mirror of
https://github.com/ueberdosis/tiptap.git
synced 2024-12-19 22:27:52 +08:00
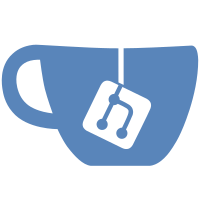
* chore:(core): migrate to tsup * chore: migrate blockquote and bold to tsup * chore: migrated bubble-menu and bullet-list to tsup * chore: migrated more packages to tsup * chore: migrate code and character extensions to tsup * chore: update package.json to simplify build for all packages * chore: move all packages to tsup as a build process * chore: change ci build task * feat(pm): add prosemirror meta package * rfix: resolve issues with build paths & export mappings * docs: update documentation to include notes for @tiptap/pm * chore(pm): update tsconfig * chore(packages): update packages * fix(pm): add package export infos & fix dependencies * chore(general): start moving to pm package as deps * chore: move to tiptap pm package internally * fix(demos): fix demos working with new pm package * fix(tables): fix tables package * fix(tables): fix tables package * chore(demos): pinned typescript version * chore: remove unnecessary tsconfig * chore: fix netlify build * fix(demos): fix package resolving for pm packages * fix(tests): fix package resolving for pm packages * fix(tests): fix package resolving for pm packages * chore(tests): fix tests not running correctly after pm package * chore(pm): add files to files array * chore: update build workflow * chore(tests): increase timeout time back to 12s * chore(docs): update docs * chore(docs): update installation guides & pm information to docs * chore(docs): add link to prosemirror docs * fix(vue-3): add missing build step * chore(docs): comment out cdn link * chore(docs): remove semicolons from docs * chore(docs): remove unnecessary installation note * chore(docs): remove unnecessary installation note
171 lines
4.6 KiB
TypeScript
171 lines
4.6 KiB
TypeScript
/// <reference types="cypress" />
|
|
|
|
import { Editor } from '@tiptap/core'
|
|
import Bold from '@tiptap/extension-bold'
|
|
import Code from '@tiptap/extension-code'
|
|
import CodeBlock from '@tiptap/extension-code-block'
|
|
import Document from '@tiptap/extension-document'
|
|
import History from '@tiptap/extension-history'
|
|
import Paragraph from '@tiptap/extension-paragraph'
|
|
import Text from '@tiptap/extension-text'
|
|
|
|
describe('can', () => {
|
|
it('not undo', () => {
|
|
const editor = new Editor({
|
|
extensions: [Document, Paragraph, Text, History],
|
|
})
|
|
|
|
const canUndo = editor.can().undo()
|
|
|
|
expect(canUndo).to.eq(false)
|
|
})
|
|
|
|
it('undo', () => {
|
|
const editor = new Editor({
|
|
extensions: [Document, Paragraph, Text, History],
|
|
})
|
|
|
|
editor.commands.setContent('foo')
|
|
|
|
const canUndo = editor.can().undo()
|
|
|
|
expect(canUndo).to.eq(true)
|
|
})
|
|
|
|
it('not chain undo', () => {
|
|
const editor = new Editor({
|
|
extensions: [Document, Paragraph, Text, History],
|
|
})
|
|
|
|
const canUndo = editor.can().chain().undo().run()
|
|
|
|
expect(canUndo).to.eq(false)
|
|
})
|
|
|
|
it('chain undo', () => {
|
|
const editor = new Editor({
|
|
extensions: [Document, Paragraph, Text, History],
|
|
})
|
|
|
|
editor.commands.setContent('foo')
|
|
|
|
const canUndo = editor.can().chain().undo().run()
|
|
|
|
expect(canUndo).to.eq(true)
|
|
})
|
|
|
|
it('returns false for non-applicable marks when selection contains node in conflict', () => {
