mirror of
https://github.com/opencv/opencv.git
synced 2024-12-05 01:39:13 +08:00
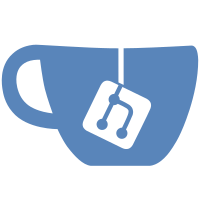
Move Charuco/Calib tutorials and samples to main repo #25378 Merge with https://github.com/opencv/opencv_contrib/pull/3708 Move Charuco/Calib tutorials and samples to main repo: - [x] update/fix charuco_detection.markdown and samples - [x] update/fix charuco_diamond_detection.markdown and samples - [x] update/fix aruco_calibration.markdown and samples - [x] update/fix aruco_faq.markdown - [x] move tutorials, samples and tests to main repo - [x] remove old tutorials, samples and tests from contrib ### Pull Request Readiness Checklist See details at https://github.com/opencv/opencv/wiki/How_to_contribute#making-a-good-pull-request - [x] I agree to contribute to the project under Apache 2 License. - [x] To the best of my knowledge, the proposed patch is not based on a code under GPL or another license that is incompatible with OpenCV - [x] The PR is proposed to the proper branch - [x] There is a reference to the original bug report and related work - [x] There is accuracy test, performance test and test data in opencv_extra repository, if applicable Patch to opencv_extra has the same branch name. - [ ] The feature is well documented and sample code can be built with the project CMake
73 lines
2.6 KiB
C++
73 lines
2.6 KiB
C++
#include <opencv2/highgui.hpp>
|
|
#include <opencv2/objdetect/charuco_detector.hpp>
|
|
#include <vector>
|
|
#include <iostream>
|
|
#include "aruco_samples_utility.hpp"
|
|
|
|
using namespace std;
|
|
using namespace cv;
|
|
|
|
namespace {
|
|
const char* about = "Create a ChArUco marker image";
|
|
const char* keys =
|
|
"{@outfile | res.png | Output image }"
|
|
"{sl | 100 | Square side length (in pixels) }"
|
|
"{ml | 60 | Marker side length (in pixels) }"
|
|
"{cd | | Input file with custom dictionary }"
|
|
"{d | 10 | dictionary: DICT_4X4_50=0, DICT_4X4_100=1, DICT_4X4_250=2,"
|
|
"DICT_4X4_1000=3, DICT_5X5_50=4, DICT_5X5_100=5, DICT_5X5_250=6, DICT_5X5_1000=7, "
|
|
"DICT_6X6_50=8, DICT_6X6_100=9, DICT_6X6_250=10, DICT_6X6_1000=11, DICT_7X7_50=12,"
|
|
"DICT_7X7_100=13, DICT_7X7_250=14, DICT_7X7_1000=15, DICT_ARUCO_ORIGINAL = 16}"
|
|
"{ids |0, 1, 2, 3 | Four ids for the ChArUco marker: id1,id2,id3,id4 }"
|
|
"{m | 0 | Margins size (in pixels) }"
|
|
"{bb | 1 | Number of bits in marker borders }"
|
|
"{si | false | show generated image }";
|
|
}
|
|
|
|
int main(int argc, char *argv[]) {
|
|
CommandLineParser parser(argc, argv, keys);
|
|
parser.about(about);
|
|
|
|
int squareLength = parser.get<int>("sl");
|
|
int markerLength = parser.get<int>("ml");
|
|
string idsString = parser.get<string>("ids");
|
|
int margins = parser.get<int>("m");
|
|
int borderBits = parser.get<int>("bb");
|
|
bool showImage = parser.get<bool>("si");
|
|
string out = parser.get<string>(0);
|
|
aruco::Dictionary dictionary = readDictionatyFromCommandLine(parser);
|
|
|
|
if(!parser.check()) {
|
|
parser.printErrors();
|
|
return 0;
|
|
}
|
|
|
|
istringstream ss(idsString);
|
|
vector<string> splittedIds;
|
|
string token;
|
|
while(getline(ss, token, ','))
|
|
splittedIds.push_back(token);
|
|
if(splittedIds.size() < 4) {
|
|
throw std::runtime_error("Incorrect ids format\n");
|
|
}
|
|
Vec4i ids;
|
|
for(int i = 0; i < 4; i++)
|
|
ids[i] = atoi(splittedIds[i].c_str());
|
|
|
|
//! [generate_diamond]
|
|
vector<int> diamondIds = {ids[0], ids[1], ids[2], ids[3]};
|
|
aruco::CharucoBoard charucoBoard(Size(3, 3), (float)squareLength, (float)markerLength, dictionary, diamondIds);
|
|
Mat markerImg;
|
|
charucoBoard.generateImage(Size(3*squareLength + 2*margins, 3*squareLength + 2*margins), markerImg, margins, borderBits);
|
|
//! [generate_diamond]
|
|
|
|
if(showImage) {
|
|
imshow("board", markerImg);
|
|
waitKey(0);
|
|
}
|
|
|
|
if (out != "")
|
|
imwrite(out, markerImg);
|
|
return 0;
|
|
}
|