mirror of
https://github.com/opencv/opencv.git
synced 2025-01-10 14:19:03 +08:00
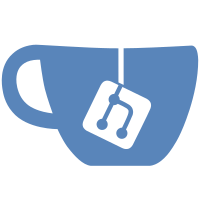
videoio: add Orbbec Gemini 2 and Astra 2 camera support ### Test Result | OS | Compiler | Camera | Result | |-----|-----------|---------|--------| |Windows11| (VS2022)MSVC17.3|Orbbec Gemini 2|Pass| |Windows11| (VS2022)MSVC17.3|Orbbec Astra 2|Pass| |Ubuntu22.04|GCC9.2|Orbbec Gemini 2|Pass| |Ubuntu22.04|GCC9.2|Orbbec Astra 2|Pass| ### Pull Request Readiness Checklist - [x] I agree to contribute to the project under Apache 2 License. - [x] To the best of my knowledge, the proposed patch is not based on a code under GPL or another license that is incompatible with OpenCV - [x] The PR is proposed to the proper branch - [x] The feature is well documented and sample code can be built with the project CMake
69 lines
2.0 KiB
C++
69 lines
2.0 KiB
C++
// This file is part of OpenCV project.
|
|
// It is subject to the license terms in the LICENSE file found in the top-level directory
|
|
// of this distribution and at http://opencv.org/license.html.
|
|
|
|
/*
|
|
* Copyright(C) 2022 by ORBBEC Technology., Inc.
|
|
* Authors:
|
|
* Huang Zhenchang <yufeng@orbbec.com>
|
|
*
|
|
* Licensed under the Apache License, Version 2.0 (the "License");
|
|
* you may not use this file except in compliance with the License.
|
|
* You may obtain a copy of the License at
|
|
* http://www.apache.org/licenses/LICENSE-2.0
|
|
*
|
|
* Unless required by applicable law or agreed to in writing, software
|
|
* distributed under the License is distributed on an "AS IS" BASIS,
|
|
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
* See the License for the specific language governing permissions and
|
|
* limitations under the License.
|
|
*/
|
|
|
|
#ifndef OPENCV_VIDEOIO_CAP_OBSENSOR_CAPTURE_HPP
|
|
#define OPENCV_VIDEOIO_CAP_OBSENSOR_CAPTURE_HPP
|
|
|
|
#include <map>
|
|
#include <mutex>
|
|
#include <condition_variable>
|
|
|
|
#include "cap_obsensor/obsensor_stream_channel_interface.hpp"
|
|
|
|
#ifdef HAVE_OBSENSOR
|
|
namespace cv {
|
|
class VideoCapture_obsensor : public IVideoCapture
|
|
{
|
|
public:
|
|
VideoCapture_obsensor(int index);
|
|
virtual ~VideoCapture_obsensor();
|
|
|
|
virtual double getProperty(int propIdx) const CV_OVERRIDE;
|
|
virtual bool setProperty(int propIdx, double propVal) CV_OVERRIDE;
|
|
virtual bool grabFrame() CV_OVERRIDE;
|
|
virtual bool retrieveFrame(int outputType, OutputArray frame) CV_OVERRIDE;
|
|
virtual int getCaptureDomain() CV_OVERRIDE {
|
|
return CAP_OBSENSOR;
|
|
}
|
|
virtual bool isOpened() const CV_OVERRIDE {
|
|
return isOpened_;
|
|
}
|
|
|
|
private:
|
|
bool isOpened_;
|
|
std::vector<Ptr<obsensor::IStreamChannel>> streamChannelGroup_;
|
|
|
|
std::mutex frameMutex_;
|
|
std::condition_variable frameCv_;
|
|
|
|
Mat depthFrame_;
|
|
Mat colorFrame_;
|
|
|
|
Mat grabbedDepthFrame_;
|
|
Mat grabbedColorFrame_;
|
|
|
|
obsensor::CameraParam camParam_;
|
|
int camParamScale_;
|
|
};
|
|
} // namespace cv::
|
|
#endif // HAVE_OBSENSOR
|
|
#endif // OPENCV_VIDEOIO_CAP_OBSENSOR_CAPTURE_HPP
|