://github.com/ant-design/ant-design.git
synced 2024-11-24 11:10:01 +08:00
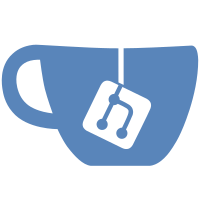
* fix * refactor[Wave]: CC => FC * fix lint * fix * fix * fix * add test case * add test case * fix test * fix test * test case * add test case * fix * fix * fix * fix * raname * fix * test case * test case * test case * fix test * test case * refactor: Use React way * test: coverage * chore: clean up * rerun fail ci * fix: React 17 error * test: fix test case * test: fix test case * fix borderRadius * test: fix test case * chore: clean up * chore: clean up Co-authored-by: 二货机器人 <smith3816@gmail.com>
106 lines
2.7 KiB
TypeScript
106 lines
2.7 KiB
TypeScript
import * as React from 'react';
|
|
import CSSMotion from 'rc-motion';
|
|
import { render, unmount } from 'rc-util/lib/React/render';
|
|
import classNames from 'classnames';
|
|
import { getTargetWaveColor, getValidateContainer } from './util';
|
|
|
|
export interface WaveEffectProps {
|
|
left: number;
|
|
top: number;
|
|
width: number;
|
|
height: number;
|
|
color: string | null;
|
|
className: string;
|
|
scale: number;
|
|
borderRadius: number[];
|
|
}
|
|
|
|
const WaveEffect: React.FC<WaveEffectProps> = (props) => {
|
|
const { className, left, top, width, height, color, borderRadius, scale } = props;
|
|
const divRef = React.useRef<HTMLDivElement>(null);
|
|
|
|
const waveStyle = {
|
|
left,
|
|
top,
|
|
width,
|
|
height,
|
|
borderRadius: borderRadius.map((radius) => `${radius}px`).join(' '),
|
|
'--wave-scale': scale,
|
|
} as React.CSSProperties & {
|
|
[name: string]: number | string;
|
|
};
|
|
|
|
if (color) {
|
|
waveStyle['--wave-color'] = color;
|
|
}
|
|
|
|
return (
|
|
<CSSMotion
|
|
visible
|
|
motionAppear
|
|
motionName="wave-motion"
|
|
motionDeadline={5000}
|
|
onAppearEnd={(_, event) => {
|
|
if (event.deadline || (event as TransitionEvent).propertyName === 'opacity') {
|
|
const holder = divRef.current?.parentElement!;
|
|
unmount(holder).then(() => {
|
|
holder.parentElement?.removeChild(holder);
|
|
});
|
|
}
|
|
return false;
|
|
}}
|
|
>
|
|
{({ className: motionClassName }) => (
|
|
<div ref={divRef} className={classNames(className, motionClassName)} style={waveStyle} />
|
|
)}
|
|
</CSSMotion>
|
|
);
|
|
};
|
|
|
|
function validateNum(value: number) {
|
|
return Number.isNaN(value) ? 0 : value;
|
|
}
|
|
|
|
export default function showWaveEffect(container: Node, node: HTMLElement, className: string) {
|
|
const nodeStyle = getComputedStyle(node);
|
|
const nodeRect = node.getBoundingClientRect();
|
|
|
|
// Get wave color from target
|
|
const waveColor = getTargetWaveColor(node);
|
|
|
|
// Get border radius
|
|
const {
|
|
borderTopLeftRadius,
|
|
borderTopRightRadius,
|
|
borderBottomLeftRadius,
|
|
borderBottomRightRadius,
|
|
} = nodeStyle;
|
|
|
|
// Do scale calc
|
|
const { offsetWidth } = node;
|
|
const scale = validateNum(nodeRect.width / offsetWidth);
|
|
|
|
// Create holder
|
|
const holder = document.createElement('div');
|
|
getValidateContainer(container).appendChild(holder);
|
|
|
|
render(
|
|
<WaveEffect
|
|
left={nodeRect.left}
|
|
top={nodeRect.top}
|
|
width={nodeRect.width}
|
|
height={nodeRect.height}
|
|
color={waveColor}
|
|
className={className}
|
|
scale={scale}
|
|
borderRadius={[
|
|
borderTopLeftRadius,
|
|
borderTopRightRadius,
|
|
borderBottomRightRadius,
|
|
borderBottomLeftRadius,
|
|
].map((radius) => validateNum(parseFloat(radius) * scale))}
|
|
/>,
|
|
holder,
|
|
);
|
|
}
|