mirror of
https://github.com/opencv/opencv.git
synced 2024-12-05 01:39:13 +08:00
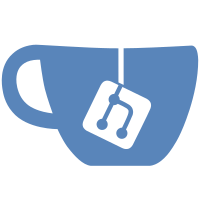
Move Charuco/Calib tutorials and samples to main repo #25378 Merge with https://github.com/opencv/opencv_contrib/pull/3708 Move Charuco/Calib tutorials and samples to main repo: - [x] update/fix charuco_detection.markdown and samples - [x] update/fix charuco_diamond_detection.markdown and samples - [x] update/fix aruco_calibration.markdown and samples - [x] update/fix aruco_faq.markdown - [x] move tutorials, samples and tests to main repo - [x] remove old tutorials, samples and tests from contrib ### Pull Request Readiness Checklist See details at https://github.com/opencv/opencv/wiki/How_to_contribute#making-a-good-pull-request - [x] I agree to contribute to the project under Apache 2 License. - [x] To the best of my knowledge, the proposed patch is not based on a code under GPL or another license that is incompatible with OpenCV - [x] The PR is proposed to the proper branch - [x] There is a reference to the original bug report and related work - [x] There is accuracy test, performance test and test data in opencv_extra repository, if applicable Patch to opencv_extra has the same branch name. - [ ] The feature is well documented and sample code can be built with the project CMake
156 lines
5.4 KiB
C++
156 lines
5.4 KiB
C++
#include <iostream>
|
|
#include <vector>
|
|
#include <opencv2/highgui.hpp>
|
|
#include <opencv2/objdetect/aruco_detector.hpp>
|
|
#include "aruco_samples_utility.hpp"
|
|
|
|
using namespace std;
|
|
using namespace cv;
|
|
|
|
namespace {
|
|
const char* about = "Pose estimation using a ArUco Planar Grid board";
|
|
|
|
//! [aruco_detect_board_keys]
|
|
const char* keys =
|
|
"{w | | Number of squares in X direction }"
|
|
"{h | | Number of squares in Y direction }"
|
|
"{l | | Marker side length (in pixels) }"
|
|
"{s | | Separation between two consecutive markers in the grid (in pixels)}"
|
|
"{d | | dictionary: DICT_4X4_50=0, DICT_4X4_100=1, DICT_4X4_250=2,"
|
|
"DICT_4X4_1000=3, DICT_5X5_50=4, DICT_5X5_100=5, DICT_5X5_250=6, DICT_5X5_1000=7, "
|
|
"DICT_6X6_50=8, DICT_6X6_100=9, DICT_6X6_250=10, DICT_6X6_1000=11, DICT_7X7_50=12,"
|
|
"DICT_7X7_100=13, DICT_7X7_250=14, DICT_7X7_1000=15, DICT_ARUCO_ORIGINAL = 16}"
|
|
"{cd | | Input file with custom dictionary }"
|
|
"{c | | Output file with calibrated camera parameters }"
|
|
"{v | | Input from video or image file, if omitted, input comes from camera }"
|
|
"{ci | 0 | Camera id if input doesnt come from video (-v) }"
|
|
"{dp | | File of marker detector parameters }"
|
|
"{rs | | Apply refind strategy }"
|
|
"{r | | show rejected candidates too }";
|
|
}
|
|
//! [aruco_detect_board_keys]
|
|
|
|
int main(int argc, char *argv[]) {
|
|
CommandLineParser parser(argc, argv, keys);
|
|
parser.about(about);
|
|
|
|
if(argc < 7) {
|
|
parser.printMessage();
|
|
return 0;
|
|
}
|
|
|
|
//! [aruco_detect_board_full_sample]
|
|
int markersX = parser.get<int>("w");
|
|
int markersY = parser.get<int>("h");
|
|
float markerLength = parser.get<float>("l");
|
|
float markerSeparation = parser.get<float>("s");
|
|
bool showRejected = parser.has("r");
|
|
bool refindStrategy = parser.has("rs");
|
|
