mirror of
https://github.com/rustdesk/rustdesk.git
synced 2024-11-24 04:12:20 +08:00
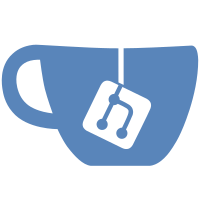
* add http(s) proxy
* Add front-end translation
* fix ui description
* For linux platform, add rustls support
* fix: Fix the proxy address test function.
* add: Added default prompts for agency agreement and some multi-language translations
* add: Http proxy request client
* fix: add async http proxy func and format the code
* add: Preliminary support for flutter front-end calling rust back-end http request
* Optimize HTTP calls
* Optimize HTTP calls
* fix: Optimize HTTP requests, refine translations, and fix dependencies
* fix: Win and macOS compilation errors
* fix: web platforms
* fix: Optimize import
* fix: Fix web platform issues
* fix: Fix web platform issues
* fix: update ci
* fix: test ci
* test: test CI
* Revert "fix: update ci"
This reverts commit 2e5f247b2e
.
* test: test CI
* test: test CI
* fix: fix lock file
* fix: Optimize imports
116 lines
3.5 KiB
Dart
116 lines
3.5 KiB
Dart
import 'dart:convert';
|
|
import 'package:flutter/foundation.dart';
|
|
import 'package:http/http.dart' as http;
|
|
import '../models/platform_model.dart';
|
|
export 'package:http/http.dart' show Response;
|
|
|
|
enum HttpMethod { get, post, put, delete }
|
|
|
|
class HttpService {
|
|
Future<http.Response> sendRequest(
|
|
Uri url,
|
|
HttpMethod method, {
|
|
Map<String, String>? headers,
|
|
dynamic body,
|
|
}) async {
|
|
headers ??= {'Content-Type': 'application/json'};
|
|
|
|
// Determine if there is currently a proxy setting, and if so, use FFI to call the Rust HTTP method.
|
|
final isProxy = await bind.mainGetProxyStatus();
|
|
|
|
if (!isProxy) {
|
|
return await _pollFultterHttp(url, method, headers: headers, body: body);
|
|
}
|
|
|
|
String headersJson = jsonEncode(headers);
|
|
String methodName = method.toString().split('.').last;
|
|
await bind.mainHttpRequest(
|
|
url: url.toString(),
|
|
method: methodName.toLowerCase(),
|
|
body: body,
|
|
header: headersJson);
|
|
|
|
var resJson = await _pollForResponse(url.toString());
|
|
return _parseHttpResponse(resJson);
|
|
}
|
|
|
|
Future<http.Response> _pollFultterHttp(
|
|
Uri url,
|
|
HttpMethod method, {
|
|
Map<String, String>? headers,
|
|
dynamic body,
|
|
}) async {
|
|
var response = http.Response('', 400);
|
|
|
|
switch (method) {
|
|
case HttpMethod.get:
|
|
response = await http.get(url, headers: headers);
|
|
break;
|
|
case HttpMethod.post:
|
|
response = await http.post(url, headers: headers, body: body);
|
|
break;
|
|
case HttpMethod.put:
|
|
response = await http.put(url, headers: headers, body: body);
|
|
break;
|
|
case HttpMethod.delete:
|
|
response = await http.delete(url, headers: headers, body: body);
|
|
break;
|
|
default:
|
|
throw Exception('Unsupported HTTP method');
|
|
}
|
|
|
|
return response;
|
|
}
|
|
|
|
Future<String> _pollForResponse(String url) async {
|
|
String? responseJson = " ";
|
|
while (responseJson == " ") {
|
|
responseJson = await bind.mainGetHttpStatus(url: url);
|
|
if (responseJson == null) {
|
|
throw Exception('The HTTP request failed');
|
|
}
|
|
if (responseJson == " ") {
|
|
await Future.delayed(const Duration(milliseconds: 100));
|
|
}
|
|
}
|
|
return responseJson!;
|
|
}
|
|
|
|
http.Response _parseHttpResponse(String responseJson) {
|
|
try {
|
|
var parsedJson = jsonDecode(responseJson);
|
|
String body = parsedJson['body'];
|
|
Map<String, String> headers = {};
|
|
for (var key in parsedJson['headers'].keys) {
|
|
headers[key] = parsedJson['headers'][key];
|
|
}
|
|
int statusCode = parsedJson['status_code'];
|
|
return http.Response(body, statusCode, headers: headers);
|
|
} catch (e) {
|
|
throw Exception('Failed to parse response: $e');
|
|
}
|
|
}
|
|
}
|
|
|
|
Future<http.Response> get(Uri url, {Map<String, String>? headers}) async {
|
|
return await HttpService().sendRequest(url, HttpMethod.get, headers: headers);
|
|
}
|
|
|
|
Future<http.Response> post(Uri url,
|
|
{Map<String, String>? headers, Object? body, Encoding? encoding}) async {
|
|
return await HttpService()
|
|
.sendRequest(url, HttpMethod.post, body: body, headers: headers);
|
|
}
|
|
|
|
Future<http.Response> put(Uri url,
|
|
{Map<String, String>? headers, Object? body, Encoding? encoding}) async {
|
|
return await HttpService()
|
|
.sendRequest(url, HttpMethod.put, body: body, headers: headers);
|
|
}
|
|
|
|
Future<http.Response> delete(Uri url,
|
|
{Map<String, String>? headers, Object? body, Encoding? encoding}) async {
|
|
return await HttpService()
|
|
.sendRequest(url, HttpMethod.delete, body: body, headers: headers);
|
|
}
|