mirror of
https://github.com/ocornut/imgui.git
synced 2024-12-12 20:19:02 +08:00
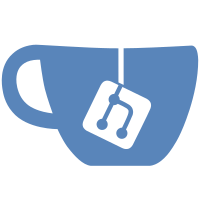
Currently, the implementation headers don't include the imgui.h header. Which means that the compilation will fail if the implementation header was included before the imgui.h header in the compilation unit. For instance, a compilation unit with the following will work: #include "imgui.h" #include "imgui_impl_glfw.h" #include "imgui_impl_opengl3.h" But a compilation unit with the following will fail because IMGUI_IMPL_API and possibly other symbols will not be defined: #include "imgui_impl_glfw.h" #include "imgui_impl_opengl3.h" #include "imgui.h" This patch includes imgui.h in the implementation headers to make inclusions order-invariant, which is a recommended practice.
31 lines
1.7 KiB
C
31 lines
1.7 KiB
C
// dear imgui: Platform Binding for SDL2
|
|
// This needs to be used along with a Renderer (e.g. DirectX11, OpenGL3, Vulkan..)
|
|
// (Info: SDL2 is a cross-platform general purpose library for handling windows, inputs, graphics context creation, etc.)
|
|
|
|
// Implemented features:
|
|
// [X] Platform: Mouse cursor shape and visibility. Disable with 'io.ConfigFlags |= ImGuiConfigFlags_NoMouseCursorChange'.
|
|
// [X] Platform: Clipboard support.
|
|
// [X] Platform: Keyboard arrays indexed using SDL_SCANCODE_* codes, e.g. ImGui::IsKeyPressed(SDL_SCANCODE_SPACE).
|
|
// [X] Platform: Gamepad support. Enabled with 'io.ConfigFlags |= ImGuiConfigFlags_NavEnableGamepad'.
|
|
// Missing features:
|
|
// [ ] Platform: SDL2 handling of IME under Windows appears to be broken and it explicitly disable the regular Windows IME. You can restore Windows IME by compiling SDL with SDL_DISABLE_WINDOWS_IME.
|
|
|
|
// You can copy and use unmodified imgui_impl_* files in your project. See main.cpp for an example of using this.
|
|
// If you are new to dear imgui, read examples/README.txt and read the documentation at the top of imgui.cpp.
|
|
// https://github.com/ocornut/imgui
|
|
|
|
#pragma once
|
|
|
|
#include "imgui.h"
|
|
|
|
struct SDL_Window;
|
|
typedef union SDL_Event SDL_Event;
|
|
|
|
IMGUI_IMPL_API bool ImGui_ImplSDL2_InitForOpenGL(SDL_Window* window, void* sdl_gl_context);
|
|
IMGUI_IMPL_API bool ImGui_ImplSDL2_InitForVulkan(SDL_Window* window);
|
|
IMGUI_IMPL_API bool ImGui_ImplSDL2_InitForD3D(SDL_Window* window);
|
|
IMGUI_IMPL_API bool ImGui_ImplSDL2_InitForMetal(SDL_Window* window);
|
|
IMGUI_IMPL_API void ImGui_ImplSDL2_Shutdown();
|
|
IMGUI_IMPL_API void ImGui_ImplSDL2_NewFrame(SDL_Window* window);
|
|
IMGUI_IMPL_API bool ImGui_ImplSDL2_ProcessEvent(const SDL_Event* event);
|