mirror of
https://github.com/opencv/opencv.git
synced 2024-12-12 23:49:36 +08:00
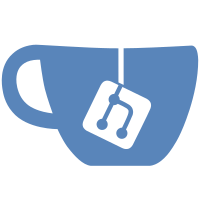
Additional fixes to 0/1D tests #25487 This has additional fixes requited for 0/1D tests. ### Pull Request Readiness Checklist See details at https://github.com/opencv/opencv/wiki/How_to_contribute#making-a-good-pull-request - [x] I agree to contribute to the project under Apache 2 License. - [x] To the best of my knowledge, the proposed patch is not based on a code under GPL or another license that is incompatible with OpenCV - [x] The PR is proposed to the proper branch - [x] There is a reference to the original bug report and related work - [x] There is accuracy test, performance test and test data in opencv_extra repository, if applicable Patch to opencv_extra has the same branch name. - [x] The feature is well documented and sample code can be built with the project CMake
45 lines
1.5 KiB
C++
45 lines
1.5 KiB
C++
// This file is part of OpenCV project.
|
|
// It is subject to the license terms in the LICENSE file found in the top-level directory
|
|
// of this distribution and at http://opencv.org/license.html.
|
|
|
|
#include "test_precomp.hpp"
|
|
#include "test_common.impl.hpp" // shared with perf tests
|
|
#include <opencv2/dnn/shape_utils.hpp>
|
|
|
|
namespace opencv_test {
|
|
void runLayer(cv::Ptr<cv::dnn::Layer> layer, std::vector<cv::Mat> &inpBlobs, std::vector<cv::Mat> &outBlobs)
|
|
{
|
|
size_t ninputs = inpBlobs.size();
|
|
std::vector<cv::Mat> inp(ninputs), outp, intp;
|
|
std::vector<cv::dnn::MatShape> inputs, outputs, internals;
|
|
std::vector<cv::dnn::MatType> inputs_types, outputs_types, internals_types;
|
|
|
|
for (size_t i = 0; i < ninputs; i++)
|
|
{
|
|
inp[i] = inpBlobs[i].clone();
|
|
inputs.push_back(cv::dnn::shape(inp[i]));
|
|
inputs_types.push_back(cv::dnn::MatType(inp[i].type()));
|
|
}
|
|
|
|
layer->getMemoryShapes(inputs, 0, outputs, internals);
|
|
layer->getTypes(inputs_types, outputs.size(), internals.size(), outputs_types, internals_types);
|
|
for (size_t i = 0; i < outputs.size(); i++)
|
|
{
|
|
outp.push_back(cv::Mat(outputs[i], outputs_types[i]));
|
|
}
|
|
for (size_t i = 0; i < internals.size(); i++)
|
|
{
|
|
intp.push_back(cv::Mat(internals[i], internals_types[i]));
|
|
}
|
|
|
|
layer->finalize(inp, outp);
|
|
layer->forward(inp, outp, intp);
|
|
|
|
size_t noutputs = outp.size();
|
|
outBlobs.resize(noutputs);
|
|
for (size_t i = 0; i < noutputs; i++)
|
|
outBlobs[i] = outp[i];
|
|
}
|
|
|
|
}
|