int camId = parser.get<int>("ci");
|
|
|
|
|
|
Mat camMatrix, distCoeffs;
|
|
readCameraParamsFromCommandLine(parser, camMatrix, distCoeffs);
|
|
aruco::Dictionary dictionary = readDictionatyFromCommandLine(parser);
|
|
aruco::DetectorParameters detectorParams = readDetectorParamsFromCommandLine(parser);
|
|
|
|
String video;
|
|
if(parser.has("v")) {
|
|
video = parser.get<String>("v");
|
|
}
|
|
|
|
if(!parser.check()) {
|
|
parser.printErrors();
|
|
return 0;
|
|
}
|
|
|
|
aruco::ArucoDetector detector(dictionary, detectorParams);
|
|
VideoCapture inputVideo;
|
|
int waitTime;
|
|
if(!video.empty()) {
|
|
inputVideo.open(video);
|
|
waitTime = 0;
|
|
} else {
|
|
inputVideo.open(camId);
|
|
waitTime = 10;
|
|
}
|
|
|
|
float axisLength = 0.5f * ((float)min(markersX, markersY) * (markerLength + markerSeparation) +
|
|
markerSeparation);
|
|
|
|
// Create GridBoard object
|
|
//! [aruco_create_board]
|
|
aruco::GridBoard board(Size(markersX, markersY), markerLength, markerSeparation, dictionary);
|
|
//! [aruco_create_board]
|
|
|
|
// Also you could create Board object
|
|
//vector<vector<Point3f> > objPoints; // array of object points of all the marker corners in the board
|
|
//vector<int> ids; // vector of the identifiers of the markers in the board
|
|
//aruco::Board board(objPoints, dictionary, ids);
|
|
|
|
double totalTime = 0;
|
|
int totalIterations = 0;
|
|
|
|
while(inputVideo.grab()) {
|
|
Mat image, imageCopy;
|
|
inputVideo.retrieve(image);
|
|
|
|
double tick = (double)getTickCount();
|
|
|
|
vector<int> ids;
|
|
vector<vector<Point2f>> corners, rejected;
|
|
Vec3d rvec, tvec;
|
|
|
|
//! [aruco_detect_and_refine]
|
|
|
|
// Detect markers
|
|
detector.detectMarkers(image, corners, ids, rejected);
|
|
|
|
// Refind strategy to detect more markers
|
|
if(refindStrategy)
|
|
detector.refineDetectedMarkers(image, board, corners, ids, rejected, camMatrix,
|
|
distCoeffs);
|
|
|
|
//! [aruco_detect_and_refine]
|
|
|
|
// Estimate board pose
|
|
int markersOfBoardDetected = 0;
|
|
if(!ids.empty()) {
|
|
// Get object and image points for the solvePnP function
|
|
cv::Mat objPoints, imgPoints;
|
|
board.matchImagePoints(corners, ids, objPoints, imgPoints);
|
|
|
|
// Find pose
|
|
cv::solvePnP(objPoints, imgPoints, camMatrix, distCoeffs, rvec, tvec);
|
|
|
|
markersOfBoardDetected = (int)objPoints.total() / 4;
|
|
}
|
|
|
|
double currentTime = ((double)getTickCount() - tick) / getTickFrequency();
|
|
totalTime += currentTime;
|
|
totalIterations++;
|
|
if(totalIterations % 30 == 0) {
|
|
cout << "Detection Time = " << currentTime * 1000 << " ms "
|
|
<< "(Mean = " << 1000 * totalTime / double(totalIterations) << " ms)" << endl;
|
|
}
|
|
|
|
// Draw results
|
|
image.copyTo(imageCopy);
|
|
if(!ids.empty())
|
|
aruco::drawDetectedMarkers(imageCopy, corners, ids);
|
|
|
|
if(showRejected && !rejected.empty())
|
|
aruco::drawDetectedMarkers(imageCopy, rejected, noArray(), Scalar(100, 0, 255));
|
|
|
|
if(markersOfBoardDetected > 0)
|
|
cv::drawFrameAxes(imageCopy, camMatrix, distCoeffs, rvec, tvec, axisLength);
|
|
|
|
imshow("out", imageCopy);
|
|
char key = (char)waitKey(waitTime);
|
|
if(key == 27) break;
|
|
//! [aruco_detect_board_full_sample]
|
|
}
|
|
|
|
return 0;
|
|
}
|