mirror of
https://github.com/opencv/opencv.git
synced 2024-12-05 01:39:13 +08:00
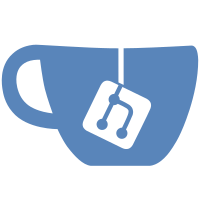
core: persistence: output reals as human-friendly expression. #25351 Close #25073 Related https://github.com/opencv/opencv/pull/25087 This patch is need to merge same time with https://github.com/opencv/opencv_contrib/pull/3714 ### Pull Request Readiness Checklist See details at https://github.com/opencv/opencv/wiki/How_to_contribute#making-a-good-pull-request - [x] I agree to contribute to the project under Apache 2 License. - [x] To the best of my knowledge, the proposed patch is not based on a code under GPL or another license that is incompatible with OpenCV - [x] The PR is proposed to the proper branch - [x] There is a reference to the original bug report and related work - [x] There is accuracy test, performance test and test data in opencv_extra repository, if applicable Patch to opencv_extra has the same branch name. - [x] The feature is well documented and sample code can be built with the project CMake
68 lines
2.2 KiB
Java
68 lines
2.2 KiB
Java
package org.opencv.test.features2d;
|
|
|
|
import org.opencv.test.OpenCVTestCase;
|
|
import org.opencv.test.OpenCVTestRunner;
|
|
import org.opencv.features2d.GFTTDetector;
|
|
|
|
public class GFTTFeatureDetectorTest extends OpenCVTestCase {
|
|
|
|
GFTTDetector detector;
|
|
|
|
@Override
|
|
protected void setUp() throws Exception {
|
|
super.setUp();
|
|
detector = GFTTDetector.create(); // default constructor have (1000, 0.01, 1, 3, 3, false, 0.04)
|
|
}
|
|
|
|
public void testCreate() {
|
|
assertNotNull(detector);
|
|
}
|
|
|
|
public void testDetectListOfMatListOfListOfKeyPoint() {
|
|
fail("Not yet implemented");
|
|
}
|
|
|
|
public void testDetectListOfMatListOfListOfKeyPointListOfMat() {
|
|
fail("Not yet implemented");
|
|
}
|
|
|
|
public void testDetectMatListOfKeyPoint() {
|
|
fail("Not yet implemented");
|
|
}
|
|
|
|
public void testDetectMatListOfKeyPointMat() {
|
|
fail("Not yet implemented");
|
|
}
|
|
|
|
public void testEmpty() {
|
|
fail("Not yet implemented");
|
|
}
|
|
|
|
public void testReadYml() {
|
|
String filename = OpenCVTestRunner.getTempFileName("yml");
|
|
|
|
writeFile(filename, "%YAML:1.0\n---\nname: \"Feature2D.GFTTDetector\"\nnfeatures: 500\nqualityLevel: 2.0000000000000000e-02\nminDistance: 2.\nblockSize: 4\ngradSize: 5\nuseHarrisDetector: 1\nk: 5.0000000000000000e-02\n");
|
|
detector.read(filename);
|
|
|
|
assertEquals(500, detector.getMaxFeatures());
|
|
assertEquals(0.02, detector.getQualityLevel());
|
|
assertEquals(2.0, detector.getMinDistance());
|
|
assertEquals(4, detector.getBlockSize());
|
|
assertEquals(5, detector.getGradientSize());
|
|
assertEquals(true, detector.getHarrisDetector());
|
|
assertEquals(0.05, detector.getK());
|
|
}
|
|
|
|
public void testWriteYml() {
|
|
String filename = OpenCVTestRunner.getTempFileName("yml");
|
|
|
|
detector.write(filename);
|
|
|
|
String truth = "%YAML:1.0\n---\nname: \"Feature2D.GFTTDetector\"\nnfeatures: 1000\nqualityLevel: 0.01\nminDistance: 1.\nblockSize: 3\ngradSize: 3\nuseHarrisDetector: 0\nk: 0.040000000000000001\n";
|
|
String actual = readFile(filename);
|
|
actual = actual.replaceAll("e([+-])0(\\d\\d)", "e$1$2"); // NOTE: workaround for different platforms double representation
|
|
assertEquals(truth, actual);
|
|
}
|
|
|
|
}
|