mirror of
https://github.com/ueberdosis/tiptap.git
synced 2024-12-24 01:17:50 +08:00
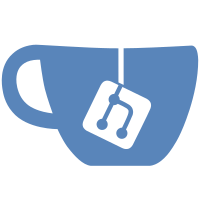
* chore: add precommit hook for eslint fixes, fix linting issues * chore: add eslint import sort plugin
62 lines
1.1 KiB
TypeScript
62 lines
1.1 KiB
TypeScript
import { mergeAttributes, Node } from '@tiptap/core'
|
|
|
|
export interface TaskListOptions {
|
|
itemTypeName: string,
|
|
HTMLAttributes: Record<string, any>,
|
|
}
|
|
|
|
declare module '@tiptap/core' {
|
|
interface Commands<ReturnType> {
|
|
taskList: {
|
|
/**
|
|
* Toggle a task list
|
|
*/
|
|
toggleTaskList: () => ReturnType,
|
|
}
|
|
}
|
|
}
|
|
|
|
export const TaskList = Node.create<TaskListOptions>({
|
|
name: 'taskList',
|
|
|
|
addOptions() {
|
|
return {
|
|
itemTypeName: 'taskItem',
|
|
HTMLAttributes: {},
|
|
}
|
|
},
|
|
|
|
group: 'block list',
|
|
|
|
content() {
|
|
return `${this.options.itemTypeName}+`
|
|
},
|
|
|
|
parseHTML() {
|
|
return [
|
|
{
|
|
tag: `ul[data-type="${this.name}"]`,
|
|
priority: 51,
|
|
},
|
|
]
|
|
},
|
|
|
|
renderHTML({ HTMLAttributes }) {
|
|
return ['ul', mergeAttributes(this.options.HTMLAttributes, HTMLAttributes, { 'data-type': this.name }), 0]
|
|
},
|
|
|
|
addCommands() {
|
|
return {
|
|
toggleTaskList: () => ({ commands }) => {
|
|
return commands.toggleList(this.name, this.options.itemTypeName)
|
|
},
|
|
}
|
|
},
|
|
|
|
addKeyboardShortcuts() {
|
|
return {
|
|
'Mod-Shift-9': () => this.editor.commands.toggleTaskList(),
|
|
}
|
|
},
|
|
})
|