mirror of
https://github.com/ant-design/ant-design.git
synced 2024-12-04 17:09:46 +08:00
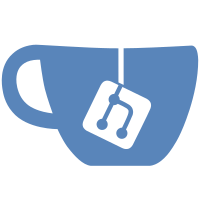
* chore: migrate to vitest * chore: update ci * fix: test correctly * test: support puppeteer * chore: update coverage * chore: update include/exclude * chore: update config * test: update incorrect tests * chore: update script * chore: update * fix: should close browser at the ended * chore: improve * fix: test cause tsc error * fix: eslint error * chore: exclude correctly * test: update snap and fix some tests * chore: update test config * fix: countup.js * fix: incorrect test * chore: update reference * test: update * fix: countup.js * fix: timeout * chore: update site test * fix: fixed countup version * chore: remove unsed code * test: update * test: update demo timeout * test: update timeout * chore: update image test * chore: update threads * fix: image/svg+xml test failed * chore: limits threads * test: update test coverage include * chore: remove jest files * chore: rename jest to vi * chore: update document * chore: fix missing @types/jsdom * chore: update coverage * chore: update snap * fix:watermark test cases are incorrect * feat: update ignore comment * test: fix test case * test: reset body scrollTop * test: clean up * test: use vi * test: update snapshot * test: update snapshot * test: fix dropdown test failed * fix: toHaveStyle cause test fail * test: improve test case * test: fix * fix: color failed, refer to https://github.com/jsdom/jsdom/pull/3560 * test: fix * test: fix * test: fix circular import * test: revert * ci: coverage failed * test: fix c8 ignore comment * chore: incorrect config * chore: fix ignore ci * test: revert svg+xml * test: fix realTimers * feat: rc-trigger should be remove * test: fix some failed test * chore: remove unused deps and configure eslint-plugin-vitest * test: update snap * chore: remove jest * test: fix lint error --------- Co-authored-by: 二货机器人 <smith3816@gmail.com> Co-authored-by: afc163 <afc163@gmail.com>
76 lines
2.0 KiB
TypeScript
76 lines
2.0 KiB
TypeScript
declare const CSSINJS_STATISTIC: any;
|
|
|
|
const enableStatistic =
|
|
process.env.NODE_ENV !== 'production' || typeof CSSINJS_STATISTIC !== 'undefined';
|
|
let recording = true;
|
|
|
|
/**
|
|
* This function will do as `Object.assign` in production. But will use Object.defineProperty:get to
|
|
* pass all value access in development. To support statistic field usage with alias token.
|
|
*/
|
|
export function merge<T extends object>(...objs: Partial<T>[]): T {
|
|
/* c8 ignore next 3 */
|
|
if (!enableStatistic) {
|
|
return Object.assign({}, ...objs);
|
|
}
|
|
|
|
recording = false;
|
|
|
|
const ret = {} as T;
|
|
|
|
objs.forEach((obj) => {
|
|
const keys = Object.keys(obj);
|
|
|
|
keys.forEach((key) => {
|
|
Object.defineProperty(ret, key, {
|
|
configurable: true,
|
|
enumerable: true,
|
|
get: () => (obj as any)[key],
|
|
});
|
|
});
|
|
});
|
|
|
|
recording = true;
|
|
return ret;
|
|
}
|
|
|
|
/** @internal Internal Usage. Not use in your production. */
|
|
export const statistic: Record<
|
|
string,
|
|
{ global: string[]; component: Record<string, string | number> }
|
|
> = {};
|
|
|
|
/** @internal Internal Usage. Not use in your production. */
|
|
// eslint-disable-next-line camelcase
|
|
export const _statistic_build_: typeof statistic = {};
|
|
|
|
/* c8 ignore next */
|
|
function noop() {}
|
|
|
|
/** Statistic token usage case. Should use `merge` function if you do not want spread record. */
|
|
export default function statisticToken<T extends object>(token: T) {
|
|
let tokenKeys: Set<string> | undefined;
|
|
let proxy = token;
|
|
let flush: (componentName: string, componentToken: Record<string, string | number>) => void =
|
|
noop;
|
|
|
|
if (enableStatistic) {
|
|
tokenKeys = new Set<string>();
|
|
|
|
proxy = new Proxy(token, {
|
|
get(obj: any, prop: any) {
|
|
if (recording) {
|
|
tokenKeys!.add(prop);
|
|
}
|
|
return obj[prop];
|
|
},
|
|
});
|
|
|
|
flush = (componentName, componentToken) => {
|
|
statistic[componentName] = { global: Array.from(tokenKeys!), component: componentToken };
|
|
};
|
|
}
|
|
|
|
return { token: proxy, keys: tokenKeys, flush };
|
|
}
|