mirror of
https://github.com/ant-design/ant-design.git
synced 2024-11-25 19:50:05 +08:00
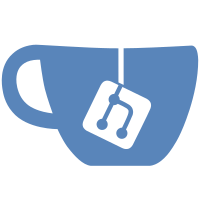
* 🐛 Fix List Item layout style problems * 🐛 horizontal list should align center * ✅ Add test cases * ✅ Add test cases for List.Item * 🐛 Fix vertical list item with extra
100 lines
3.2 KiB
JavaScript
100 lines
3.2 KiB
JavaScript
import React from 'react';
|
|
import { mount } from 'enzyme';
|
|
import List from '..';
|
|
|
|
describe('List Item Layout', () => {
|
|
const data = [{
|
|
key: 1,
|
|
href: 'http://ant.design',
|
|
title: `ant design`,
|
|
avatar: 'https://zos.alipayobjects.com/rmsportal/ODTLcjxAfvqbxHnVXCYX.png',
|
|
description: 'Ant Design, a design language for background applications, is refined by Ant UED Team.',
|
|
content: 'We supply a series of design principles, practical patterns and high quality design resources (Sketch and Axure), to help people create their product prototypes beautifully and efficiently.',
|
|
extra: 'extra',
|
|
}];
|
|
|
|
it('horizontal itemLayout List which contains string nodes should not be flex container', () => {
|
|
const wrapper = mount(
|
|
<List
|
|
dataSource={data}
|
|
renderItem={item => (
|
|
<List.Item key={item.title}>
|
|
I am <span>ant</span> design list item
|
|
</List.Item>
|
|
)}
|
|
/>
|
|
);
|
|
expect(wrapper.find('.ant-list-item').at(0).hasClass('ant-list-item-no-flex')).toBe(true);
|
|
});
|
|
|
|
it('horizontal itemLayout List should be flex container defaultly', () => {
|
|
const wrapper = mount(
|
|
<List
|
|
dataSource={data}
|
|
renderItem={item => (
|
|
<List.Item key={item.title}>
|
|
<List.Item.Meta
|
|
title={<a href={item.href}>{item.title}</a>}
|
|
description={item.description}
|
|
/>
|
|
</List.Item>
|
|
)}
|
|
/>
|
|
);
|
|
expect(wrapper.find('.ant-list-item').at(0).hasClass('ant-list-item-no-flex')).toBe(false);
|
|
});
|
|
|
|
it('vertical itemLayout List should be flex container when there is extra node', () => {
|
|
const wrapper = mount(
|
|
<List
|
|
itemLayout="vertical"
|
|
dataSource={data}
|
|
renderItem={item => (
|
|
<List.Item key={item.title} extra={item.extra}>
|
|
<List.Item.Meta
|
|
title={<a href={item.href}>{item.title}</a>}
|
|
description={item.description}
|
|
/>
|
|
</List.Item>
|
|
)}
|
|
/>
|
|
);
|
|
expect(wrapper.find('.ant-list-item').at(0).hasClass('ant-list-item-no-flex')).toBe(false);
|
|
});
|
|
|
|
it('vertical itemLayout List should not be flex container when there is not extra node', () => {
|
|
const wrapper = mount(
|
|
<List
|
|
itemLayout="vertical"
|
|
dataSource={data}
|
|
renderItem={item => (
|
|
<List.Item key={item.title}>
|
|
<List.Item.Meta
|
|
title={<a href={item.href}>{item.title}</a>}
|
|
description={item.description}
|
|
/>
|
|
</List.Item>
|
|
)}
|
|
/>
|
|
);
|
|
expect(wrapper.find('.ant-list-item').at(0).hasClass('ant-list-item-no-flex')).toBe(true);
|
|
});
|
|
|
|
it('horizontal itemLayout List should accept extra node', () => {
|
|
const wrapper = mount(
|
|
<List
|
|
dataSource={data}
|
|
renderItem={item => (
|
|
<List.Item key={item.title} actions={[<a>Action</a>]} extra={<span>{item.extra}</span>}>
|
|
<List.Item.Meta
|
|
title={<a href={item.href}>{item.title}</a>}
|
|
description={item.description}
|
|
/>
|
|
</List.Item>
|
|
)}
|
|
/>
|
|
);
|
|
expect(wrapper.render()).toMatchSnapshot();
|
|
});
|
|
});
|