mirror of
https://github.com/ant-design/ant-design.git
synced 2024-11-24 02:59:58 +08:00
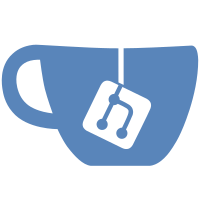
* docs: update select opt * docs: update form deps demo * docs: upload onChange * docs: update form nest Form.List demo
58 lines
1.4 KiB
TypeScript
58 lines
1.4 KiB
TypeScript
import React from 'react';
|
|
import { Alert, Form, Input, Typography } from 'antd';
|
|
|
|
const App: React.FC = () => {
|
|
const [form] = Form.useForm();
|
|
return (
|
|
<Form
|
|
form={form}
|
|
name="dependencies"
|
|
autoComplete="off"
|
|
style={{ maxWidth: 600 }}
|
|
layout="vertical"
|
|
>
|
|
<Alert message=" Try modify `Password2` and then modify `Password`" type="info" showIcon />
|
|
|
|
<Form.Item label="Password" name="password" rules={[{ required: true }]}>
|
|
<Input />
|
|
</Form.Item>
|
|
|
|
{/* Field */}
|
|
<Form.Item
|
|
label="Confirm Password"
|
|
name="password2"
|
|
dependencies={['password']}
|
|
rules={[
|
|
{
|
|
required: true,
|
|
},
|
|
({ getFieldValue }) => ({
|
|
validator(_, value) {
|
|
if (!value || getFieldValue('password') === value) {
|
|
return Promise.resolve();
|
|
}
|
|
return Promise.reject(new Error('The new password that you entered do not match!'));
|
|
},
|
|
}),
|
|
]}
|
|
>
|
|
<Input />
|
|
</Form.Item>
|
|
|
|
{/* Render Props */}
|
|
<Form.Item noStyle dependencies={['password2']}>
|
|
{() => (
|
|
<Typography>
|
|
<p>
|
|
Only Update when <code>password2</code> updated:
|
|
</p>
|
|
<pre>{JSON.stringify(form.getFieldsValue(), null, 2)}</pre>
|
|
</Typography>
|
|
)}
|
|
</Form.Item>
|
|
</Form>
|
|
);
|
|
};
|
|
|
|
export default App;
|