mirror of
https://github.com/opencv/opencv.git
synced 2024-11-26 12:10:49 +08:00
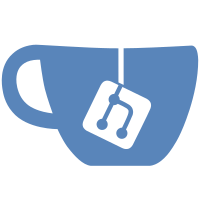
G-API Serialization routines * Serialization backend in tests, initial version * S11N/00: A Great Rename - "Serialization" is too long and too error-prone to type, so now it is renamed to "s11n" everywhere; - Same applies to "SRLZ"; - Tests also renamed to start with 'S11N.*' (easier to run); - Also updated copyright years in new files to 2020. * S11N/01: Some basic interface segregation - Moved some details (low-level functions) out of serialization.hpp; - Introduced I::IStream and I::OStream interfaces; - Implemented those via the existing [De]SerializationStream classes; - Moved all operators to use interfaces instead of classes; - Moved the htonl/ntohl handling out of operators (to the classes). The implementation didn't change much, it is a subject to the further refactoring * S11N/02: Basic operator reorg, basic tests, vector support - Reorganized operators on atomic types to follow >>/<< model (put them closer in the code for the respective types); - Introduce more operators for basic (scalar) types; - Drop all vector s11n overloads -- replace with a generic (template-based) one; - Introduced a new test suite where low-level s11n functionality is tested (for the basic types). * S11N/03: Operators reorganization - Sorted the Opaque types enum by complexity; - Reorganized the existing operators for basic types, also ordered by complexity; - Organized operators in three groups (Basics, OpenCV, G-API); - Added a generic serialization for variant<>; - Reimplemented some of the existing operators (for OpenCV and G-API data structures); - Introduced new operators for cv::gimpl data types. These operators (and so, the data structures) are not yet used in the graph dump/reconstruction routine, it will be done as a next step. * S11N/04: The Great Clean-up - Drop the duplicates of GModel data structures from the serialization, serialize the GModel data structures themselve instead (hand-written code replaced with operators). - Also removed usuned code for printing, etc. * S11N/05: Internal API Clean-up - Minimize the serialization API to just Streams and Operators; - Refactor and fix the graph serialization (deconstruction and reconstruction) routines, fix data addressing problems there; - Move the serialization.[ch]pp files to the core G-API library * S11N/06: Top-level API introduction - !!!This is likely the most invasive commit in the series!!! - Introduced a top-level API to serialize and deserialize a GComputation - Extended the compiler to support both forms of a GComputation: an expession based and a deserialized one. This has led to changes in the cv::GComputation::Priv and in its dependent components (even the transformation tests); - Had to extend the kernel API (GKernel) with extra information on operations (mainly `outMeta`) which was only available for expression based graphs. Now the `outMeta` can be taken from kernels too (and for the deserialized graphs it is the only way); - Revisited the internal serialization API, had to expose previously hidden entities (like `GSerialized`); - Extended the serialized graph info with new details (object counter, protocol). Added unordered_map generic serialization for that; - Reworked the very first pipeline test to be "proper"; GREEN now, the rest is to be reworked in the next iteration. * S11N/07: Tests reworked - Moved the sample pipeline tests w/serialization to test the public API (`cv::gapi::serialize`, then followed by `cv::gapi::deserialize<>`). All GREEN. - As a consequence, dropped the "Serialization" test backend as no longer necessary. * S11N/08: Final touches - Exposed the C++ native data types at Streams level; - Switched the ByteMemoryIn/OutStreams to store data in `char` internally (2x less memory for sample pipelines); - Fixed and refactored Mat dumping to the stream; - Renamed S11N pipeline tests to their new meaning. * linux build fix * fix RcDesc and int uint warnings * more Linux build fix * white space and virtual android error fix (attempt) * more warnings to be fixed * android warnings fix attempt * one more attempt for android build fix * android warnings one more fix * return back override * avoid size_t * static deserialize * and how do you like this, elon? anonymous namespace to fix android warning. * static inline * trying to fix standalone build * mat dims fix * fix mat r/w for standalone Co-authored-by: Dmitry Matveev <dmitry.matveev@intel.com>
198 lines
6.2 KiB
CMake
198 lines
6.2 KiB
CMake
# FIXME: Rework standalone build in more generic maner
|
|
# (Restructure directories, add common pass, etc)
|
|
if(NOT DEFINED OPENCV_INITIAL_PASS)
|
|
cmake_minimum_required(VERSION 3.3)
|
|
project(gapi_standalone)
|
|
include("cmake/standalone.cmake")
|
|
return()
|
|
endif()
|
|
|
|
if(NOT TARGET ade)
|
|
# can't build G-API because of the above reasons
|
|
ocv_module_disable(gapi)
|
|
return()
|
|
endif()
|
|
|
|
if(INF_ENGINE_TARGET)
|
|
ocv_option(OPENCV_GAPI_INF_ENGINE "Build GraphAPI module with Inference Engine support" ON)
|
|
endif()
|
|
|
|
set(the_description "OpenCV G-API Core Module")
|
|
|
|
ocv_add_module(gapi
|
|
REQUIRED
|
|
opencv_imgproc
|
|
OPTIONAL
|
|
opencv_video
|
|
)
|
|
|
|
if(MSVC)
|
|
# Disable obsollete warning C4503 popping up on MSVC <<2017
|
|
# https://docs.microsoft.com/en-us/cpp/error-messages/compiler-warnings/compiler-warning-level-1-c4503?view=vs-2019
|
|
ocv_warnings_disable(CMAKE_CXX_FLAGS /wd4503)
|
|
endif()
|
|
|
|
file(GLOB gapi_ext_hdrs
|
|
"${CMAKE_CURRENT_LIST_DIR}/include/opencv2/*.hpp"
|
|
"${CMAKE_CURRENT_LIST_DIR}/include/opencv2/${name}/*.hpp"
|
|
"${CMAKE_CURRENT_LIST_DIR}/include/opencv2/${name}/*.h"
|
|
"${CMAKE_CURRENT_LIST_DIR}/include/opencv2/${name}/cpu/*.hpp"
|
|
"${CMAKE_CURRENT_LIST_DIR}/include/opencv2/${name}/fluid/*.hpp"
|
|
"${CMAKE_CURRENT_LIST_DIR}/include/opencv2/${name}/gpu/*.hpp"
|
|
"${CMAKE_CURRENT_LIST_DIR}/include/opencv2/${name}/infer/*.hpp"
|
|
"${CMAKE_CURRENT_LIST_DIR}/include/opencv2/${name}/ocl/*.hpp"
|
|
"${CMAKE_CURRENT_LIST_DIR}/include/opencv2/${name}/own/*.hpp"
|
|
"${CMAKE_CURRENT_LIST_DIR}/include/opencv2/${name}/render/*.hpp"
|
|
"${CMAKE_CURRENT_LIST_DIR}/include/opencv2/${name}/streaming/*.hpp"
|
|
"${CMAKE_CURRENT_LIST_DIR}/include/opencv2/${name}/plaidml/*.hpp"
|
|
"${CMAKE_CURRENT_LIST_DIR}/include/opencv2/${name}/util/*.hpp"
|
|
)
