mirror of
https://github.com/ant-design/ant-design.git
synced 2024-11-25 11:40:04 +08:00
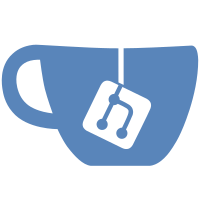
It looks like this was broken in https://github.com/ant-design/ant-design/pull/27790. According to `rc-collapse`, the `collapsible` prop inside Collapse.Panel is optional, so it should be optional here as well if we're just forwarding props directly to the child component.
44 lines
1.3 KiB
TypeScript
44 lines
1.3 KiB
TypeScript
import * as React from 'react';
|
|
import RcCollapse from 'rc-collapse';
|
|
import classNames from 'classnames';
|
|
import { ConfigContext } from '../config-provider';
|
|
import devWarning from '../_util/devWarning';
|
|
|
|
export type CollapsibleType = 'header' | 'disabled';
|
|
|
|
export interface CollapsePanelProps {
|
|
key: string | number;
|
|
header: React.ReactNode;
|
|
/** @deprecated Use `collapsible="disabled"` instead */
|
|
disabled?: boolean;
|
|
className?: string;
|
|
style?: React.CSSProperties;
|
|
showArrow?: boolean;
|
|
prefixCls?: string;
|
|
forceRender?: boolean;
|
|
id?: string;
|
|
extra?: React.ReactNode;
|
|
collapsible?: CollapsibleType;
|
|
}
|
|
|
|
const CollapsePanel: React.FC<CollapsePanelProps> = props => {
|
|
devWarning(
|
|
!('disabled' in props),
|
|
'Collapse.Panel',
|
|
'`disabled` is deprecated. Please use `collapsible="disabled"` instead.',
|
|
);
|
|
|
|
const { getPrefixCls } = React.useContext(ConfigContext);
|
|
const { prefixCls: customizePrefixCls, className = '', showArrow = true } = props;
|
|
const prefixCls = getPrefixCls('collapse', customizePrefixCls);
|
|
const collapsePanelClassName = classNames(
|
|
{
|
|
[`${prefixCls}-no-arrow`]: !showArrow,
|
|
},
|
|
className,
|
|
);
|
|
return <RcCollapse.Panel {...props} prefixCls={prefixCls} className={collapsePanelClassName} />;
|
|
};
|
|
|
|
export default CollapsePanel;
|