mirror of
https://github.com/ant-design/ant-design.git
synced 2024-11-24 19:19:57 +08:00
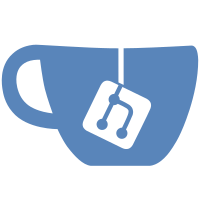
* feat: config support container * feat: add test * feat: reset demo * feat: test * feat: test * feat: test * feat: test * feat: md * feat: limit * fix: prefix * feat: limit * feat: message support app config * feat: notification support app config * feat: add maxCount test * feat: icons * feat: 增加 2 个 todo * feat: holderRender * feat: md * feat: message 兼容旧逻辑 * feat: notification 兼容完成 * feat: modal 兼容完成 * feat: 优化 modal * feat: 优化代码 * feat: 优化代码 * feat: 给 CP 添加 demo * feat: 优先级完成/demo迁移完成 * feat: review * feat: review * feat: 优化 modal 代码 * feat: 支持 rtl * feat: 修改注释 * feat: 优化单测 * feat: 优先级注释 * feat: remove getPrefixCls * feat: demo * feat: demo * feat: demo --------- Co-authored-by: MadCcc <madccc@foxmail.com>
190 lines
5.6 KiB
TypeScript
190 lines
5.6 KiB
TypeScript
import React from 'react';
|
|
|
|
import notification, { actDestroy, actWrapper } from '..';
|
|
import { act } from '../../../tests/utils';
|
|
import App from '../../app';
|
|
import ConfigProvider from '../../config-provider';
|
|
import { awaitPromise, triggerMotionEnd } from './util';
|
|
|
|
describe('notification.config', () => {
|
|
beforeAll(() => {
|
|
actWrapper(act);
|
|
});
|
|
|
|
beforeEach(() => {
|
|
jest.useFakeTimers();
|
|
});
|
|
|
|
afterEach(async () => {
|
|
// Clean up
|
|
notification.destroy();
|
|
await triggerMotionEnd();
|
|
|
|
notification.config({
|
|
prefixCls: undefined,
|
|
getContainer: undefined,
|
|
});
|
|
|
|
jest.useRealTimers();
|
|
|
|
await awaitPromise();
|
|
});
|
|
|
|
it('should be able to config maxCount', async () => {
|
|
notification.config({
|
|
maxCount: 5,
|
|
duration: 0.5,
|
|
});
|
|
|
|
for (let i = 0; i < 10; i += 1) {
|
|
notification.open({
|
|
message: 'Notification message',
|
|
key: i,
|
|
duration: 999,
|
|
});
|
|
|
|
// eslint-disable-next-line no-await-in-loop
|
|
await awaitPromise();
|
|
|
|
act(() => {
|
|
// One frame is 16ms
|
|
jest.advanceTimersByTime(100);
|
|
});
|
|
|
|
// eslint-disable-next-line no-await-in-loop
|
|
await triggerMotionEnd(false);
|
|
|
|
const count = document.querySelectorAll('.ant-notification-notice').length;
|
|
expect(count).toBeLessThanOrEqual(5);
|
|
}
|
|
|
|
act(() => {
|
|
notification.open({
|
|
message: 'Notification last',
|
|
key: '11',
|
|
duration: 999,
|
|
});
|
|
});
|
|
|
|
act(() => {
|
|
// One frame is 16ms
|
|
jest.advanceTimersByTime(100);
|
|
});
|
|
await triggerMotionEnd(false);
|
|
|
|
expect(document.querySelectorAll('.ant-notification-notice')).toHaveLength(5);
|
|
expect(document.querySelectorAll('.ant-notification-notice')[4].textContent).toBe(
|
|
'Notification last',
|
|
);
|
|
|
|
act(() => {
|
|
jest.runAllTimers();
|
|
});
|
|
act(() => {
|
|
jest.runAllTimers();
|
|
});
|
|
|
|
await triggerMotionEnd(false);
|
|
|
|
expect(document.querySelectorAll('.ant-notification-notice')).toHaveLength(0);
|
|
});
|
|
it('should be able to config holderRender', async () => {
|
|
document.body.innerHTML = '';
|
|
actDestroy();
|
|
ConfigProvider.config({
|
|
holderRender: (children) => (
|
|
<ConfigProvider prefixCls="test" iconPrefixCls="icon">
|
|
{children}
|
|
</ConfigProvider>
|
|
),
|
|
});
|
|
|
|
notification.open({ message: 'Notification message' });
|
|
await awaitPromise();
