mirror of
https://github.com/opencv/opencv.git
synced 2024-12-05 01:39:13 +08:00
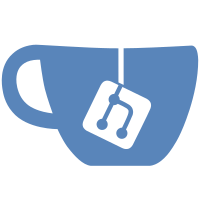
Added clapack * bring a small subset of Lapack, automatically converted to C, into OpenCV * added missing lsame_ prototype * * small fix in make_clapack script * trying to fix remaining CI problems * fixed character arrays' initializers * get rid of F2C_STR_MAX * * added back single-precision versions for QR, LU and Cholesky decompositions. It adds very little extra overhead. * added stub version of sdesdd. * uncommented calls to all the single-precision Lapack functions from opencv/core/src/hal_internal.cpp. * fixed warning from Visual Studio + cleaned f2c runtime a bit * * regenerated Lapack w/o forward declarations of intrinsic functions (such as sqrt(), r_cnjg() etc.) * at once, trailing whitespaces are removed from the generated sources, just in case * since there is no declarations of intrinsic functions anymore, we could turn some of them into inline functions * trying to eliminate the crash on ARM * fixed API and semantics of s_copy * * CLapack has been tested successfully. It's now time to restore the standard LAPACK detection procedure * removed some more trailing whitespaces * * retained only the essential stuff in CLapack * added checks to lapack calls to gracefully return "not implemented" instead of returning invalid results with "ok" status * disabled warning when building lapack * cmake: update LAPACK detection Co-authored-by: Alexander Alekhin <alexander.a.alekhin@gmail.com>
97 lines
2.1 KiB
C
97 lines
2.1 KiB
C
#include "f2c.h"
|
|
|
|
static const int CLAPACK_NOT_IMPLEMENTED = -1024;
|
|
|
|
int sgesdd_(char *jobz, int *m, int *n, float *a, int *lda,
|
|
float *s, float *u, int *ldu, float *vt, int *ldvt, float *work,
|
|
int *lwork, int *iwork, int *info)
|
|
{
|
|
*info = CLAPACK_NOT_IMPLEMENTED;
|
|
return 0;
|
|
}
|
|
|
|
int dgels_(char *trans, int *m, int *n, int *nrhs, double *a,
|
|
int *lda, double *b, int *ldb, double *work, int *lwork, int *info)
|
|
{
|
|
*info = CLAPACK_NOT_IMPLEMENTED;
|
|
return 0;
|
|
}
|
|
|
|
int dgesv_(int *n, int *nrhs, double *a, int *lda, int *ipiv,
|
|
double *b, int *ldb, int *info)
|
|
{
|
|
*info = CLAPACK_NOT_IMPLEMENTED;
|
|
return 0;
|
|
}
|
|
|
|
int dgetrf_(int *m, int *n, double *a, int *lda, int *ipiv,
|
|
int *info)
|
|
{
|
|
*info = CLAPACK_NOT_IMPLEMENTED;
|
|
return 0;
|
|
}
|
|
|
|
int dposv_(char *uplo, int *n, int *nrhs, double *a, int *
|
|
lda, double *b, int *ldb, int *info)
|
|
{
|
|
*info = CLAPACK_NOT_IMPLEMENTED;
|
|
return 0;
|
|
}
|
|
|
|
int dpotrf_(char *uplo, int *n, double *a, int *lda, int *
|
|
info)
|
|
{
|
|
*info = CLAPACK_NOT_IMPLEMENTED;
|
|
return 0;
|
|
}
|
|
|
|
int sgels_(char *trans, int *m, int *n, int *nrhs, float *a,
|
|
int *lda, float *b, int *ldb, float *work, int *lwork, int *info)
|
|
{
|
|
*info = CLAPACK_NOT_IMPLEMENTED;
|
|
return 0;
|
|
}
|
|
|
|
int sgeev_(char *jobvl, char *jobvr, int *n, float *a, int *
|
|
lda, float *wr, float *wi, float *vl, int *ldvl, float *vr, int *
|
|
ldvr, float *work, int *lwork, int *info)
|
|
{
|
|
*info = CLAPACK_NOT_IMPLEMENTED;
|
|
return 0;
|
|
}
|
|
|
|
int sgeqrf_(int *m, int *n, float *a, int *lda, float *tau,
|
|
float *work, int *lwork, int *info)
|
|
{
|
|
*info = CLAPACK_NOT_IMPLEMENTED;
|
|
return 0;
|
|
}
|
|
|
|
int sgesv_(int *n, int *nrhs, float *a, int *lda, int *ipiv,
|
|
float *b, int *ldb, int *info)
|
|
{
|
|
*info = CLAPACK_NOT_IMPLEMENTED;
|
|
return 0;
|
|
}
|
|
|
|
int sgetrf_(int *m, int *n, float *a, int *lda, int *ipiv,
|
|
int *info)
|
|
{
|
|
*info = CLAPACK_NOT_IMPLEMENTED;
|
|
return 0;
|
|
}
|
|
|
|
int sposv_(char *uplo, int *n, int *nrhs, float *a, int *
|
|
lda, float *b, int *ldb, int *info)
|
|
{
|
|
*info = CLAPACK_NOT_IMPLEMENTED;
|
|
return 0;
|
|
}
|
|
|
|
int spotrf_(char *uplo, int *n, float *a, int *lda, int *
|
|
info)
|
|
{
|
|
*info = CLAPACK_NOT_IMPLEMENTED;
|
|
return 0;
|
|
}
|