mirror of
https://github.com/opencv/opencv.git
synced 2025-01-11 06:48:19 +08:00
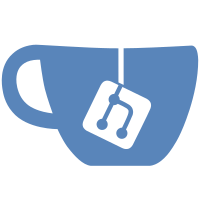
Highgui backend on top of Framebuffer #25661 ### Pull Request Readiness Checklist See details at https://github.com/opencv/opencv/wiki/How_to_contribute#making-a-good-pull-request - [ x] I agree to contribute to the project under Apache 2 License. - [ x] To the best of my knowledge, the proposed patch is not based on a code under GPL or another license that is incompatible with OpenCV - [ x] The PR is proposed to the proper branch - [ ] There is a reference to the original bug report and related work - [ x] There is accuracy test, performance test and test data in opencv_extra repository, if applicable Patch to opencv_extra has the same branch name. - [ x] The feature is well documented and sample code can be built with the project CMake Environment variables used: OPENCV_UI_BACKEND - you need to add the value “FB” OPENCV_UI_PRIORITY_FB - requires priority indication OPENCV_HIGHGUI_FB_MODE={FB|XVFB|EMU} - mode of using Framebuffer (default "FB") - FB - Linux Framebuffer - XVFB - virtual Framebuffer - EMU - emulation (images are not displayed) OPENCV_HIGHGUI_FB_DEVICE (FRAMEBUFFER) - path to the Framebuffer file (default "/dev/fb0"). Examples of using: sudo OPENCV_UI_BACKEND=FB ./opencv_test_highgui sudo OPENCV_UI_PRIORITY_FB=1111 ./opencv_test_highgui OPENCV_UI_BACKEND=FB OPENCV_HIGHGUI_FB_MODE=EMU ./opencv_test_highgui sudo OPENCV_UI_BACKEND=FB OPENCV_HIGHGUI_FB_MODE=FB ./opencv_test_highgui export DISPLAY=:99 Xvfb $DISPLAY -screen 0 1024x768x24 -fbdir /tmp/ -f /tmp/user.xvfb.auth& sudo -u sipeed XAUTHORITY=/tmp/user.xvfb.auth x11vnc -display $DISPLAY -listen localhost& DISPLAY=:0 gvncviewer localhost& FRAMEBUFFER=/tmp/Xvfb_screen0 OPENCV_UI_BACKEND=FB OPENCV_HIGHGUI_FB_MODE=XVFB ./opencv_test_highgui
136 lines
3.2 KiB
C++
136 lines
3.2 KiB
C++
// This file is part of OpenCV project.
|
|
// It is subject to the license terms in the LICENSE file found in the top-level directory
|
|
// of this distribution and at http://opencv.org/license.html.
|
|
|
|
#ifndef OPENCV_HIGHGUI_WINDOWS_FRAMEBUFFER_HPP
|
|
#define OPENCV_HIGHGUI_WINDOWS_FRAMEBUFFER_HPP
|
|
|
|
#include "backend.hpp"
|
|
|
|
#include <linux/fb.h>
|
|
#include <linux/input.h>
|
|
|
|
#include <termios.h>
|
|
|
|
namespace cv {
|
|
namespace highgui_backend {
|
|
|
|
enum OpenCVFBMode{
|
|
FB_MODE_EMU,
|
|
FB_MODE_FB,
|
|
FB_MODE_XVFB
|
|
};
|
|
|
|
class FramebufferBackend;
|
|
class FramebufferWindow : public UIWindow
|
|
{
|
|
FramebufferBackend &backend;
|
|
std::string FB_ID;
|
|
Rect windowRect;
|
|
|
|
int flags;
|
|
Mat currentImg;
|
|
|
|
public:
|
|
FramebufferWindow(FramebufferBackend &backend, int flags);
|
|
virtual ~FramebufferWindow();
|
|
|
|
virtual void imshow(InputArray image) override;
|
|
|
|
virtual double getProperty(int prop) const override;
|
|
virtual bool setProperty(int prop, double value) override;
|
|
|
|
virtual void resize(int width, int height) override;
|
|
virtual void move(int x, int y) override;
|
|
|
|
virtual Rect getImageRect() const override;
|
|
|
|
virtual void setTitle(const std::string& title) override;
|
|
|
|
virtual void setMouseCallback(MouseCallback onMouse, void* userdata /*= 0*/) override;
|
|
|
|
virtual std::shared_ptr<UITrackbar> createTrackbar(
|
|
const std::string& name,
|
|
int count,
|
|
TrackbarCallback onChange /*= 0*/,
|
|
void* userdata /*= 0*/
|
|
) override;
|
|
|
|
virtual std::shared_ptr<UITrackbar> findTrackbar(const std::string& name) override;
|
|
|
|
virtual const std::string& getID() const override;
|
|
|
|
virtual bool isActive() const override;
|
|
|
|
virtual void destroy() override;
|
|
}; // FramebufferWindow
|
|
|
|
class FramebufferBackend: public UIBackend
|
|
{
|
|
OpenCVFBMode mode;
|
|
|
|
struct termios old, current;
|
|
|
|
void initTermios(int echo, int wait);
|
|
void resetTermios(void);
|
|
int getch_(int echo, int wait);
|
|
bool kbhit();
|
|
|
|
fb_var_screeninfo varInfo;
|
|
fb_fix_screeninfo fixInfo;
|
|
int fbWidth;
|
|
int fbHeight;
|
|
int fbXOffset;
|
|
int fbYOffset;
|
|
int fbBitsPerPixel;
|
|
int fbLineLength;
|
|
long int fbScreenSize;
|
|
unsigned char* fbPointer;
|
|
unsigned int fbPointer_dist;
|
|
Mat backgroundBuff;
|
|
|
|
int fbOpenAndGetInfo();
|
|
int fbID;
|
|
|
|
unsigned int xvfb_len_header;
|
|
unsigned int xvfb_len_colors;
|
|
unsigned int xvfb_len_pixmap;
|
|
int XvfbOpenAndGetInfo();
|
|
|
|
public:
|
|
|
|
fb_var_screeninfo &getVarInfo();
|
|
fb_fix_screeninfo &getFixInfo();
|
|
int getFramebuffrerID();
|
|
int getFBWidth();
|
|
int getFBHeight();
|
|
int getFBXOffset();
|
|
int getFBYOffset();
|
|
int getFBBitsPerPixel();
|
|
int getFBLineLength();
|
|
unsigned char* getFBPointer();
|
|
Mat& getBackgroundBuff();
|
|
OpenCVFBMode getMode();
|
|
|
|
FramebufferBackend();
|
|
|
|
virtual ~FramebufferBackend();
|
|
|
|
virtual void destroyAllWindows()override;
|
|
|
|
// namedWindow
|
|
virtual std::shared_ptr<UIWindow> createWindow(
|
|
const std::string& winname,
|
|
int flags
|
|
)override;
|
|
|
|
virtual int waitKeyEx(int delay /*= 0*/)override;
|
|
virtual int pollKey() override;
|
|
|
|
virtual const std::string getName() const override;
|
|
};
|
|
|
|
}} // cv::highgui_backend::
|
|
|
|
#endif
|