|
|
expect(document.querySelectorAll('.ant-message')).toHaveLength(0);
|
|
expect(document.querySelectorAll('.anticon-close')).toHaveLength(0);
|
|
expect(document.querySelectorAll('.test-notification')).toHaveLength(1);
|
|
expect(document.querySelectorAll('.icon-close')).toHaveLength(1);
|
|
ConfigProvider.config({ holderRender: undefined });
|
|
});
|
|
it('should be able to config holderRender config rtl', async () => {
|
|
document.body.innerHTML = '';
|
|
actDestroy();
|
|
ConfigProvider.config({
|
|
holderRender: (children) => <ConfigProvider direction="rtl">{children}</ConfigProvider>,
|
|
});
|
|
notification.open({ message: 'Notification message' });
|
|
await awaitPromise();
|
|
expect(document.querySelector('.ant-notification-rtl')).toBeTruthy();
|
|
|
|
document.body.innerHTML = '';
|
|
actDestroy();
|
|
notification.config({ rtl: true });
|
|
notification.open({ message: 'Notification message' });
|
|
await awaitPromise();
|
|
expect(document.querySelector('.ant-notification-rtl')).toBeTruthy();
|
|
|
|
document.body.innerHTML = '';
|
|
actDestroy();
|
|
notification.config({ rtl: false });
|
|
notification.open({ message: 'Notification message' });
|
|
await awaitPromise();
|
|
expect(document.querySelector('.ant-notification-rtl')).toBeFalsy();
|
|
|
|
notification.config({ rtl: undefined });
|
|
ConfigProvider.config({ holderRender: undefined });
|
|
});
|
|
it('should be able to config holderRender and static config', async () => {
|
|
// level 1
|
|
document.body.innerHTML = '';
|
|
actDestroy();
|
|
ConfigProvider.config({ prefixCls: 'prefix-1' });
|
|
notification.open({ message: 'Notification message' });
|
|
await awaitPromise();
|
|
expect(document.querySelectorAll('.prefix-1-notification')).toHaveLength(1);
|
|
|
|
// level 2
|
|
document.body.innerHTML = '';
|
|
actDestroy();
|
|
ConfigProvider.config({
|
|
prefixCls: 'prefix-1',
|
|
holderRender: (children) => <ConfigProvider prefixCls="prefix-2">{children}</ConfigProvider>,
|
|
});
|
|
notification.open({ message: 'Notification message' });
|
|
await awaitPromise();
|
|
expect(document.querySelectorAll('.prefix-2-notification')).toHaveLength(1);
|
|
|
|
// level 3
|
|
document.body.innerHTML = '';
|
|
actDestroy();
|
|
notification.config({ prefixCls: 'prefix-3-notification' });
|
|
notification.open({ message: 'Notification message' });
|
|
await awaitPromise();
|
|
expect(document.querySelectorAll('.prefix-3-notification')).toHaveLength(1);
|
|
|
|
// clear config
|
|
notification.config({ prefixCls: '' });
|
|
ConfigProvider.config({ prefixCls: '', iconPrefixCls: '', holderRender: undefined });
|
|
});
|
|
|
|
it('should be able to config holderRender use App', async () => {
|
|
document.body.innerHTML = '';
|
|
actDestroy();
|
|
ConfigProvider.config({
|
|
holderRender: (children) => <App notification={{ maxCount: 1 }}>{children}</App>,
|
|
});
|
|
|
|
notification.open({ message: 'Notification message' });
|
|
notification.open({ message: 'Notification message' });
|
|
|
|
await awaitPromise();
|
|
const noticeWithoutLeaving = Array.from(
|
|
document.querySelectorAll('.ant-notification-notice-wrapper'),
|
|
).filter((ele) => !ele.classList.contains('ant-notification-fade-leave'));
|
|
|
|
expect(noticeWithoutLeaving).toHaveLength(1);
|
|
|
|
ConfigProvider.config({ holderRender: undefined });
|
|
});
|
|
});
|