|
|
const editor = new Editor({
|
|
extensions: [Document, Paragraph, Text, History, CodeBlock, Bold],
|
|
})
|
|
|
|
editor
|
|
.chain()
|
|
.setCodeBlock()
|
|
.insertContent('Test code block')
|
|
.setTextSelection({ from: 2, to: 3 })
|
|
.selectAll()
|
|
.run()
|
|
|
|
const canSetMarkToBold = editor.can().setMark('bold')
|
|
|
|
expect(canSetMarkToBold).to.eq(false)
|
|
})
|
|
|
|
it('returns false for non-applicable marks when selection contains marks in conflict', () => {
|
|
const editor = new Editor({
|
|
extensions: [Document, Paragraph, Text, History, Code, Bold],
|
|
})
|
|
|
|
editor.chain().setContent('<code>test</code>').setTextSelection({ from: 2, to: 3 }).run()
|
|
|
|
const canSetMarkToBold = editor.can().setMark('bold')
|
|
|
|
expect(canSetMarkToBold).to.eq(false)
|
|
})
|
|
|
|
it('returns false for non-applicable marks when stored marks in conflict', () => {
|
|
const editor = new Editor({
|
|
extensions: [Document, Paragraph, Text, History, Code, Bold],
|
|
})
|
|
|
|
editor.chain().setContent('<code>test</code>').run()
|
|
|
|
const canSetMarkToBold = editor.can().setMark('bold')
|
|
|
|
expect(canSetMarkToBold).to.eq(false)
|
|
})
|
|
|
|
it('returns false for non-applicable marks when selecting multiple nodes in conflict', () => {
|
|
const editor = new Editor({
|
|
extensions: [Document, Paragraph, Text, History, Code, Bold],
|
|
})
|
|
|
|
editor.chain().setContent('<code>test</code><code>123</code>').selectAll().run()
|
|
|
|
const canSetMarkToBold = editor.can().setMark('bold')
|
|
|
|
expect(canSetMarkToBold).to.eq(false)
|
|
})
|
|
|
|
it('returns true for applicable marks when selection does not contain nodes in conflict', () => {
|
|
const editor = new Editor({
|
|
extensions: [Document, Paragraph, Text, History, CodeBlock, Bold],
|
|
})
|
|
|
|
editor
|
|
.chain()
|
|
.setCodeBlock()
|
|
.insertContent('Test code block')
|
|
.exitCode()
|
|
.insertContent('Additional paragraph node')
|
|
.selectAll()
|
|
.run()
|
|
|
|
const canSetMarkToBold = editor.can().setMark('bold')
|
|
|
|
expect(canSetMarkToBold).to.eq(true)
|
|
})
|
|
|
|
it('returns true for applicable marks when stored marks are not in conflict', () => {
|
|
const editor = new Editor({
|
|
extensions: [Document, Paragraph, Text, History, Code, Bold],
|
|
})
|
|
|
|
editor.chain().setContent('<code>test</code>').toggleCode().run()
|
|
|
|
const canSetMarkToBold = editor.can().setMark('bold')
|
|
|
|
expect(canSetMarkToBold).to.eq(true)
|
|
})
|
|
|
|
it('returns true for applicable marks when selection does not contain marks in conflict', () => {
|
|
const editor = new Editor({
|
|
extensions: [Document, Paragraph, Text, History, Code, Bold],
|
|
})
|
|
|
|
editor
|
|
.chain()
|
|
.setContent('<code>test</code>')
|
|
.setTextSelection({ from: 2, to: 3 })
|
|
.toggleCode()
|
|
.run()
|
|
|
|
const canSetMarkToBold = editor.can().setMark('bold')
|
|
|
|
expect(canSetMarkToBold).to.eq(true)
|
|
})
|
|
|
|
it('returns true for applicable marks if at least one node in selection has no marks in conflict', () => {
|
|
const editor = new Editor({
|
|
extensions: [Document, Paragraph, Text, History, Code, Bold],
|
|
})
|
|
|
|
editor.chain().setContent('<code>test</code><i>123</i>').selectAll().run()
|
|
|
|
const canSetMarkToBold = editor.can().setMark('bold')
|
|
|
|
expect(canSetMarkToBold).to.eq(true)
|
|
})
|
|
})
|