mirror of
https://github.com/ant-design/ant-design.git
synced 2024-12-11 05:49:10 +08:00
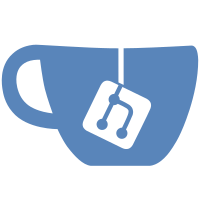
* docs: Add components overview page * fix detail * remove ContributorsList form overview page * fix components url * improve code style * remove extra file * fix detail * fix lint * fix lint * docs: Finish components overview page * fix lint * docs: Update cover * fix lint * update cover * update menu * improve overview page * refactor code * fix order * update title * add components count * fix overview page ssr bug * move less file * update title margin Co-authored-by: arvinxx <arvinx@foxmail.com>
85 lines
3.0 KiB
JavaScript
85 lines
3.0 KiB
JavaScript
import React from 'react';
|
|
import { Helmet } from 'react-helmet-async';
|
|
import { Link } from 'bisheng/router';
|
|
import { useIntl } from 'react-intl';
|
|
import { Divider, Row, Col, Card, Typography, Tag, Space } from 'antd';
|
|
import { getChildren } from 'jsonml.js/lib/utils';
|
|
import { getMetaDescription, getLocalizedPathname, getThemeConfig, getMenuItems } from '../utils';
|
|
import './ComponentOverview.less';
|
|
|
|
const { Title } = Typography;
|
|
const ComponentOverview = ({
|
|
componentsData = [],
|
|
doc: {
|
|
meta: { title },
|
|
content,
|
|
},
|
|
utils: { toReactComponent },
|
|
}) => {
|
|
const { locale } = useIntl();
|
|
const documentTitle = `${title} - Ant Design`;
|
|
const contentChild = getMetaDescription(getChildren(content));
|
|
const themeConfig = getThemeConfig();
|
|
const menuItems = getMenuItems(
|
|
componentsData,
|
|
locale,
|
|
themeConfig.categoryOrder,
|
|
themeConfig.typeOrder,
|
|
);
|
|
|
|
return (
|
|
<section className="markdown">
|
|
<Helmet encodeSpecialCharacters={false}>
|
|
<title>{documentTitle}</title>
|
|
<meta property="og:title" content={documentTitle} />
|
|
{contentChild && <meta name="description" content={contentChild} />}
|
|
</Helmet>
|
|
<h1>{title}</h1>
|
|
{toReactComponent(['section', { className: 'markdown' }].concat(getChildren(content)))}
|
|
<Divider />
|
|
{menuItems
|
|
.filter(i => i.order > -1)
|
|
.map(group => (
|
|
<div key={group.title} className="components-overview">
|
|
<Title level={2} className="components-overview-group-title">
|
|
<Space align="center">
|
|
{group.title}
|
|
<Tag style={{ display: 'block' }}>{group.children.length}</Tag>
|
|
</Space>
|
|
</Title>
|
|
<Row gutter={[24, 24]}>
|
|
{group.children
|
|
.sort((a, b) => a.title.charCodeAt(0) - b.title.charCodeAt(0))
|
|
.map(component => {
|
|
const url = `${component.filename
|
|
.replace(/(\/index)?((\.zh-cn)|(\.en-us))?\.md$/i, '')
|
|
.toLowerCase()}/`;
|
|
return (
|
|
<Col xs={24} sm={12} lg={8} xl={6} key={component.title}>
|
|
<Link to={getLocalizedPathname(url, locale === 'zh-CN')}>
|
|
<Card
|
|
size="small"
|
|
className="components-overview-card"
|
|
title={
|
|
<div className="components-overview-title">
|
|
{component.title} {component.subtitle}
|
|
</div>
|
|
}
|
|
>
|
|
<div className="components-overview-img">
|
|
<img src={component.cover} alt={component.title} />
|
|
</div>
|
|
</Card>
|
|
</Link>
|
|
</Col>
|
|
);
|
|
})}
|
|
</Row>
|
|
</div>
|
|
))}
|
|
</section>
|
|
);
|
|
};
|
|
|
|
export default ComponentOverview;
|