mirror of
https://github.com/opencv/opencv.git
synced 2024-11-25 19:50:38 +08:00
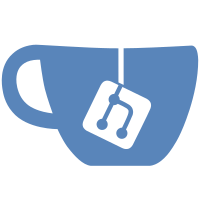
V4L (V4L2): Refactoring. Added missed camera properties. Fixed getting `INF` for some properties. Singlethread as always (#12893) * cap_v4l: 1 Added cap_properties verbalization. 2 Set Get of properties elementary refactoring. 3 Removed converting parameters to/from [0,1] range. 4 Added all known conversion from V4L2_CID_* to CV_CAP_PROP_* * cap_v4l: 1. Removed all query for parameters range. 2. Refactored capture initialization. 3. Added selecting input channel by CV_CAP_PROP_MODE. Default value -1 the channels not changed. * cap_v4l: 1. Refactoring of Convert To RGB * cap_v4l: 1. Fixed use of video buffer index. 2. Removed extra memcopy for grab image. 3. Removed device closing from autosetup_capture_mode_v4l2 * cap_v4l: 1. The `goto` was eliminated 2. Fixed use of temporary buffer index for V4L2_PIX_FMT_SN9C10X 3. Fixed use of the bufferIndex 4. Removed trailing spaces and unused variables. * cap_v4l: 1. Alias for capture->buffers[capture->bufferIndex] 2. Reduced size of data for memcpy: bytesused instead of length 3. Refactoring. Code duplication. More info for debug * cap_v4l: 1. Added the ability to grab and retrieveFrame independently several times * cap_v4l: 1. Not need to close/open device for new capture parameters applying. 2. Removed using of device name as a flag that the capture is closed. Added sufficient function. 3. Refactoring. Added requestBuffers and createBuffers * cap_v4l: 1. Added tryIoctl with `select` like was in mainloop_v4l2. 2. Fixed buffer request for device without closing the device. 3. Some static function moved to CvCaptureCAM_V4L 4. Removed unused defines * cap_v4l: 1. Thread-safe now * cap_v4l: 1. Fixed thread-safe destructor 2. Fixed FPS setting * Missed brake * Removed thread-safety * cap_v4l: 1. Reverted conversion parameters to/from [0,1] by default for backward compatibility. 2. Added setting for turn off compatibility mode: set CV_CAP_PROP_MODE to 65536 3. Most static functions moved to CvCaptureCAM_V4L 4. Refactoring of icvRetrieveFrameCAM_V4L and using of frame_allocated flag * cap_v4l: 1. Added conversion to RGB from NV12, NV21 2. Refactoring. Removed wrappers for known format conversions. * Added `CAP_PROP_CHANNEL` to the enum VideoCaptureProperties. CAP_V4L migrated to use VideoCaptureProperties. * 1. Update comments. 2. Environment variable `OPENCV_VIDEOIO_V4L_RANGE_NORMALIZED` for setting default backward compatibility mode. 3. Revert getting of `CAP_PROP_MODE` as fourcc code in backward compatibility mode. * videoio: update cap_v4l - compatibilityMode => normalizePropRange * videoio(test): V4L2 MJPEG test `v4l2-ctl --list-formats` should have 'MJPG' entry * videoio: fix buffer initialization to avoid "munmap: Invalid argument" messages
59 lines
2.3 KiB
C++
59 lines
2.3 KiB
C++
// This file is part of OpenCV project.
|
|
// It is subject to the license terms in the LICENSE file found in the top-level directory
|
|
// of this distribution and at http://opencv.org/license.html.
|
|
|
|
// Note: all tests here are DISABLED by default due specific requirements.
|
|
// Don't use #if 0 - these tests should be tested for compilation at least.
|
|
//
|
|
// Usage: opencv_test_videoio --gtest_also_run_disabled_tests --gtest_filter=*VideoIO_Camera*<tested case>*
|
|
|
|
#include "test_precomp.hpp"
|
|
|
|
namespace opencv_test { namespace {
|
|
|
|
static void test_readFrames(/*const*/ VideoCapture& capture, const int N = 100)
|
|
{
|
|
Mat frame;
|
|
int64 time0 = cv::getTickCount();
|
|
for (int i = 0; i < N; i++)
|
|
{
|
|
SCOPED_TRACE(cv::format("frame=%d", i));
|
|
|
|
capture >> frame;
|
|
ASSERT_FALSE(frame.empty());
|
|
|
|
EXPECT_GT(cvtest::norm(frame, NORM_INF), 0) << "Complete black image has been received";
|
|
}
|
|
int64 time1 = cv::getTickCount();
|
|
printf("Processed %d frames on %.2f FPS\n", N, (N * cv::getTickFrequency()) / (time1 - time0 + 1));
|
|
}
|
|
|
|
TEST(DISABLED_VideoIO_Camera, basic)
|
|
{
|
|
VideoCapture capture(0);
|
|
ASSERT_TRUE(capture.isOpened());
|
|
std::cout << "Camera 0 via " << capture.getBackendName() << " backend" << std::endl;
|
|
std::cout << "Frame width: " << capture.get(CAP_PROP_FRAME_WIDTH) << std::endl;
|
|
std::cout << " height: " << capture.get(CAP_PROP_FRAME_HEIGHT) << std::endl;
|
|
std::cout << "Capturing FPS: " << capture.get(CAP_PROP_FPS) << std::endl;
|
|
test_readFrames(capture);
|
|
capture.release();
|
|
}
|
|
|
|
TEST(DISABLED_VideoIO_Camera, validate_V4L2_MJPEG)
|
|
{
|
|
VideoCapture capture(CAP_V4L2);
|
|
ASSERT_TRUE(capture.isOpened());
|
|
ASSERT_TRUE(capture.set(CAP_PROP_FOURCC, VideoWriter::fourcc('M', 'J', 'P', 'G')));
|
|
std::cout << "Camera 0 via " << capture.getBackendName() << " backend" << std::endl;
|
|
std::cout << "Frame width: " << capture.get(CAP_PROP_FRAME_WIDTH) << std::endl;
|
|
std::cout << " height: " << capture.get(CAP_PROP_FRAME_HEIGHT) << std::endl;
|
|
std::cout << "Capturing FPS: " << capture.get(CAP_PROP_FPS) << std::endl;
|
|
int fourcc = (int)capture.get(CAP_PROP_FOURCC);
|
|
std::cout << "FOURCC code: " << cv::format("0x%8x", fourcc) << std::endl;
|
|
test_readFrames(capture);
|
|
capture.release();
|
|
}
|
|
|
|
}} // namespace
|