mirror of
https://github.com/opencv/opencv.git
synced 2024-12-16 02:19:12 +08:00
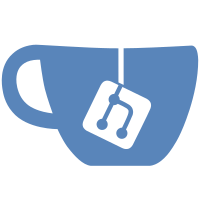
Added Fastcv HAL changes in the 3rdparty folder. Code Changes includes HAL code , Fastcv libs and Headers Change-Id: I2f0ddb1f57515c82ae86ba8c2a82965b1a9626ec Requires binaries from https://github.com/opencv/opencv_3rdparty/pull/86. Related patch to opencv_contrib: https://github.com/opencv/opencv_contrib/pull/3811 ### Pull Request Readiness Checklist See details at https://github.com/opencv/opencv/wiki/How_to_contribute#making-a-good-pull-request - [x] I agree to contribute to the project under Apache 2 License. - [x] To the best of my knowledge, the proposed patch is not based on a code under GPL or another license that is incompatible with OpenCV - [ ] The PR is proposed to the proper branch - [ ] There is a reference to the original bug report and related work - [ ] There is accuracy test, performance test and test data in opencv_extra repository, if applicable Patch to opencv_extra has the same branch name. - [ ] The feature is well documented and sample code can be built with the project CMake
83 lines
3.4 KiB
C++
83 lines
3.4 KiB
C++
/*
|
|
* Copyright (c) 2024 Qualcomm Innovation Center, Inc. All rights reserved.
|
|
* SPDX-License-Identifier: Apache-2.0
|
|
*/
|
|
|
|
#ifndef OPENCV_FASTCV_HAL_UTILS_HPP_INCLUDED
|
|
#define OPENCV_FASTCV_HAL_UTILS_HPP_INCLUDED
|
|
|
|
#include "fastcv.h"
|
|
#include <opencv2/core/utils/logger.hpp>
|
|
|
|
#define INITIALIZATION_CHECK \
|
|
{ \
|
|
if (!FastCvContext::getContext().isInitialized) \
|
|
{ \
|
|
return CV_HAL_ERROR_UNKNOWN; \
|
|
} \
|
|
}
|
|
|
|
#define CV_HAL_RETURN(status, func) \
|
|
{ \
|
|
if( status == FASTCV_SUCCESS ) \
|
|
{ \
|
|
CV_LOG_DEBUG(NULL, "FastCV HAL for "<<#func<<" run successfully!"); \
|
|
return CV_HAL_ERROR_OK; \
|
|
} \
|
|
else if(status == FASTCV_EBADPARAM || status == FASTCV_EUNALIGNPARAM || \
|
|
status == FASTCV_EUNSUPPORTED || status == FASTCV_EHWQDSP || \
|
|
status == FASTCV_EHWGPU) \
|
|
{ \
|
|
CV_LOG_DEBUG(NULL, "FastCV status:"<<getFastCVErrorString(status) \
|
|
<<"Switching to default OpenCV solution!"); \
|
|
return CV_HAL_ERROR_NOT_IMPLEMENTED; \
|
|
} \
|
|
else \
|
|
{ \
|
|
CV_LOG_ERROR(NULL,"FastCV error:"<<getFastCVErrorString(status)); \
|
|
return CV_HAL_ERROR_UNKNOWN; \
|
|
} \
|
|
}
|
|
|
|
#define CV_HAL_RETURN_NOT_IMPLEMENTED(reason) \
|
|
{ \
|
|
CV_LOG_DEBUG(NULL,"Switching to default OpenCV\nInfo: "<<reason); \
|
|
return CV_HAL_ERROR_NOT_IMPLEMENTED; \
|
|
}
|
|
|
|
#define FCV_KernelSize_SHIFT 3
|
|
#define FCV_MAKETYPE(ksize,depth) ((ksize<<FCV_KernelSize_SHIFT) + depth)
|
|
|
|
const char* getFastCVErrorString(int status);
|
|
const char* borderToString(int border);
|
|
const char* interpolationToString(int interpolation);
|
|
|
|
struct FastCvContext
|
|
{
|
|
public:
|
|
// initialize at first call
|
|
// Defines a static local variable context. Variable is created only once.
|
|
static FastCvContext& getContext()
|
|
{
|
|
static FastCvContext context;
|
|
return context;
|
|
}
|
|
|
|
FastCvContext()
|
|
{
|
|
if (fcvSetOperationMode(FASTCV_OP_CPU_PERFORMANCE) != 0)
|
|
{
|
|
CV_LOG_WARNING(NULL, "Failed to switch FastCV operation mode");
|
|
isInitialized = false;
|
|
}
|
|
else
|
|
{
|
|
CV_LOG_INFO(NULL, "FastCV Operation Mode Switched");
|
|
isInitialized = true;
|
|
}
|
|
}
|
|
|
|
bool isInitialized;
|
|
};
|
|
|
|
#endif |