mirror of
https://github.com/opencv/opencv.git
synced 2024-11-24 11:10:21 +08:00
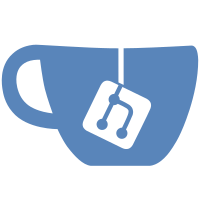
[GSoC] OpenCV.js: WASM SIMD optimization 2.0 * gsoc_2020_simd Add perf test for filter2d * add perf test for kernel scharr and kernel gaussianBlur * add perf test for blur, medianBlur, erode, dilate * fix the errors for the opencv PR robot fix the trailing whitespace. * add perf tests for kernel remap, warpAffine, warpPersepective, pyrDown * fix a bug in modules/js/perf/perf_imgproc/perf_remap.js * add function smoothBorder in helpfun.js and remove replicated function in perf test of warpAffine and warpPrespective * fix the trailing white space issues * add OpenCV.js loader * Implement the Loader with help of WebAssembly Feature Detection, remove trailing whitespaces * modify the explantion for loader in js_setup.markdown and fix bug in loader.js
117 lines
3.7 KiB
JavaScript
117 lines
3.7 KiB
JavaScript
const isNodeJs = (typeof window) === 'undefined'? true : false;
|
||
|
||
if (isNodeJs) {
|
||
var Benchmark = require('benchmark');
|
||
var cv = require('../../opencv');
|
||
var HelpFunc = require('../perf_helpfunc');
|
||
var Base = require('../base');
|
||
} else {
|
||
var paramsElement = document.getElementById('params');
|
||
var runButton = document.getElementById('runButton');
|
||
var logElement = document.getElementById('log');
|
||
}
|
||
|
||
function perf() {
|
||
|
||
console.log('opencv.js loaded');
|
||
if (isNodeJs) {
|
||
global.cv = cv;
|
||
global.combine = HelpFunc.combine;
|
||
global.cvtStr2cvSize = HelpFunc.cvtStr2cvSize;
|
||
global.cvSize = Base.getCvSize();
|
||
} else {
|
||
enableButton();
|
||
cvSize = getCvSize();
|
||
}
|
||
let totalCaseNum, currentCaseId;
|
||
|
||
const ErodeSize = [cvSize.szQVGA, cvSize.szVGA, cvSize.szSVGA, cvSize.szXGA, cvSize.szSXGA];
|
||
const ErodeType = ["CV_8UC1", "CV_8UC4"];
|
||
const combiErode = combine(ErodeSize, ErodeType);
|
||
|
||
function addErodeCase(suite, type) {
|
||
suite.add('erode', function() {
|
||
cv.erode(src, dst, kernel);
|
||
}, {
|
||
'setup': function() {
|
||
let size = this.params.size;
|
||
let matType = cv[this.params.matType];
|
||
let src = new cv.Mat(size, matType);
|
||
let dst = new cv.Mat(size, matType);
|
||
let kernel = new cv.Mat();
|
||
},
|
||
'teardown': function() {
|
||
src.delete();
|
||
dst.delete();
|
||
kernel.delete();
|
||
}
|
||
});
|
||
}
|
||
|
||
function addErodeModeCase(suite, combination, type) {
|
||
totalCaseNum += combination.length;
|
||
for (let i = 0; i < combination.length; ++i) {
|
||
let size = combination[i][0];
|
||
let matType = combination[i][1];
|
||
|
||
let params = {size: size, matType:matType};
|
||
addKernelCase(suite, params, type, addErodeCase);
|
||
}
|
||
}
|
||
|
||
function genBenchmarkCase(paramsContent) {
|
||
let suite = new Benchmark.Suite;
|
||
totalCaseNum = 0;
|
||
currentCaseId = 0;
|
||
|
||
if (/\([0-9]+x[0-9]+,[\ ]*CV\_\w+\)/g.test(paramsContent.toString())) {
|
||
let params = paramsContent.toString().match(/\([0-9]+x[0-9]+,[\ ]*CV\_\w+\)/g)[0];
|
||
let paramObjs = [];
|
||
paramObjs.push({name:"size", value:"", reg:[""], index:0});
|
||
paramObjs.push({name:"matType", value:"", reg:["/CV\_[0-9]+[FSUfsu]C[0-9]/"], index:1});
|
||
let locationList = decodeParams2Case(params, paramObjs, erodeCombinations);
|
||
|
||
for (let i = 0; i < locationList.length; i++){
|
||
let first = locationList[i][0];
|
||
let second = locationList[i][1];
|
||
addErodeModeCase(suite, [erodeCombinations[first][second]], first);
|
||
}
|
||
} else {
|
||
log("no filter or getting invalid params, run all the cases");
|
||
addErodeModeCase(suite, combiErode, 0);
|
||
}
|
||
setBenchmarkSuite(suite, "erode", currentCaseId);
|
||
log(`Running ${totalCaseNum} tests from erode`);
|
||
suite.run({ 'async': true }); // run the benchmark
|
||
}
|
||
|
||
let erodeCombinations = [combiErode];
|
||
|
||
if (isNodeJs) {
|
||
const args = process.argv.slice(2);
|
||
let paramsContent = '';
|
||
if (/--test_param_filter=\([0-9]+x[0-9]+,[\ ]*CV\_\w+\)/g.test(args.toString())) {
|
||
paramsContent = args.toString().match(/\([0-9]+x[0-9]+,[\ ]*CV\_\w+\)/g)[0];
|
||
}
|
||
genBenchmarkCase(paramsContent);
|
||
} else {
|
||
runButton.onclick = function() {
|
||
let paramsContent = paramsElement.value;
|
||
genBenchmarkCase(paramsContent);
|
||
if (totalCaseNum !== 0) {
|
||
disableButton();
|
||
}
|
||
}
|
||
}
|
||
};
|
||
|
||
async function main() {
|
||
if (cv instanceof Promise) {
|
||
cv = await cv;
|
||
perf();
|
||
} else {
|
||
cv.onRuntimeInitialized = perf;
|
||
}
|
||
}
|
||
|
||
main(); |