|
|
|
|
set(gapi_srcs
|
|
# Front-end part
|
|
src/api/gorigin.cpp
|
|
src/api/gmat.cpp
|
|
src/api/garray.cpp
|
|
src/api/gopaque.cpp
|
|
src/api/gscalar.cpp
|
|
src/api/gkernel.cpp
|
|
src/api/gbackend.cpp
|
|
src/api/gproto.cpp
|
|
src/api/gnode.cpp
|
|
src/api/gcall.cpp
|
|
src/api/gcomputation.cpp
|
|
src/api/operators.cpp
|
|
src/api/kernels_core.cpp
|
|
src/api/kernels_imgproc.cpp
|
|
src/api/kernels_video.cpp
|
|
src/api/render.cpp
|
|
src/api/render_ocv.cpp
|
|
src/api/ginfer.cpp
|
|
src/api/ft_render.cpp
|
|
|
|
# Compiler part
|
|
src/compiler/gmodel.cpp
|
|
src/compiler/gmodelbuilder.cpp
|
|
src/compiler/gislandmodel.cpp
|
|
src/compiler/gcompiler.cpp
|
|
src/compiler/gcompiled.cpp
|
|
src/compiler/gstreaming.cpp
|
|
src/compiler/passes/helpers.cpp
|
|
src/compiler/passes/dump_dot.cpp
|
|
src/compiler/passes/islands.cpp
|
|
src/compiler/passes/meta.cpp
|
|
src/compiler/passes/kernels.cpp
|
|
src/compiler/passes/exec.cpp
|
|
src/compiler/passes/transformations.cpp
|
|
src/compiler/passes/pattern_matching.cpp
|
|
src/compiler/passes/perform_substitution.cpp
|
|
src/compiler/passes/streaming.cpp
|
|
|
|
# Executor
|
|
src/executor/gexecutor.cpp
|
|
src/executor/gstreamingexecutor.cpp
|
|
src/executor/gasync.cpp
|
|
|
|
# CPU Backend (currently built-in)
|
|
src/backends/cpu/gcpubackend.cpp
|
|
src/backends/cpu/gcpukernel.cpp
|
|
src/backends/cpu/gcpuimgproc.cpp
|
|
src/backends/cpu/gcpuvideo.cpp
|
|
src/backends/cpu/gcpucore.cpp
|
|
|
|
# Fluid Backend (also built-in, FIXME:move away)
|
|
src/backends/fluid/gfluidbuffer.cpp
|
|
src/backends/fluid/gfluidbackend.cpp
|
|
src/backends/fluid/gfluidimgproc.cpp
|
|
src/backends/fluid/gfluidimgproc_func.dispatch.cpp
|
|
src/backends/fluid/gfluidcore.cpp
|
|
|
|
# OCL Backend (currently built-in)
|
|
src/backends/ocl/goclbackend.cpp
|
|
src/backends/ocl/goclkernel.cpp
|
|
src/backends/ocl/goclimgproc.cpp
|
|
src/backends/ocl/goclcore.cpp
|
|
|
|
# IE Backend. FIXME: should be included by CMake
|
|
# if and only if IE support is enabled
|
|
src/backends/ie/giebackend.cpp
|
|
|
|
# Render Backend.
|
|
src/backends/render/grenderocvbackend.cpp
|
|
src/backends/render/grenderocv.cpp
|
|
|
|
#PlaidML Backend
|
|
src/backends/plaidml/gplaidmlcore.cpp
|
|
src/backends/plaidml/gplaidmlbackend.cpp
|
|
|
|
# Compound
|
|
src/backends/common/gcompoundbackend.cpp
|
|
src/backends/common/gcompoundkernel.cpp
|
|
|
|
# Serialization API and routines
|
|
src/api/s11n.cpp
|
|
src/backends/common/serialization.cpp
|
|
)
|
|
|
|
ocv_add_dispatched_file(backends/fluid/gfluidimgproc_func SSE4_1 AVX2)
|
|
|
|
ocv_list_add_prefix(gapi_srcs "${CMAKE_CURRENT_LIST_DIR}/")
|
|
|
|
# For IDE users
|
|
ocv_source_group("Src" FILES ${gapi_srcs})
|
|
ocv_source_group("Include" FILES ${gapi_ext_hdrs})
|
|
|
|
ocv_set_module_sources(HEADERS ${gapi_ext_hdrs} SOURCES ${gapi_srcs})
|
|
ocv_module_include_directories("${CMAKE_CURRENT_LIST_DIR}/src")
|
|
|
|
ocv_create_module()
|
|
|
|
ocv_target_link_libraries(${the_module} PRIVATE ade)
|
|
if(OPENCV_GAPI_INF_ENGINE)
|
|
ocv_target_link_libraries(${the_module} PRIVATE ${INF_ENGINE_TARGET})
|
|
endif()
|
|
if(HAVE_TBB)
|
|
ocv_target_link_libraries(${the_module} PRIVATE tbb)
|
|
endif()
|
|
|
|
set(__test_extra_deps "")
|
|
if(OPENCV_GAPI_INF_ENGINE)
|
|
list(APPEND __test_extra_deps ${INF_ENGINE_TARGET})
|
|
endif()
|
|
ocv_add_accuracy_tests(${__test_extra_deps})
|
|
|
|
# FIXME: test binary is linked with ADE directly since ADE symbols
|
|
# are not exported from libopencv_gapi.so in any form - thus
|
|
# there're two copies of ADE code in memory when tests run (!)
|
|
# src/ is specified to include dirs for INTERNAL tests only.
|
|
if(TARGET opencv_test_gapi)
|
|
target_include_directories(opencv_test_gapi PRIVATE "${CMAKE_CURRENT_LIST_DIR}/src")
|
|
target_link_libraries(opencv_test_gapi PRIVATE ade)
|
|
endif()
|
|
|
|
if(HAVE_FREETYPE)
|
|
ocv_target_compile_definitions(${the_module} PRIVATE -DHAVE_FREETYPE)
|
|
if(TARGET opencv_test_gapi)
|
|
ocv_target_compile_definitions(opencv_test_gapi PRIVATE -DHAVE_FREETYPE)
|
|
endif()
|
|
ocv_target_link_libraries(${the_module} PRIVATE ${FREETYPE_LIBRARIES})
|
|
ocv_target_include_directories(${the_module} PRIVATE ${FREETYPE_INCLUDE_DIRS})
|
|
endif()
|
|
|
|
if(HAVE_PLAIDML)
|
|
ocv_target_compile_definitions(${the_module} PRIVATE -DHAVE_PLAIDML)
|
|
if(TARGET opencv_test_gapi)
|
|
ocv_target_compile_definitions(opencv_test_gapi PRIVATE -DHAVE_PLAIDML)
|
|
endif()
|
|
ocv_target_link_libraries(${the_module} PRIVATE ${PLAIDML_LIBRARIES})
|
|
ocv_target_include_directories(${the_module} SYSTEM PRIVATE ${PLAIDML_INCLUDE_DIRS})
|
|
endif()
|
|
|
|
if(WIN32)
|
|
# Required for htonl/ntohl on Windows
|
|
target_link_libraries(${the_module} PRIVATE wsock32 ws2_32)
|
|
endif()
|
|
|
|
ocv_add_perf_tests()
|
|
ocv_add_samples()
|